# Live pyqtgraph plot
Pglive package adds support for thread-safe live plotting based on pyqtgraph.
It supports PyQt5, PyQt6 and PySide6.
# Description #
Pyqtgraph doesn't offer easy way to implement live plotting out of the box.
The aim of PgLive module is to provide easy way of thread-safe live plotting.
To do this, PgLive provides DataConnector object, which consumes data
and manages data plotting. DataConnector interface provides Pause and Resume method, update rate and maximum number of
plotted points. All that needs to be done is to connect plots and data sources with DataConnector.
Once data is collected, DataConnector is sending signals to the GUI main loop.
**Focus on data handling - leave plotting to pglive.**
You can find many examples for PyQt5, PyQt6 or PySide6.
# Code example #
```python
import sys
from math import sin
from threading import Thread
from time import sleep
from PyQt6.QtWidgets import QApplication
from pglive.sources.data_connector import DataConnector
from pglive.sources.live_plot import LiveLinePlot
from pglive.sources.live_plot_widget import LivePlotWidget
"""
Line plot is displayed in this example.
"""
app = QApplication(sys.argv)
running = True
plot_widget = LivePlotWidget(title="Line Plot @ 100Hz")
plot_curve = LiveLinePlot()
plot_widget.addItem(plot_curve)
# DataConnector holding 600 points and plots @ 100Hz
data_connector = DataConnector(plot_curve, max_points=600, update_rate=100)
def sin_wave_generator(connector):
"""Sine wave generator"""
x = 0
while running:
x += 1
data_point = sin(x * 0.01)
# Callback to plot new data point
connector.cb_append_data_point(data_point, x)
sleep(0.01)
plot_widget.show()
# Start sin_wave_generator in new Thread and send data to data_connector
Thread(target=sin_wave_generator, args=(data_connector,)).start()
app.exec()
running = False
```
Output:
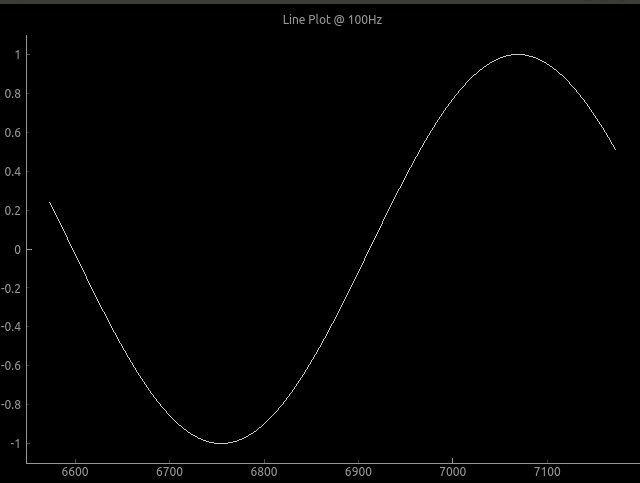
To run built-in examples, use python3 -m parameter like:
`python3 -m pglive.examples_pyqt6.all_plot_types`
`python3 -m pglive.examples_pyqt6.crosshair`
# Using PyQt5/6 designer #
1. Add QWidget to Your layout
2. Promote QWidget to `LivePlotWidget` and set header file to `pglive.sources.live_plot_widget`
3. Click `Add` and `Promote` button
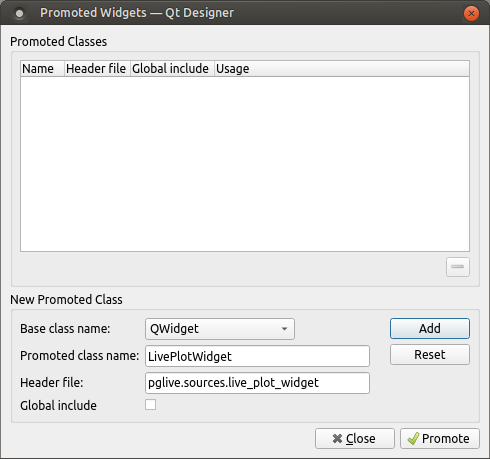
# Available plot types #
Pglive supports four plot types: `LiveLinePlot`, `LiveScatterPlot`, `LiveHBarPlot` (horizontal bar plot),
`LiveVBarPlot` (vertical bar plot) and `LiveCandleStickPlot`.
