# jquants-algo
[](https://pypi.org/project/jquants-algo/)
[](https://opensource.org/licenses/MIT)
[](https://codecov.io/gh/10mohi6/jquants-algo-python)
[](https://github.com/10mohi6/jquants-algo-python/actions/workflows/python-package.yml)
[](https://pypi.org/project/jquants-algo/)
[](https://pepy.tech/project/jquants-algo)
jquants-algo is a python library for algorithmic trading with japanese stock trade using J-Quants on Python 3.8 and above.
## Installation
$ pip install jquants-algo
## Usage
### backtest
```python
from jquants_algo import Algo
import pprint
class MyAlgo(Algo):
def strategy(self):
fast_ma = self.sma(period=3)
slow_ma = self.sma(period=5)
# golden cross
self.sell_exit = self.buy_entry = (fast_ma > slow_ma) & (
fast_ma.shift() <= slow_ma.shift()
)
# dead cross
self.buy_exit = self.sell_entry = (fast_ma < slow_ma) & (
fast_ma.shift() >= slow_ma.shift()
)
algo = MyAlgo(
mail_address="<your J-Quants mail address>",
password="<your J-Quants password>",
ticker="7203", # TOYOTA
size=100, # 100 shares
)
pprint.pprint(algo.backtest())
```
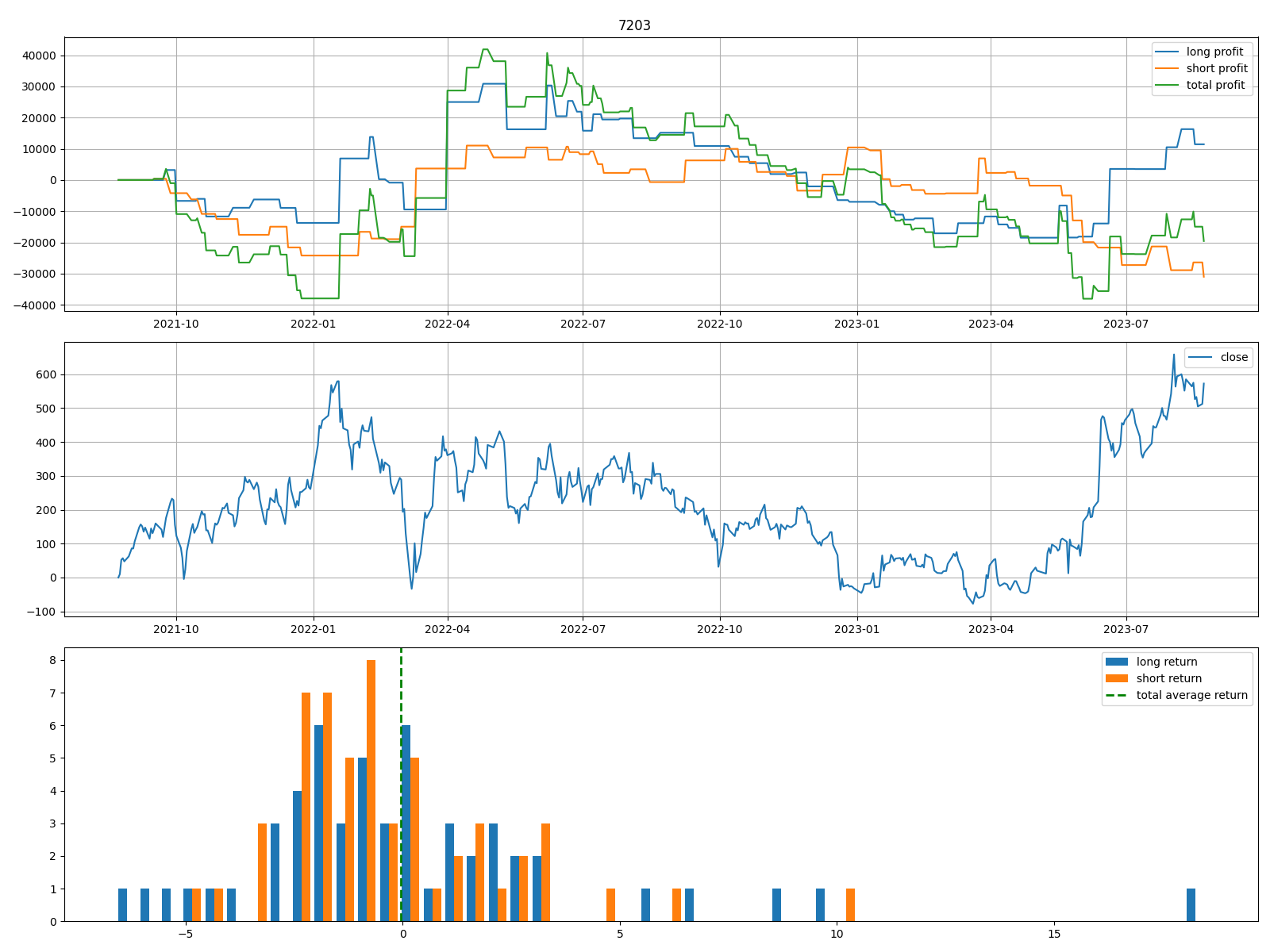
```python
{'long': {'average return': '0.156',
'maximum drawdown': '49350.000',
'profit': '11450.000',
'profit factor': '1.080',
'riskreward ratio': '1.455',
'sharpe ratio': '0.038',
'trades': '54.000',
'win rate': '0.426'},
'short': {'average return': '-0.238',
'maximum drawdown': '42050.000',
'profit': '-31020.000',
'profit factor': '0.754',
'riskreward ratio': '1.319',
'sharpe ratio': '-0.091',
'trades': '55.000',
'win rate': '0.364'},
'total': {'average return': '-0.043',
'maximum drawdown': '79950.000',
'profit': '-19570.000',
'profit factor': '0.927',
'riskreward ratio': '1.423',
'sharpe ratio': '-0.013',
'trades': '109.000',
'win rate': '0.394'}}
