Name | leonardo-api JSON |
Version |
0.0.10
JSON |
| download |
home_page | |
Summary | Leonardo.ai Python API |
upload_time | 2023-11-24 16:00:40 |
maintainer | |
docs_url | None |
author | |
requires_python | >=3.8 |
license | MIT License Copyright (c) 2023 Ilya Vereshchagin Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
ai
api
artificial intelligence
image generation
leonardo
leonardo.ai
llm
stablediffusion
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
## This is Leonardo.ai API.
[](https://badge.fury.io/py/leonardo-api) [](https://github.com/wwakabobik/leonardo_api/actions/workflows/master-linters.yml)
This package contains Python API for [Leonardo.ai](https://leonardo.ai/) based on official [API documentation](https://docs.leonardo.ai/reference).
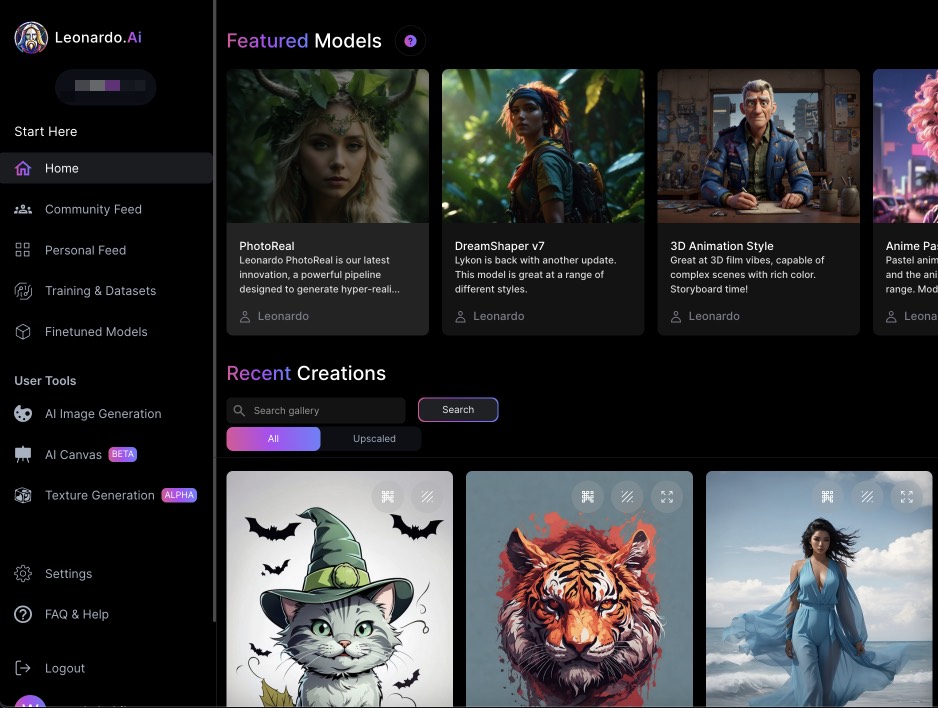
To install the package, please use package from [pypi](https://pypi.org/project/leonardo-api/):
```bash
pip install leonardo-api
```
This Python API provides access to Leonardo API using synchronous methods (based on requests library) as well as asynchronous (aiohttp). You can choose one of them - `Leonardo` or `LeonardoAsync`.
