# Face Detection For Python
This package implements parts of Google®'s [**MediaPipe**](https://mediapipe.dev/#!) models in pure Python (with a little help from Numpy and PIL) without `Protobuf` graphs and with minimal dependencies (just [**TF Lite**](https://www.tensorflow.org/lite/api_docs) and [**Pillow**](https://python-pillow.org/)).
## Models and Examples
The package provides the following models:
* Face Detection
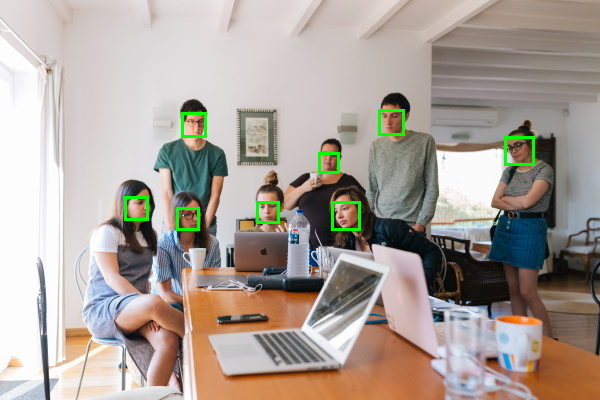
* Face Landmark Detection
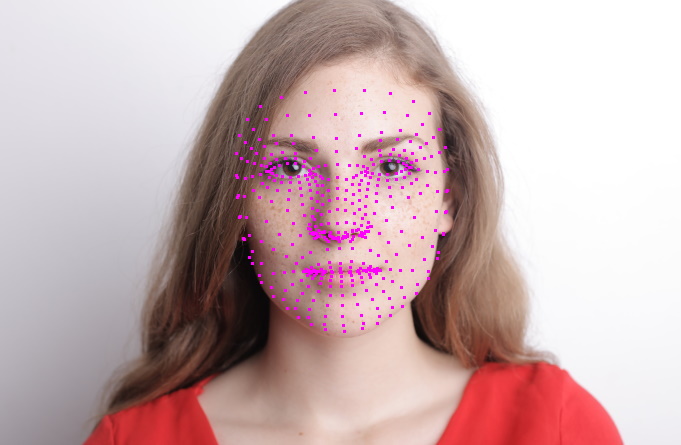
* Iris Landmark Detection
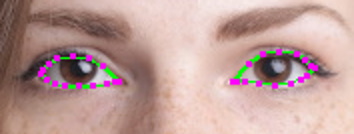
* Iris recoloring example
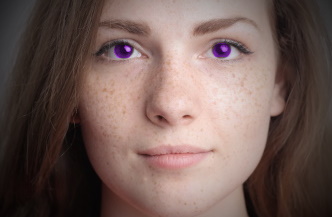
## Motivation
The package doesn't use the graph approach implemented by **MediaPipe** and
is therefore not as flexible. It is, however, somewhat easier to use and
understand and more accessible to recreational programming and experimenting
with the pretrained ML models than the rather complex **MediaPipe** framework.
Here's how face detection works and an image like shown above can be produced:
```python
from fdlite import FaceDetection, FaceDetectionModel
from fdlite.render import Colors, detections_to_render_data, render_to_image
from PIL import Image
image = Image.open('group.jpg')
detect_faces = FaceDetection(model_type=FaceDetectionModel.BACK_CAMERA)
faces = detect_faces(image)
if not len(faces):
print('no faces detected :(')
else:
render_data = detections_to_render_data(faces, bounds_color=Colors.GREEN)
render_to_image(render_data, image).show()
```
While this example isn't that much simpler than the **MediaPipe** equivalent,
some models (e.g. iris detection) aren't available in the Python API.
Note that the package ships with five models:
* `FaceDetectionModel.FRONT_CAMERA` - a smaller model optimised for
selfies and close-up portraits; this is the default model used
* `FaceDetectionModel.BACK_CAMERA` - a larger model suitable for group
images and wider shots with smaller faces
* `FaceDetectionModel.SHORT` - a model best suited for short range images,
i.e. faces are within 2 metres from the camera
* `FaceDetectionModel.FULL` - a model best suited for mid range images,
i.e. faces are within 5 metres from the camera
* `FaceDetectionModel.FULL_SPARSE` - a model best suited for mid range images,
i.e. faces are within 5 metres from the camera
The `FaceDetectionModel.FULL` and `FaceDetectionModel.FULL_SPARSE` models are
equivalent in terms of detection quality. They differ in that the full model
is a dense model whereas the sparse model runs up to 30% faster on CPUs. On a
GPU, both models exhibit similar runtime performance. In addition, the dense
full model has slightly better [Recall](https://en.wikipedia.org/wiki/Precision_and_recall),
whereas the sparse model features a higher [Precision](https://en.wikipedia.org/wiki/Precision_and_recall).