```
### predict
```python
from jquants_algo import Algo
import pprint
class MyAlgo(Algo):
def strategy(self):
fast_ma = self.sma(period=3)
slow_ma = self.sma(period=5)
# golden cross
self.sell_exit = self.buy_entry = (fast_ma > slow_ma) & (
fast_ma.shift() <= slow_ma.shift()
)
# dead cross
self.buy_exit = self.sell_entry = (fast_ma < slow_ma) & (
fast_ma.shift() >= slow_ma.shift()
)
algo = MyAlgo(
mail_address="<your J-Quants mail address>",
password="<your J-Quants password>",
ticker="7203", # TOYOTA
size=100, # 100 shares
)
pprint.pprint(algo.predict())
```
```python
{'buy entry': True,
'buy exit': False,
'close': 2416.5,
'date': '2023-08-22',
'sell entry': False,
'sell exit': True}
```
### advanced
```python
from jquants_algo import Algo
import pprint
class MyAlgo(Algo):
def strategy(self):
rsi = self.rsi(period=10)
ema = self.ema(period=20)
lower = ema - (ema * 0.001)
upper = ema + (ema * 0.001)
self.buy_entry = (rsi < 30) & (self.df.Close < lower)
self.sell_entry = (rsi > 70) & (self.df.Close > upper)
self.sell_exit = ema > self.df.Close
self.buy_exit = ema < self.df.Close
algo = MyAlgo(
mail_address="<your J-Quants mail address>",
password="<your J-Quants password>",
ticker="7203", # TOYOTA
size=100, # 100 shares
outputs_dir_path="outputs",
data_dir_path="data",
)
pprint.pprint(algo.backtest())
pprint.pprint(algo.predict())
```
## Supported indicators
- Simple Moving Average 'sma'
- Exponential Moving Average 'ema'
- Moving Average Convergence Divergence 'macd'
- Relative Strenght Index 'rsi'
- Bollinger Bands 'bbands'
- Market Momentum 'mom'
- Stochastic Oscillator 'stoch'
- Average True Range 'atr'
## Getting started
For help getting started with J-Quants, view our online [documentation](https://jpx-jquants.com/).