To start, you must have paid subscription and create an API access token from you [settings page](https://app.leonardo.ai/settings)->User API. Then, init manager class with using your access_token:
```python
from leonardo_api import Leonardo
leonardo = Leonardo(auth_token='abcd-1234-5678-90ef-deadbeef00000')
```
Now you can use all API methods, provided by Leonardo.ai API, i.e. starting getting user info and generating your first image:
```python
response = leonardo.get_user_info() # get your user info
response = leonardo.post_generations(prompt="The quick brown fox jumps over the lazy dog", num_images=1,
negative_prompt='schrodinger cat paradox',
model_id='e316348f-7773-490e-adcd-46757c738eb7', width=1024, height=768,
guidance_scale=7)
```
In according to API reference, you will get the json answer with content about pending job like following:
```json
{"sdGenerationJob": {"generationId": "123456-0987-aaaa-bbbb-01010101010"}}
```
To obtain your image you need to use additional method:
```python
response = leonardo.get_single_generation(generation_id) # get it using response['sdGenerationJob']['generationId']
```
Or, optionally, you may wait for job completion using following method:
```python
response = leonardo.wait_for_image_generation(generation_id=response['sdGenerationJob']['generationId'])
```
Finally, you'll get your array of images:
```python
[{'url': 'https://cdn.leonardo.ai/users/abcd-1234-5678-90ef-deadbeef00000/generations/123456-0987-aaaa-bbbb-01010101010/Absolute_Reality_v16_The_quick_brown_fox_jumps_0.jpg', 'nsfw': False, 'id': 'aaaaaa-bbbb-cccc-dddd-ffffffffff', 'likeCount': 0, 'generated_image_variation_generics': []}]
```
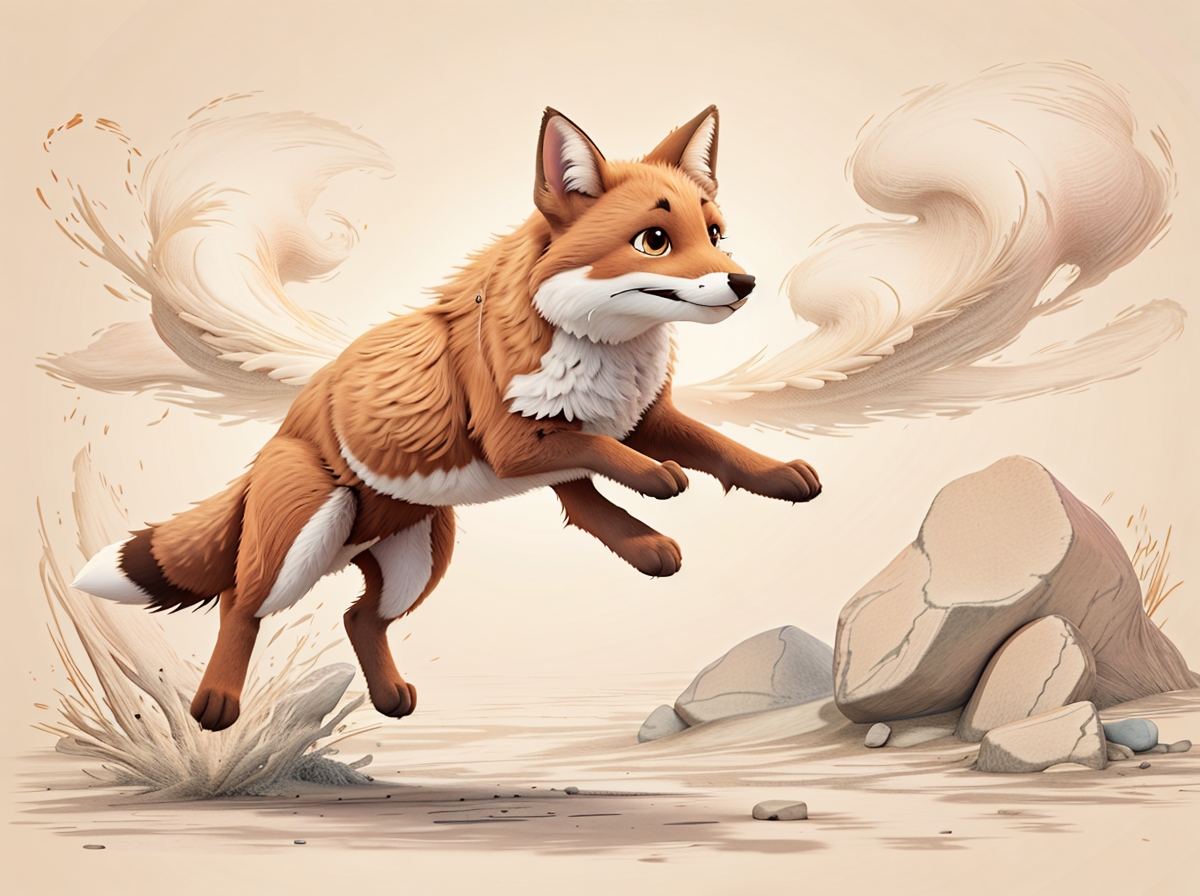
You'll find descriptions for rest of the methods in official [API reference](https://docs.leonardo.ai/reference).