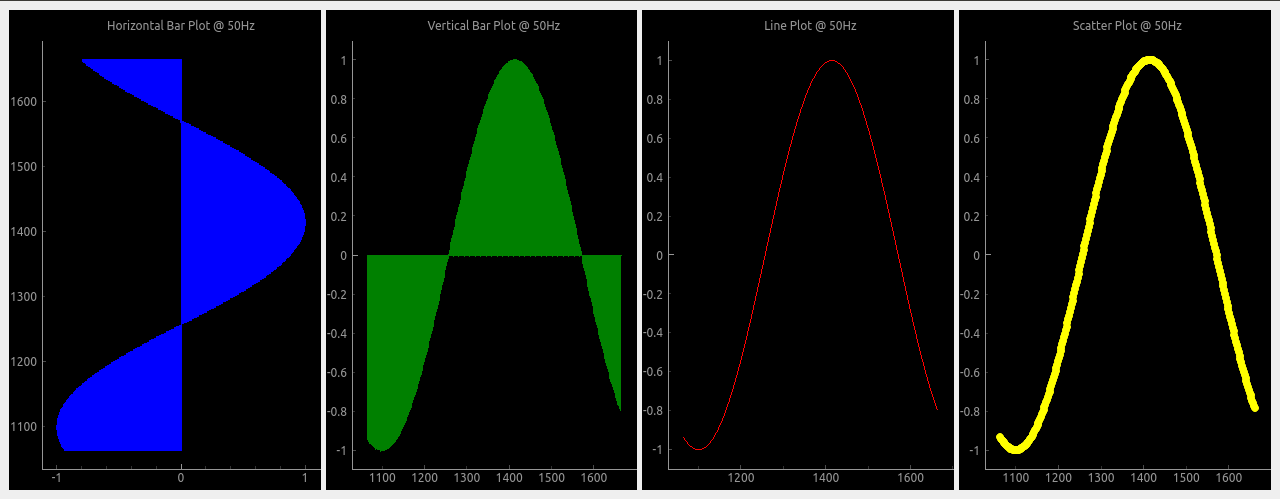
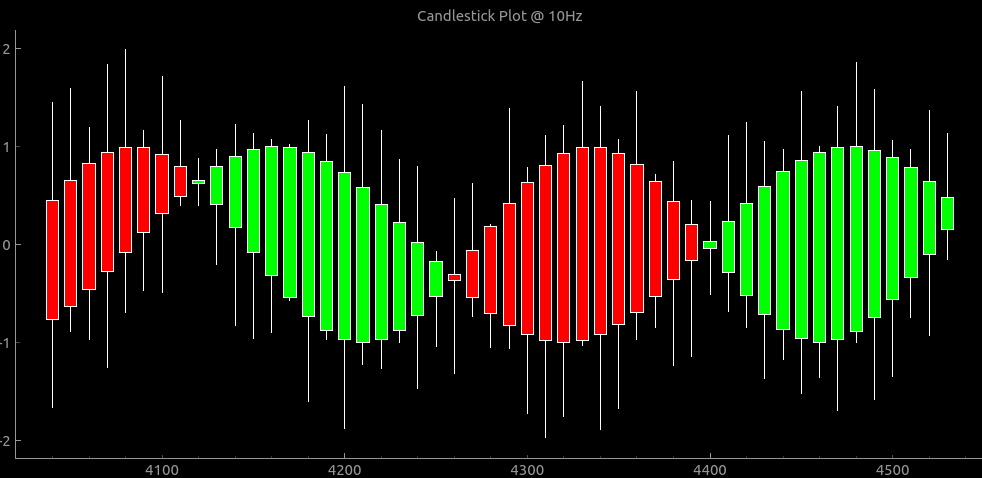
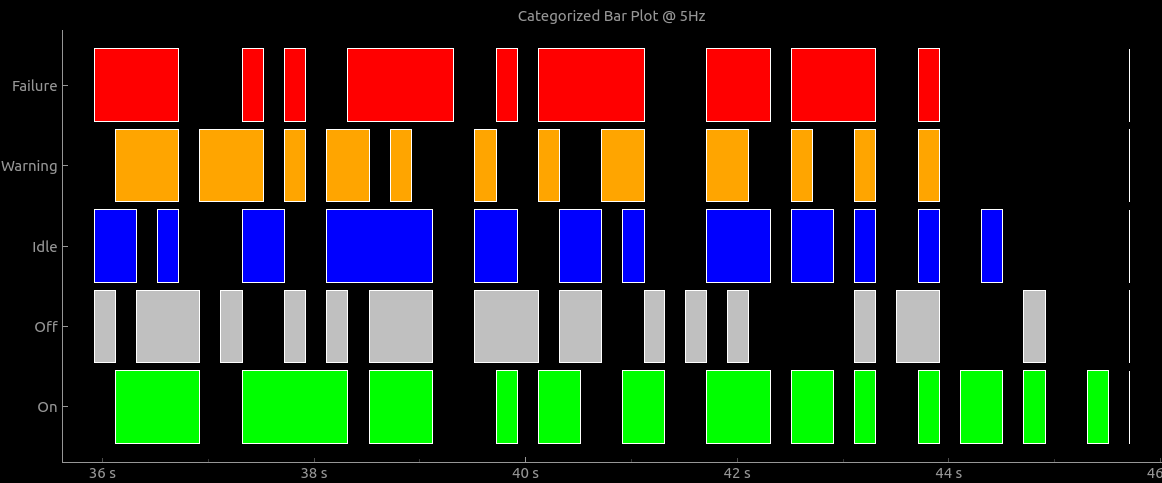
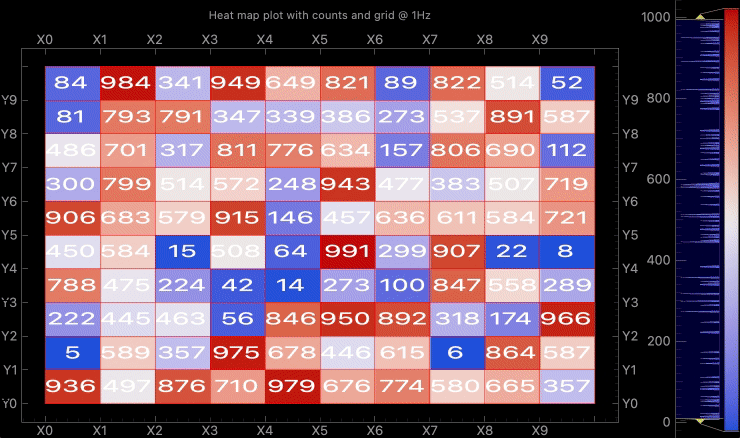
# Plot speed optimizations #
Scaling plot view to plotted data has a huge impact on plotting performance.
Re-plotting might be laggy when using high update frequencies and multiple plots.