Raw data
{
"_id": null,
"home_page": "",
"name": "jquants-algo",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "",
"keywords": "algorithmic trading,python,japanese stock,trade,J-Quants,jquants",
"author": "",
"author_email": "10mohi6 <10.mohi.6.y@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/8a/60/c09d5145ed65765b529ca3d6bcf60941cc73e0cad05ba09fd02aa4c347f4/jquants-algo-0.1.1.tar.gz",
"platform": null,
"description": "# jquants-algo\n\n[](https://pypi.org/project/jquants-algo/)\n[](https://opensource.org/licenses/MIT)\n[](https://codecov.io/gh/10mohi6/jquants-algo-python)\n[](https://github.com/10mohi6/jquants-algo-python/actions/workflows/python-package.yml)\n[](https://pypi.org/project/jquants-algo/)\n[](https://pepy.tech/project/jquants-algo)\n\njquants-algo is a python library for algorithmic trading with japanese stock trade using J-Quants on Python 3.8 and above.\n\n## Installation\n\n $ pip install jquants-algo\n\n## Usage\n\n### backtest\n\n```python\nfrom jquants_algo import Algo\nimport pprint\n\nclass MyAlgo(Algo):\n def strategy(self):\n fast_ma = self.sma(period=3)\n slow_ma = self.sma(period=5)\n # golden cross\n self.sell_exit = self.buy_entry = (fast_ma > slow_ma) & (\n fast_ma.shift() <= slow_ma.shift()\n )\n # dead cross\n self.buy_exit = self.sell_entry = (fast_ma < slow_ma) & (\n fast_ma.shift() >= slow_ma.shift()\n )\n\nalgo = MyAlgo(\n mail_address=\"<your J-Quants mail address>\",\n password=\"<your J-Quants password>\",\n ticker=\"7203\", # TOYOTA\n size=100, # 100 shares\n)\npprint.pprint(algo.backtest())\n```\n\n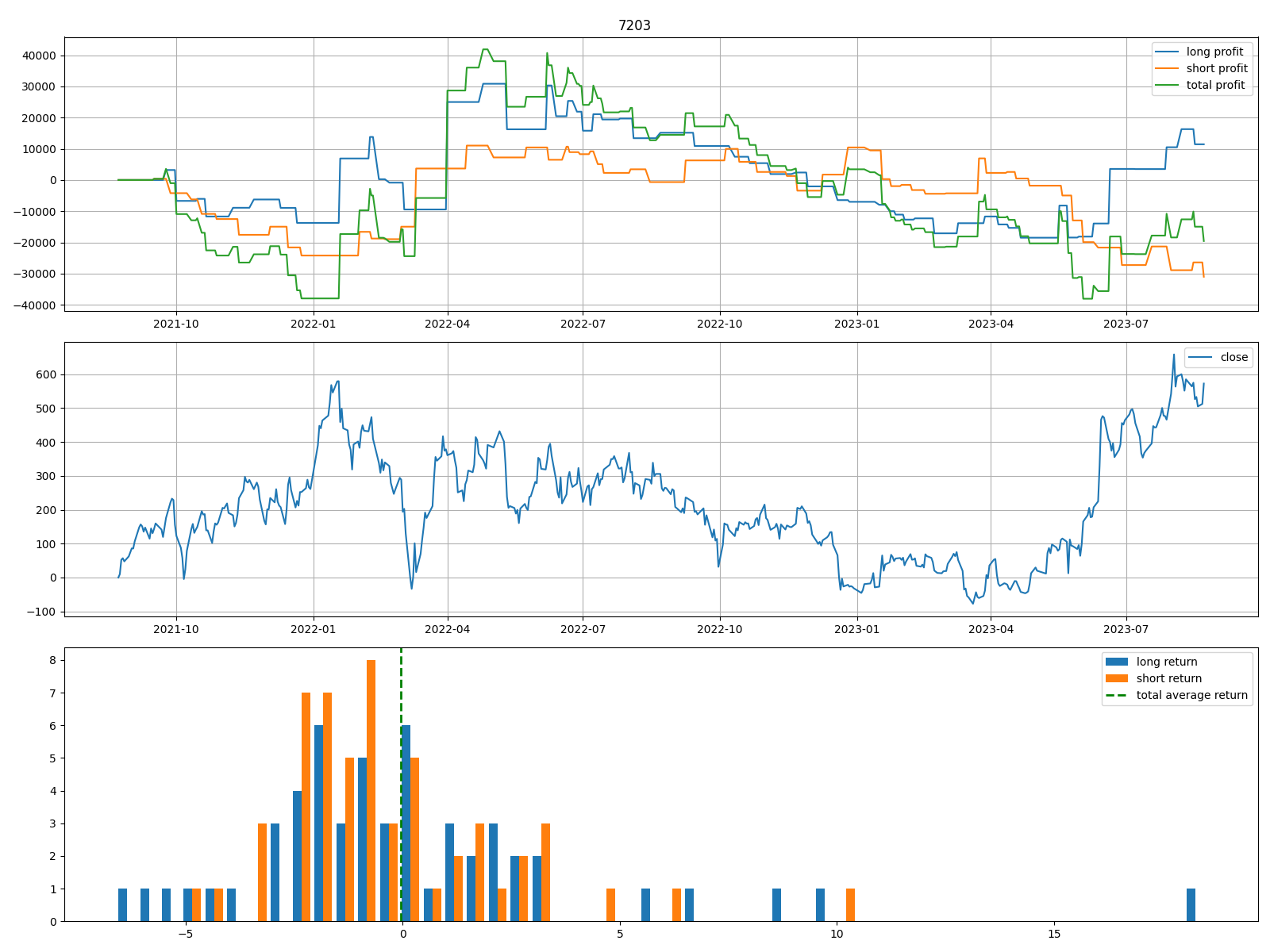\n\n```python\n{'long': {'average return': '0.156',\n 'maximum drawdown': '49350.000',\n 'profit': '11450.000',\n 'profit factor': '1.080',\n 'riskreward ratio': '1.455',\n 'sharpe ratio': '0.038',\n 'trades': '54.000',\n 'win rate': '0.426'},\n 'short': {'average return': '-0.238',\n 'maximum drawdown': '42050.000',\n 'profit': '-31020.000',\n 'profit factor': '0.754',\n 'riskreward ratio': '1.319',\n 'sharpe ratio': '-0.091',\n 'trades': '55.000',\n 'win rate': '0.364'},\n 