---
As option, you may want to use preloaded dicts with models (nsfw/community/platform):
```python
from leonardo_api import platform_models, custom_models, nsfw_models
```
Which contains details of models like following:
```python
{
"data": {
"custom_models": [
{
"id": "f1929ea3-b169-4c18-a16c-5d58b4292c69",
"name": "RPG v5",
# rest of the model data
}
# rest models
]
}
}
```
Have fun and enjoy!
## Donations
If you like this project, you can support it by donating via [DonationAlerts](https://www.donationalerts.com/r/rocketsciencegeek).
Raw data
{
"_id": null,
"home_page": "",
"name": "leonardo-api",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "Iliya Vereshchagin <i.vereshchagin@gmail.com>",
"keywords": "ai,api,artificial intelligence,image generation,leonardo,leonardo.ai,llm,stablediffusion",
"author": "",
"author_email": "Iliya Vereshchagin <i.vereshchagin@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/6f/5f/2544352931d94a6cd55640cfac4e387005e2976a049d988ef1696198e68b/leonardo_api-0.0.10.tar.gz",
"platform": null,
"description": "## This is Leonardo.ai API.\n\n[](https://badge.fury.io/py/leonardo-api) [](https://github.com/wwakabobik/leonardo_api/actions/workflows/master-linters.yml)\n\nThis package contains Python API for [Leonardo.ai](https://leonardo.ai/) based on official [API documentation](https://docs.leonardo.ai/reference).\n\n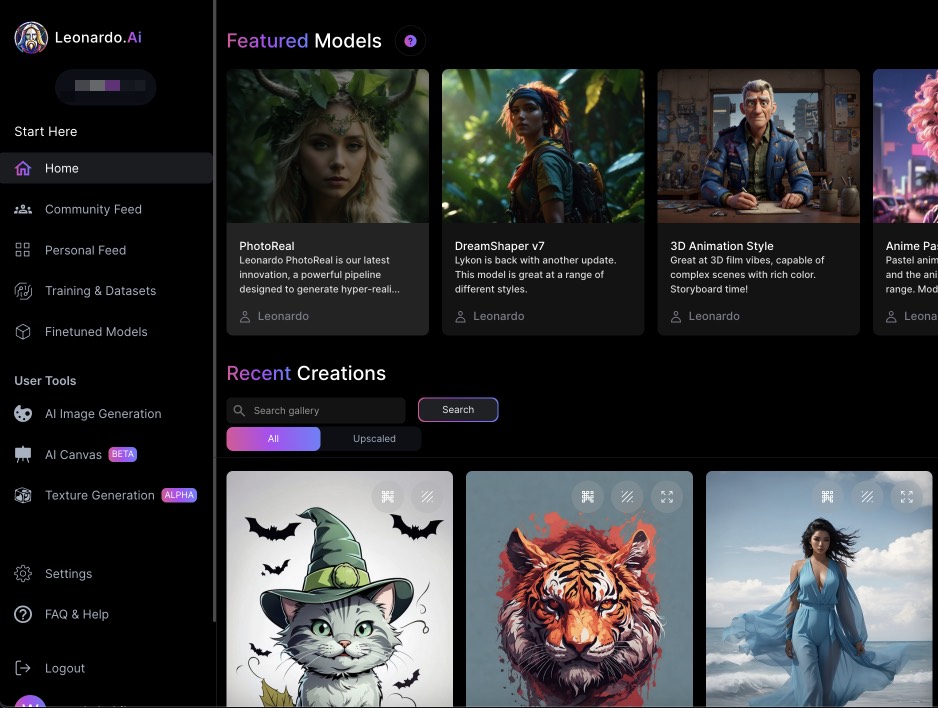\n\nTo install the package, please use package from [pypi](https://pypi.org/project/leonardo-api/):\n\n```bash\npip install leonardo-api\n```\n\nThis Python API provides access to Leonardo API using synchronous methods (based on requests library) as well as asynchronous (aiohttp). You can choose one of them - `Leonardo` or `LeonardoAsync`.\n\nTo start, you must have paid subscription and create an API access token from you [settings page](https://app.leonardo.ai/settings)->User API. Then, init manager class with using your access_token:\n\n```python\nfrom leonardo_api import Leonardo\n\nleonardo = Leonardo(auth_token='abcd-1234-5678-90ef-deadbeef00000')\n```\n\nNow you can