To increase plotting performance, pglive introduces `LiveAxisRange`, that can be used in `LivePlotWidget`.
User can specify when and how is a new view of plotted data calculated.
Have a look in the [live_plot_range.py](https://github.com/domarm-comat/pglive/blob/main/pglive/examples_pyqt6/live_plot_range.py) example.
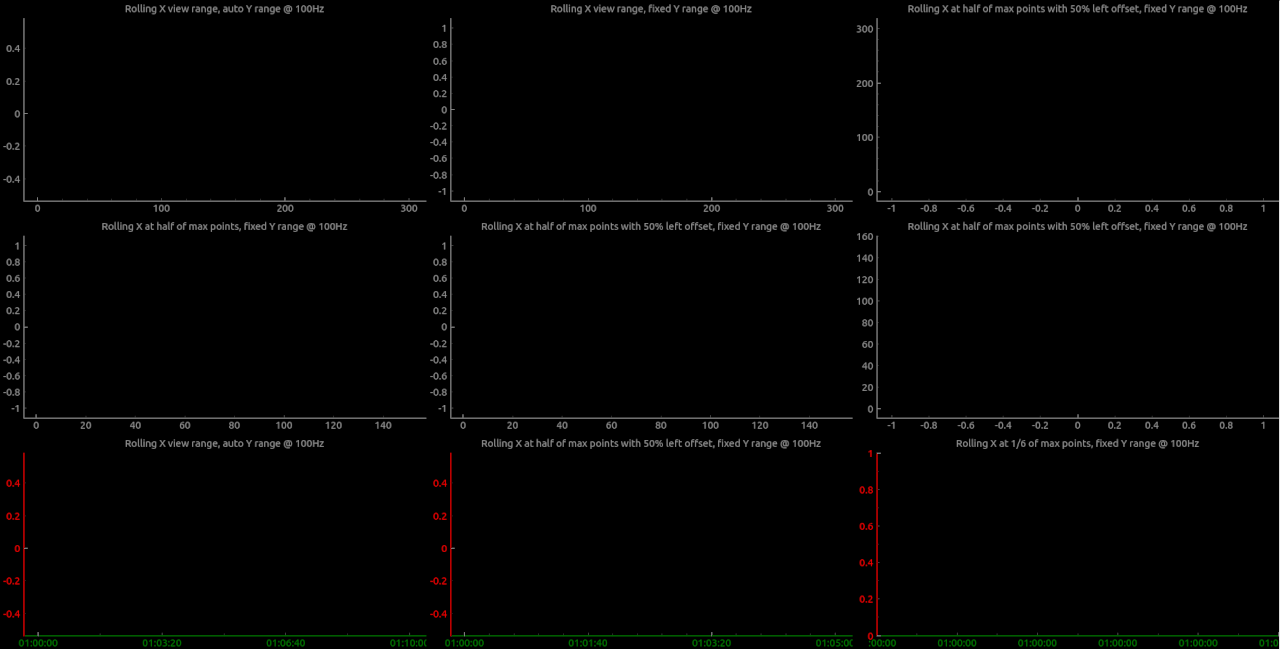
In case you want to plot wider area with LiveAxisRange you can use `crop_offset_to_data` flag.