'total': {'average return': '-0.043',\n 'maximum drawdown': '79950.000',\n 'profit': '-19570.000',\n 'profit factor': '0.927',\n 'riskreward ratio': '1.423',\n 'sharpe ratio': '-0.013',\n 'trades': '109.000',\n 'win rate': '0.394'}}\n```\n\n### predict\n\n```python\nfrom jquants_algo import Algo\nimport pprint\n\nclass MyAlgo(Algo):\n def strategy(self):\n fast_ma = self.sma(period=3)\n slow_ma = self.sma(period=5)\n # golden cross\n self.sell_exit = self.buy_entry = (fast_ma > slow_ma) & (\n fast_ma.shift() <= slow_ma.shift()\n )\n # dead cross\n self.buy_exit = self.sell_entry = (fast_ma < slow_ma) & (\n fast_ma.shift() >= slow_ma.shift()\n )\n\nalgo = MyAlgo(\n mail_address=\"<your J-Quants mail address>\",\n password=\"<your J-Quants password>\",\n ticker=\"7203\", # TOYOTA\n size=100, # 100 shares\n)\npprint.pprint(algo.predict())\n```\n\n```python\n{'buy entry': True,\n 'buy exit': False,\n 'close': 2416.5,\n 'date': '2023-08-22',\n 'sell entry': False,\n 'sell exit': True}\n```\n\n### advanced\n\n```python\nfrom jquants_algo import Algo\nimport pprint\n\nclass MyAlgo(Algo):\n def strategy(self):\n rsi = self.rsi(period=10)\n ema = self.ema(period=20)\n lower = ema - (ema * 0.001)\n upper = ema + (ema * 0.001)\n self.buy_entry = (rsi < 30) & (self.df.Close < lower)\n self.sell_entry = (rsi > 70) & (self.df.Close > upper)\n self.sell_exit = ema > self.df.Close\n self.buy_exit = ema < self.df.Close\n\nalgo = MyAlgo(\n mail_address=\"<your J-Quants mail address>\",\n password=\"<your J-Quants password>\",\n ticker=\"7203\", # TOYOTA\n size=100, # 100 shares\n outputs_dir_path=\"outputs\",\n data_dir_path=\"data\",\n)\npprint.pprint(algo.backtest())\npprint.pprint(algo.predict())\n```\n\n## Supported indicators\n\n- Simple Moving Average 'sma'\n- Exponential Moving Average 'ema'\n- Moving Average Convergence Divergence 'macd'\n- Relative Strenght Index 'rsi'\n- Bollinger Bands 'bbands'\n- Market Momentum 'mom'\n- Stochastic Oscillator 'stoch'\n- Average True Range 'atr'\n\n## Getting started\n\nFor help getting started with J-Quants, view our online [documentation](https://jpx-jquants.com/).\n",