use all API methods, provided by Leonardo.ai API, i.e. starting getting user info and generating your first image:\n\n```python\nresponse = leonardo.get_user_info() # get your user info\nresponse = leonardo.post_generations(prompt=\"The quick brown fox jumps over the lazy dog\", num_images=1,\n negative_prompt='schrodinger cat paradox',\n model_id='e316348f-7773-490e-adcd-46757c738eb7', width=1024, height=768,\n guidance_scale=7)\n```\n\nIn according to API reference, you will get the json answer with content about pending job like following:\n\n```json\n{\"sdGenerationJob\": {\"generationId\": \"123456-0987-aaaa-bbbb-01010101010\"}}\n```\n\nTo obtain your image you need to use additional method:\n\n```python\nresponse = leonardo.get_single_generation(generation_id) # get it using response['sdGenerationJob']['generationId']\n```\n\nOr, optionally, you may wait for job completion using following method:\n\n```python\nresponse = leonardo.wait_for_image_generation(generation_id=response['sdGenerationJob']['generationId'])\n```\n\nFinally, you'll get your array of images:\n\n```python\n[{'url': 'https://cdn.leonardo.ai/users/abcd-1234-5678-90ef-deadbeef00000/generations/123456-0987-aaaa-bbbb-01010101010/Absolute_Reality_v16_The_quick_brown_fox_jumps_0.jpg', 'nsfw': False, 'id': 'aaaaaa-bbbb-cccc-dddd-ffffffffff', 'likeCount': 0, 'generated_image_variation_generics': []}]\n```\n\n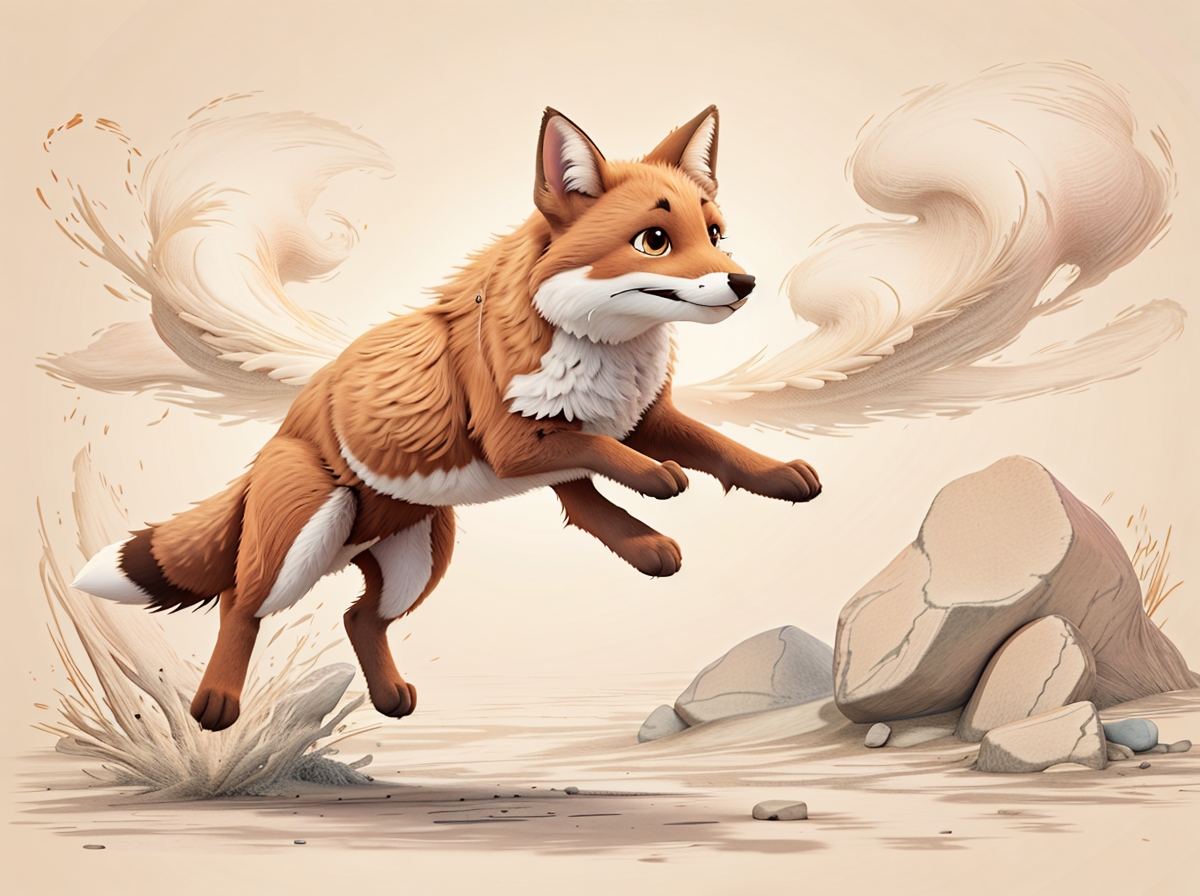\n\nYou'll find descriptions for rest of the methods in official [API reference](https://docs.leonardo.ai/reference).\n\n---\n\nAs option, you may want to use preloaded dicts with models (nsfw/community/platform):\n\n```python\nfrom leonardo_api import platform_models, custom_models, nsfw_models\n```\n\nWhich contains details of models like following:\n\n```python\n{\n \"data\": {\n \"custom_models\": [\n {\n \"id\": \"f1929ea3-b169-4c18-a16c-5d58b4292c69\",\n \"name\": \"RPG v5\",\n # rest of the model data\n }\n # rest models\n ]\n }\n}\n```\n\nHave fun and enjoy!\n\n## Donations\nIf you like this project, you can support it by donating via [DonationAlerts](https://www.donationalerts.com/r/rocketsciencegeek).\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2023 Ilya Vereshchagin Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.",