For example, you want to store 60 seconds, display 30 seconds in a view and move view every 1 second.
You will end up with big empty space to the left if `crop_offset_to_data = False`.
Take a look into [crop_offset_to_data.py](https://github.com/domarm-comat/pglive/blob/main/pglive/examples_pyqt6/crop_offset_to_data.py) example.
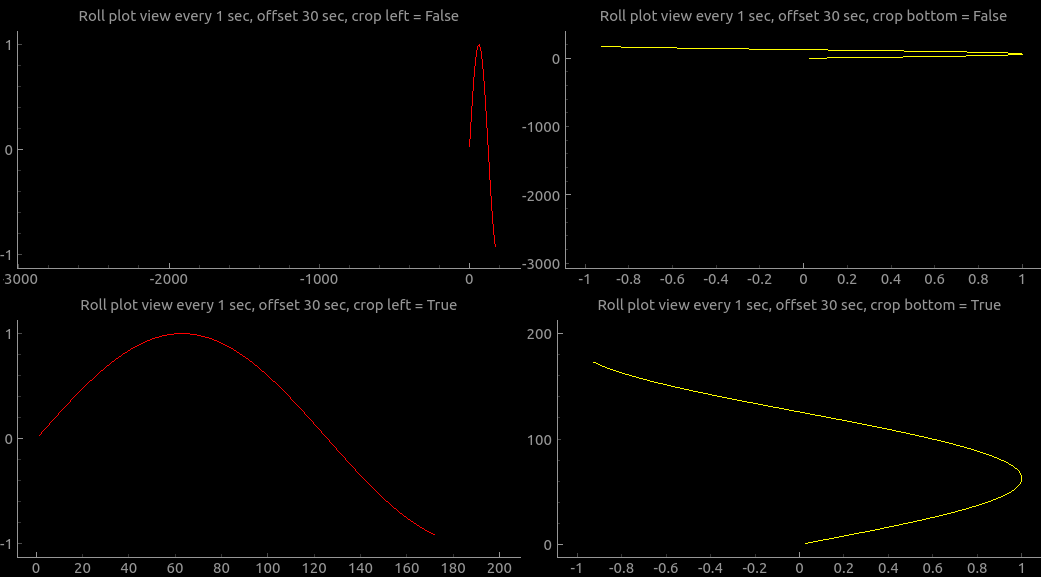
Introduced in *v0.4.0*
# Crosshair #
Pglive comes with built-in Crosshair as well. Take a look at [crosshair.py](https://github.com/domarm-comat/pglive/blob/main/pglive/examples_pyqt6/crosshair.py) example.
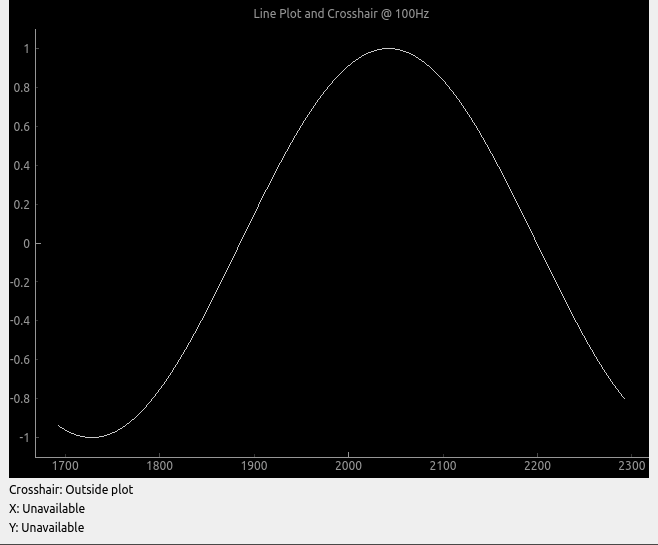
# Leading lines #
Leading line displays horizontal or vertical line (or both) at the last plotted point.