"bugtrack_url": null,
"license": "",
"summary": "jquants-algo is a python library for algorithmic trading with japanese stock trade using J-Quants on Python 3.8 and above.",
"version": "0.1.1",
"project_urls": {
"Documentation": "https://github.com/10mohi6/jquants-algo-python",
"Homepage": "https://github.com/10mohi6/jquants-algo-python",
"Repository": "https://github.com/10mohi6/jquants-algo-python.git"
},
"split_keywords": [
"algorithmic trading",
"python",
"japanese stock",
"trade",
"j-quants",
"jquants"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "8572fd25c87e1500b3abf14221c14f987289b28e9372b596e0b65d318a991a16",
"md5": "100bf231b88c4b3274684fd53b2b717a",
"sha256": "7d0898dbc2f785454ef4c2c920a96ead2f0b9a6678bb7c1a0a001101395e7ef8"
},
"downloads": -1,
"filename": "jquants_algo-0.1.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "100bf231b88c4b3274684fd53b2b717a",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 6770,
"upload_time": "2023-11-13T15:21:18",
"upload_time_iso_8601": "2023-11-13T15:21:18.026390Z",
"url": "https://files.pythonhosted.org/packages/85/72/fd25c87e1500b3abf14221c14f987289b28e9372b596e0b65d318a991a16/jquants_algo-0.1.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8a60c09d5145ed65765b529ca3d6bcf60941cc73e0cad05ba09fd02aa4c347f4",
"md5": "24d9a6dafcad509f8e93b4e7ea8420ae",
"sha256": "d29a6ac0af0c9ec2b53a44c4059f60772575c488fbf7763f5a67bf66f47aa376"
},
"downloads": -1,
"filename": "jquants-algo-0.1.1.tar.gz",
"has_sig": false,
"md5_digest": "24d9a6dafcad509f8e93b4e7ea8420ae",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 7344,
"upload_time": "2023-11-13T15:21:20",
"upload_time_iso_8601": "2023-11-13T15:21:20.559353Z",
"url": "https://files.pythonhosted.org/packages/8a/60/c09d5145ed65765b529ca3d6bcf60941cc73e0cad05ba09fd02aa4c347f4/jquants-algo-0.1.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-11-13 15:21:20",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "10mohi6",
"github_project": "jquants-algo-python",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "certifi",