"summary": "Leonardo.ai Python API",
"version": "0.0.10",
"project_urls": {
"Bug Tracker": "https://github.com/wwakabobik/leonardo_api/issues",
"Homepage": "https://github.com/wwakabobik/leonardo_api"
},
"split_keywords": [
"ai",
"api",
"artificial intelligence",
"image generation",
"leonardo",
"leonardo.ai",
"llm",
"stablediffusion"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "1c457f7331db88c5cb5cfce19335b8c5f7a8f33593ece8b832b26ea5b854edfd",
"md5": "d9cbfa1ed326245b8a458a0dacb7e0fb",
"sha256": "77034653bbd6a0978124710ea6801662746e572a6bddda3194f07f2a135e65d4"
},
"downloads": -1,
"filename": "leonardo_api-0.0.10-py3-none-any.whl",
"has_sig": false,
"md5_digest": "d9cbfa1ed326245b8a458a0dacb7e0fb",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 48363,
"upload_time": "2023-11-24T16:00:34",
"upload_time_iso_8601": "2023-11-24T16:00:34.293164Z",
"url": "https://files.pythonhosted.org/packages/1c/45/7f7331db88c5cb5cfce19335b8c5f7a8f33593ece8b832b26ea5b854edfd/leonardo_api-0.0.10-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6f5f2544352931d94a6cd55640cfac4e387005e2976a049d988ef1696198e68b",
"md5": "9a2ffd0a664e47af8d6fd415d0d9263e",
"sha256": "d0e9dd8a61e116377a5b0a33798009bacce1a0f96b13fb05e55000e7367a556c"
},
"downloads": -1,
"filename": "leonardo_api-0.0.10.tar.gz",
"has_sig": false,
"md5_digest": "9a2ffd0a664e47af8d6fd415d0d9263e",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 814307,
"upload_time": "2023-11-24T16:00:40",
"upload_time_iso_8601": "2023-11-24T16:00:40.171056Z",
"url": "https://files.pythonhosted.org/packages/6f/5f/2544352931d94a6cd55640cfac4e387005e2976a049d988ef1696198e68b/leonardo_api-0.0.10.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-11-24 16:00:40",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "wwakabobik",
"github_project": "leonardo_api",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "leonardo-api"
}