You can choose its color and which axis value is displayed along with it.
Example at [leading_line.py](https://github.com/domarm-comat/pglive/blob/main/pglive/examples_pyqt6/leading_line.py)
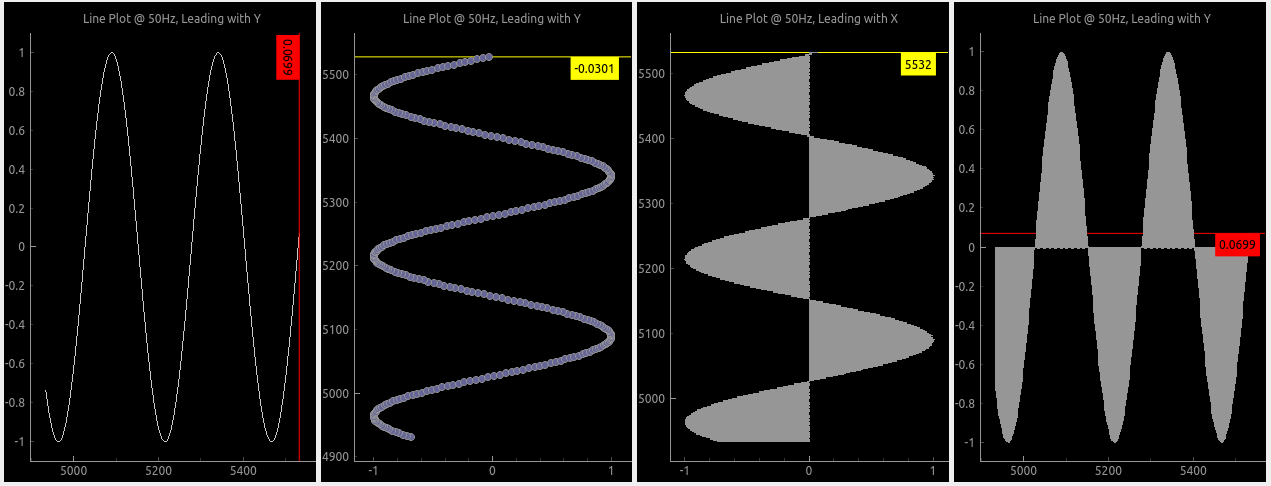
# Axis #
To make life easier, pglive includes a few axis improvements:
- Colored axis line using new `axisPen` attribute
- Time and DateTime tick format, converting timestamp into human-readable format
- Use `tick_angle` attribute to change tick angle from 0 default degree
Example at [axis.py](https://github.com/domarm-comat/pglive/blob/main/pglive/examples_pyqt6/axis.py)
[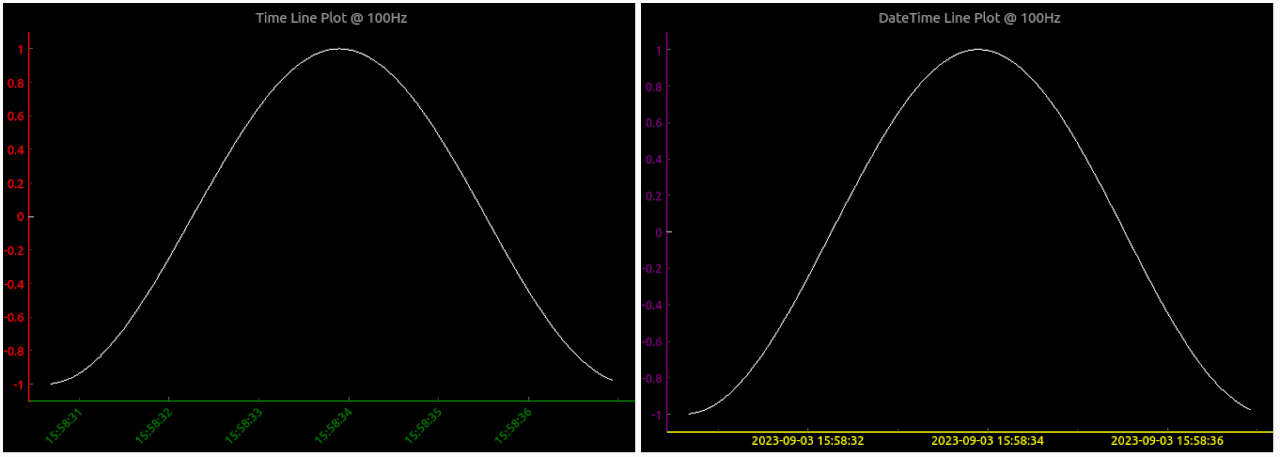](https://postimg.cc/RqBy04V6)
# Summary #
- With Pglive You've got an easy Thread-safe live plot implementation in Pyqt5, Pyqt6 or PySide6
- You can use all `kwargs` that works in [pyqtgraph](https://pyqtgraph.readthedocs.io/en/latest/getting_started/index.html#getting-started-ref)
- Use your plots with `DataConnector` directly
- It works with Python3.9, 3.10, 3.11 and 3.12 as well
- Multiple optimized plot types
- Many examples for easy start
If you find PgLive helpful, please consider [supporting me](https://ko-fi.com/domarmcomatsk), it helps a lot!