"specs": [
[
"==",
"2023.7.22"
]
]
},
{
"name": "charset-normalizer",
"specs": [
[
"==",
"3.3.2"
]
]
},
{
"name": "contourpy",
"specs": [
[
"==",
"1.1.1"
]
]
},
{
"name": "coverage",
"specs": [
[
"==",
"7.3.2"
]
]
},
{
"name": "cycler",
"specs": [
[
"==",
"0.12.1"
]
]
},
{
"name": "exceptiongroup",
"specs": [
[
"==",
"1.1.3"
]
]
},
{
"name": "fonttools",
"specs": [
[
"==",
"4.44.0"
]
]
},
{
"name": "idna",
"specs": [
[
"==",
"3.4"
]
]
},
{
"name": "importlib-resources",
"specs": [
[
"==",
"6.1.1"
]
]
},
{
"name": "iniconfig",
"specs": [
[
"==",
"2.0.0"
]
]
},
{
"name": "joblib",
"specs": [
[
"==",
"1.3.2"
]
]
},
{
"name": "jquants-api-client",
"specs": [
[
"==",
"1.5.0"
]
]
},
{
"name": "kiwisolver",
"specs": [
[
"==",
"1.4.5"
]
]
},
{
"name": "matplotlib",
"specs": [
[
"==",
"3.7.3"
]
]
},
{
"name": "numpy",
"specs": [
[
"==",
"1.24.4"
]
]
},
{
"name": "packaging",
"specs": [
[
"==",
"23.2"
]
]
},
{
"name": "pandas",
"specs": [
[
"==",
"1.5.3"
]
]
},
{
"name": "Pillow",
"specs": [
[
"==",
"10.1.0"
]
]
},
{
"name": "pluggy",
"specs": [
[
"==",
"1.3.0"
]
]
},
{
"name": "pyparsing",
"specs": [
[
"==",
"3.1.1"
]
]
},
{
"name": "pytest",
"specs": [
[
"==",
"7.4.3"
]
]
},
{
"name": "pytest-cov",
"specs": [
[
"==",
"4.1.0"
]
]
},
{
"name": "pytest-mock",
"specs": [
[
"==",
"3.12.0"
]
]
},
{
"name": "python-dateutil",
"specs": [
[
"==",
"2.8.2"
]
]
},
{
"name": "pytz",
"specs": [
[
"==",
"2023.3.post1"
]
]
},
{
"name": "requests",
"specs": [
[
"==",
"2.31.0"
]
]
},
{
"name": "scipy",
"specs": [
[
"==",
"1.10.1"
]
]
},
{
"name": "six",
"specs": [
[
"==",
"1.16.0"
]
]
},
{
"name": "tenacity",
"specs": [
[
"==",
"8.2.3"
]
]
},
{
"name": "threadpoolctl",
"specs": [
[
"==",
"3.2.0"
]
]
},
{
"name": "tomli",
"specs": [
[
"==",
"2.0.1"
]
]
},
{
"name": "types-python-dateutil",
"specs": [
[
"==",
"2.8.19.14"
]
]
},
{
"name": "types-requests",
"specs": [
[
"==",
"2.31.0.6"
]
]
},
{
"name": "types-urllib3",
"specs": [
[
"==",
"1.26.25.14"
]
]
},
{
"name": "urllib3",
"specs": [
[
"==",
"1.26.18"
]
]
},
{
"name": "zipp",
"specs": [
[
"==",
"3.17.0"
]
]
}
],
"lcname": "jquants-algo"
}