If you don't know whether the image is a close-up portrait or you get no
detections with the default model, try using the `BACK_CAMERA`-model instead.
## Installation
The latest release version is available in [PyPI](https://pypi.org/project/face-detection-tflite/0.1.0/)
and can be installed via:
```sh
pip install -U face-detection-tflite
```
The package can be also installed from source by navigating to the folder
containing `setup.py` and running
```sh
pip install .
```
from a shell or command prompt.
Raw data
{
"_id": null,
"home_page": "https://github.com/patlevin/face-detection-tflite",
"name": "face-detection-tflite",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "AI, face-detection, tensorflow, tflite, face-landmarks, iris-detection, face-mesh",
"author": "Patrick Levin",
"author_email": "vertical-pink@protonmail.com",
"download_url": "https://files.pythonhosted.org/packages/90/b7/ea63b3707e23591c95ff050835acf34538ea888a3d85d04466828e8654ad/face-detection-tflite-0.6.0.tar.gz",
"platform": null,
"description": "# Face Detection For Python\n\nThis package implements parts of Google\u00ae's [**MediaPipe**](https://mediapipe.dev/#!) models in pure Python (with a little help from Numpy and PIL) without `Protobuf` graphs and with minimal dependencies (just [**TF Lite**](https://www.tensorflow.org/lite/api_docs) and [**Pillow**](https://python-pillow.org/)).\n\n## Models and Examples\n\nThe package provides the following models:\n\n* Face Detection\n\n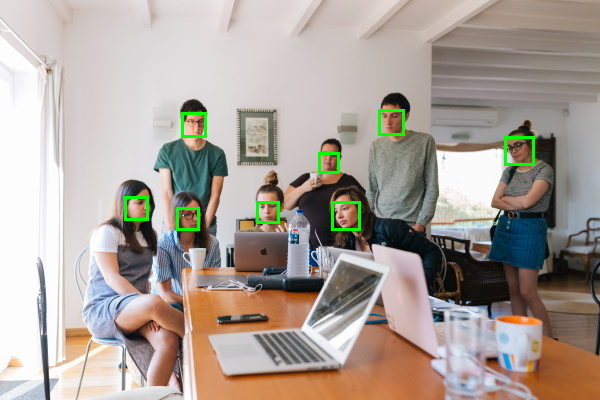\n\n* Face Landmark Detection\n\n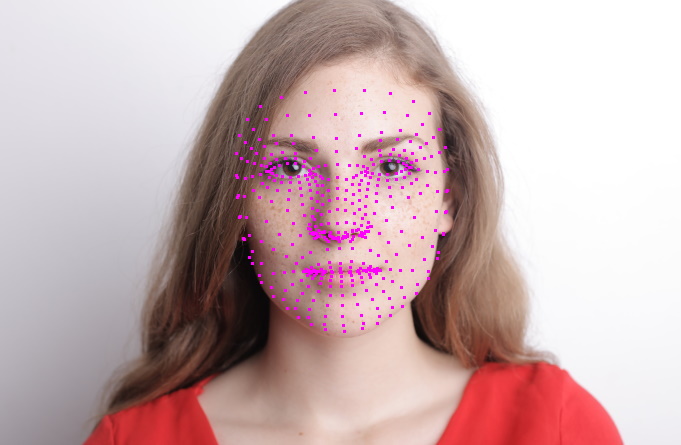\n\n* Iris Landmark Detection\n\n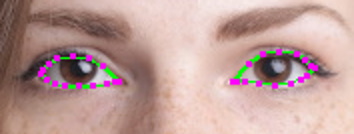\n\n* Iris recoloring example\n\n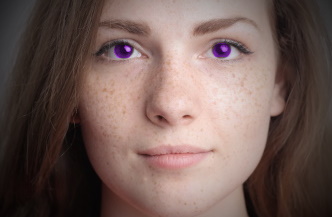\n\n## Motivation\n\nThe package doesn't use the graph approach implemented by **MediaPipe** and\nis therefore not as flexible. It is, however, somewhat easier to use and\nunderstand and more accessible to recreational programming and experimenting\nwith the pretrained ML models than the rather complex **MediaPipe** framework.\n\nHere's how face detection works and an image like shown above can be produced:\n\n```python\nfrom fdlite import FaceDetection, FaceDetectionModel\nfrom fdlite.render import Colors, detections_to_render_data, render_to_image \nfrom PIL import Image\n\nimage = Image.open('group.jpg')\ndetect_faces = FaceDetection(model_type=FaceDetectionModel.BACK_CAMERA)\nfaces = detect_faces(image)\nif not len(faces):\n print('no faces detected :(')\nelse:\n render_data = detections_to_render_data(faces, bounds_color=Colors.GREEN)\n render_to_image(render_data, image).show()\n```\n\nWhile this example isn't that much simpler than the **MediaPipe** equivalent,\nsome models (e.g. iris detection) aren't available in the Python API.