Thanks to all contributors, feel free to suggest missing feature or any bug on [GitHub](https://github.com/domarm-comat/pglive/issues).
Raw data
{
"_id": null,
"home_page": "https://github.com/domarm-comat/pglive",
"name": "pglive",
"maintainer": null,
"docs_url": null,
"requires_python": "<3.13,>=3.9",
"maintainer_email": null,
"keywords": "pyqtgraph, realtime, live, plotting, pyqt5, pyqt6, pyside6",
"author": "Martin Domaracky",
"author_email": "domarm@comat.sk",
"download_url": "https://files.pythonhosted.org/packages/38/64/6ccc234fdff1965125d610aca5733165f24c4508b9ae0a623f923a941068/pglive-0.8.1.tar.gz",
"platform": null,
"description": "# Live pyqtgraph plot\n\nPglive package adds support for thread-safe live plotting based on pyqtgraph. \nIt supports PyQt5, PyQt6 and PySide6.\n\n# Description #\n\nPyqtgraph doesn't offer easy way to implement live plotting out of the box.\nThe aim of PgLive module is to provide easy way of thread-safe live plotting.\nTo do this, PgLive provides DataConnector object, which consumes data \nand manages data plotting. DataConnector interface provides Pause and Resume method, update rate and maximum number of\nplotted points. All that needs to be done is to connect plots and data sources with DataConnector.\nOnce data is collected, DataConnector is sending signals to the GUI main loop.\n\n**Focus on data handling - leave plotting to pglive.**\n\nYou can find many examples for PyQt5, PyQt6 or PySide6.\n\n# Code example #\n\n```python\nimport sys\nfrom math import sin\nfrom threading import Thread\nfrom time import sleep\n\nfrom PyQt6.QtWidgets import QApplication\n\nfrom pglive.sources.data_connector import DataConnector\nfrom pglive.sources.live_plot import LiveLinePlot\nfrom pglive.sources.live_plot_widget import LivePlotWidget\n\n\"\"\"\nLine plot is displayed in this example.\n\"\"\"\napp = QApplication(sys.argv)\nrunning = True\n\nplot_widget = LivePlotWidget(title=\"Line Plot @ 100Hz\")\nplot_curve = LiveLinePlot()\nplot_widget.addItem(plot_curve)\n# DataConnector holding 600 points and plots @ 100Hz\ndata_connector = DataConnector(plot_curve, max_points=600, update_rate=100)\n\n\ndef sin_wave_generator(connector):\n \"\"\"Sine wave generator\"\"\"\n x = 0\n while running:\n x += 1\n data_point = sin(x * 0.01)\n # Callback to plot new data point\n connector.cb_append_data_point(data_point, x)\n sleep(0.01)\n\n\nplot_widget.show()\n# Start sin_wave_generator in new Thread and send data to data_connector\nThread(target=sin_wave_generator, args=(data_connector,)).start()\napp.exec()\nrunning = False\n```\n\nOutput:\n\n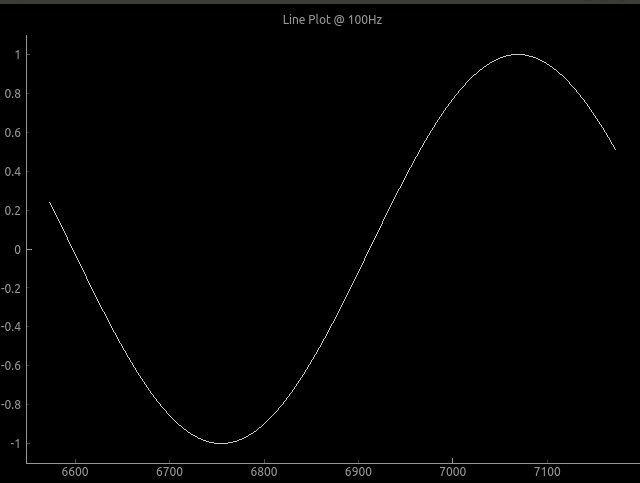\n\nTo