\n\nNote that the package ships with five models:\n\n* `FaceDetectionModel.FRONT_CAMERA` - a smaller model optimised for\n selfies and close-up portraits; this is the default model used\n* `FaceDetectionModel.BACK_CAMERA` - a larger model suitable for group\n images and wider shots with smaller faces\n* `FaceDetectionModel.SHORT` - a model best suited for short range images,\n i.e. faces are within 2 metres from the camera\n* `FaceDetectionModel.FULL` - a model best suited for mid range images,\n i.e. faces are within 5 metres from the camera\n* `FaceDetectionModel.FULL_SPARSE` - a model best suited for mid range images,\n i.e. faces are within 5 metres from the camera\n\nThe `FaceDetectionModel.FULL` and `FaceDetectionModel.FULL_SPARSE` models are\nequivalent in terms of detection quality. They differ in that the full model\nis a dense model whereas the sparse model runs up to 30% faster on CPUs. On a\nGPU, both models exhibit similar runtime performance. In addition, the dense\nfull model has slightly better [Recall](https://en.wikipedia.org/wiki/Precision_and_recall),\nwhereas the sparse model features a higher [Precision](https://en.wikipedia.org/wiki/Precision_and_recall).\n\nIf you don't know whether the image is a close-up portrait or you get no\ndetections with the default model, try using the `BACK_CAMERA`-model instead.\n\n## Installation\n\nThe latest release version is available in [PyPI](https://pypi.org/project/face-detection-tflite/0.1.0/)\nand can be installed via:\n\n```sh\npip install -U face-detection-tflite\n```\n\nThe package can be also installed from source by navigating to the folder\ncontaining `setup.py` and running\n\n```sh\npip install .\n```\n\nfrom a shell or command prompt.\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A Python port of Google MediaPipe Face Detection modules",
"version": "0.6.0",
"project_urls": {
"Bug Tracker": "https://github.com/patlevin/face-detection-tflite/issues",
"Homepage": "https://github.com/patlevin/face-detection-tflite"
},
"split_keywords": [
"ai",
" face-detection",
" tensorflow",
" tflite",
" face-landmarks",
" iris-detection",
" face-mesh"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "4860108c6c287763d6067c60d196f7dd9bf397dcb8a3dd425a004ca858b08966",
"md5": "bc133c0bb5a33f0dd4137a9aa406692f",
"sha256": "1eeb3d6a51dffc43116af273f3209c6aa61d873986ba22f81f76b9ae7a4fe1ce"
},
"downloads": -1,
"filename": "face_detection_tflite-0.6.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "bc133c0bb5a33f0dd4137a9aa406692f",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 6851288,
"upload_time": "2024-05-13T21:28:44",
"upload_time_iso_8601": "2024-05-13T21:28:44.489228Z",
"url": "https://files.pythonhosted.org/packages/48/60/108c6c287763d6067c60d196f7dd9bf397dcb8a3dd425a004ca858b08966/face_detection_tflite-0.6.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "90b7ea63b3707e23591c95ff050835acf34538ea888a3d85d04466828e8654ad",
"md5": "8ae69eafe82766732b8a55ab38718a0c",
"sha256": "7cb9ce9994b8353fffc963e12a155af6c6c6187ae5b7312fddf33fe7c6e48f11"
},
"downloads": -1,
"filename": "face-detection-tflite-0.6.0.tar.gz",
"has_sig": false,
"md5_digest": "8ae69eafe82766732b8a55ab38718a0c",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 7344756,
"upload_time": "2024-05-13T21:28:57",
"upload_time_iso_8601": "2024-05-13T21:28:57.283419Z",
"url": "https://files.pythonhosted.org/packages/90/b7/ea63b3707e23591c95ff050835acf34538ea888a3d85d04466828e8654ad/face-detection-tflite-0.6.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-05-13 21:28:57",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "patlevin",
"github_project": "face-detection-tflite",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "face-detection-tflite"
}