run built-in examples, use python3 -m parameter like: \n`python3 -m pglive.examples_pyqt6.all_plot_types` \n`python3 -m pglive.examples_pyqt6.crosshair`\n\n# Using PyQt5/6 designer #\n\n1. Add QWidget to Your layout\n2. Promote QWidget to `LivePlotWidget` and set header file to `pglive.sources.live_plot_widget`\n3. Click `Add` and `Promote` button\n\n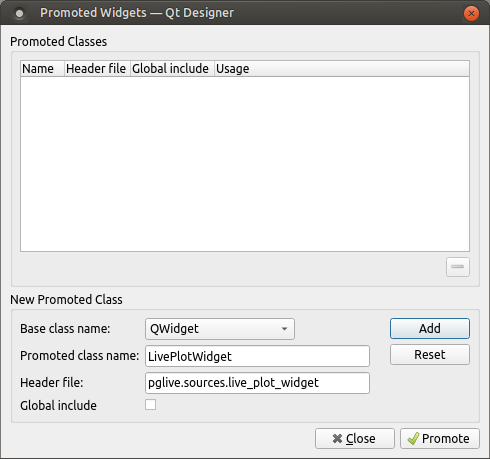\n\n# Available plot types #\n\nPglive supports four plot types: `LiveLinePlot`, `LiveScatterPlot`, `LiveHBarPlot` (horizontal bar plot),\n`LiveVBarPlot` (vertical bar plot) and `LiveCandleStickPlot`.\n\n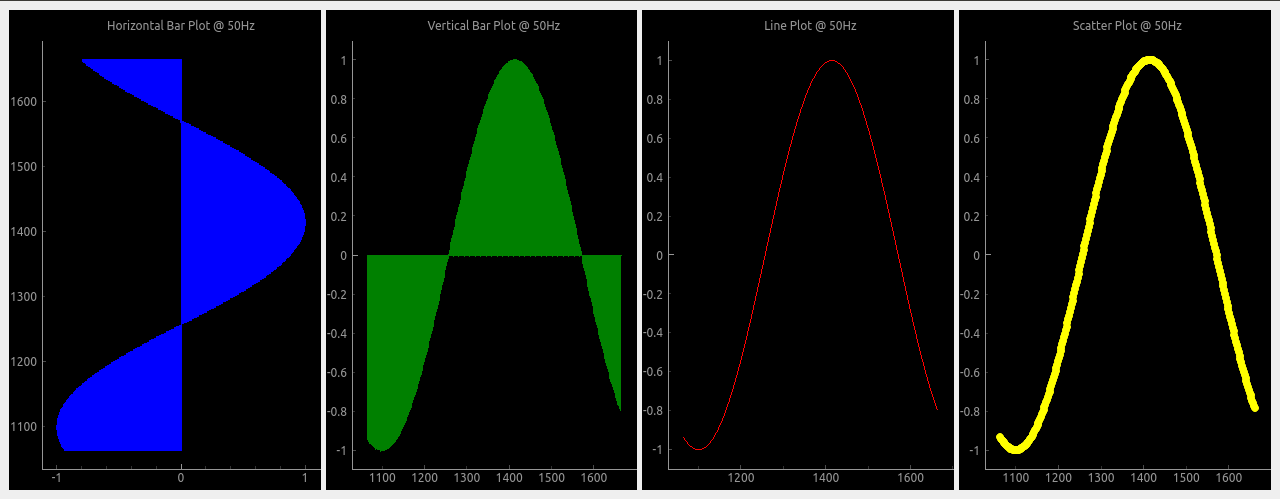\n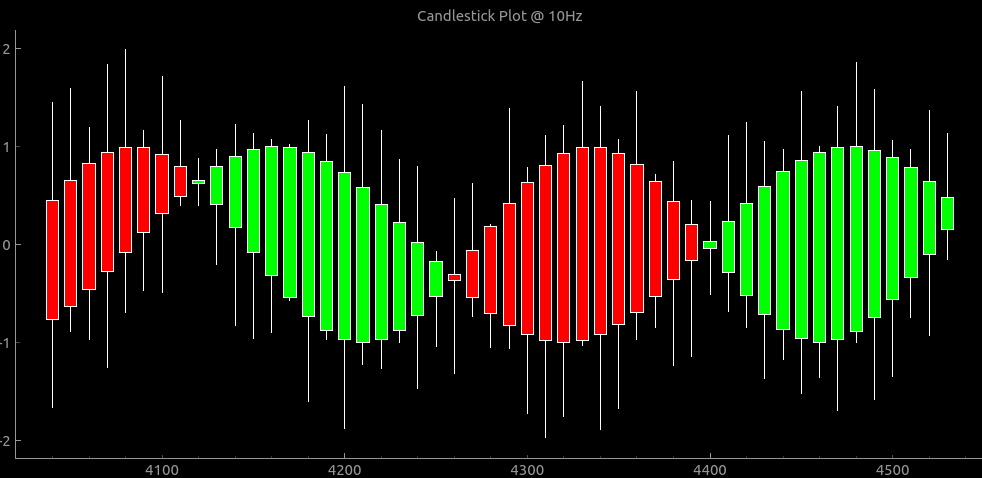\n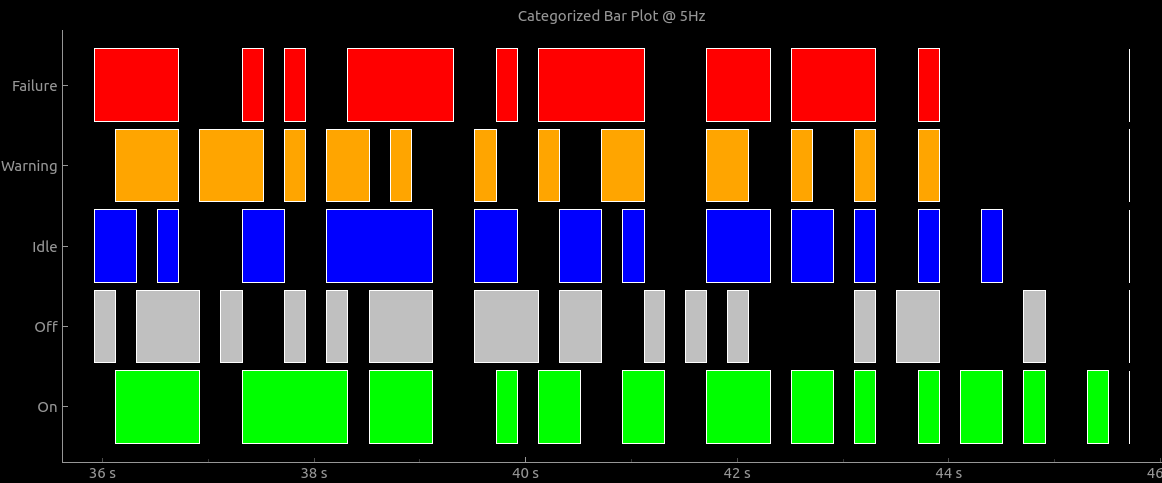\n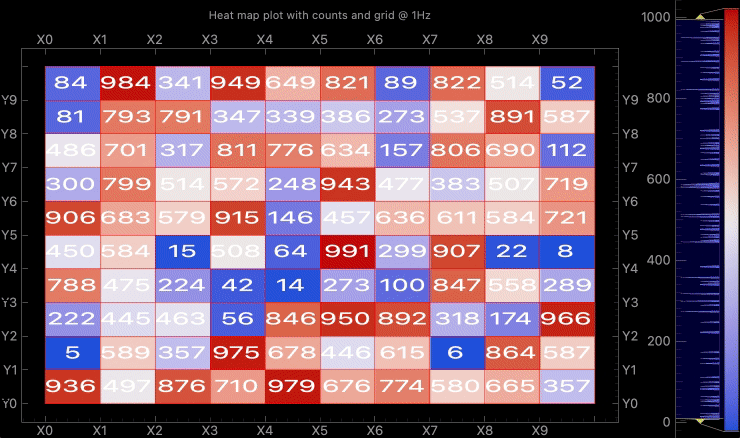\n\n# Plot speed optimizations #\n\nScaling plot view to plotted data has a huge impact on plotting performance.\nRe-plotting might be laggy when using high update frequencies and multiple plots. \nTo increase plotting performance, pglive introduces `LiveAxisRange`, that can be used in `LivePlotWidget`.\nUser can specify when and how is a new view of plotted data calculated.\n\nHave a look in the [live_plot_range.py](https://github.com/domarm-comat/pglive/blob/main/pglive/examples_pyqt6/live_plot_range.py) example.\n\n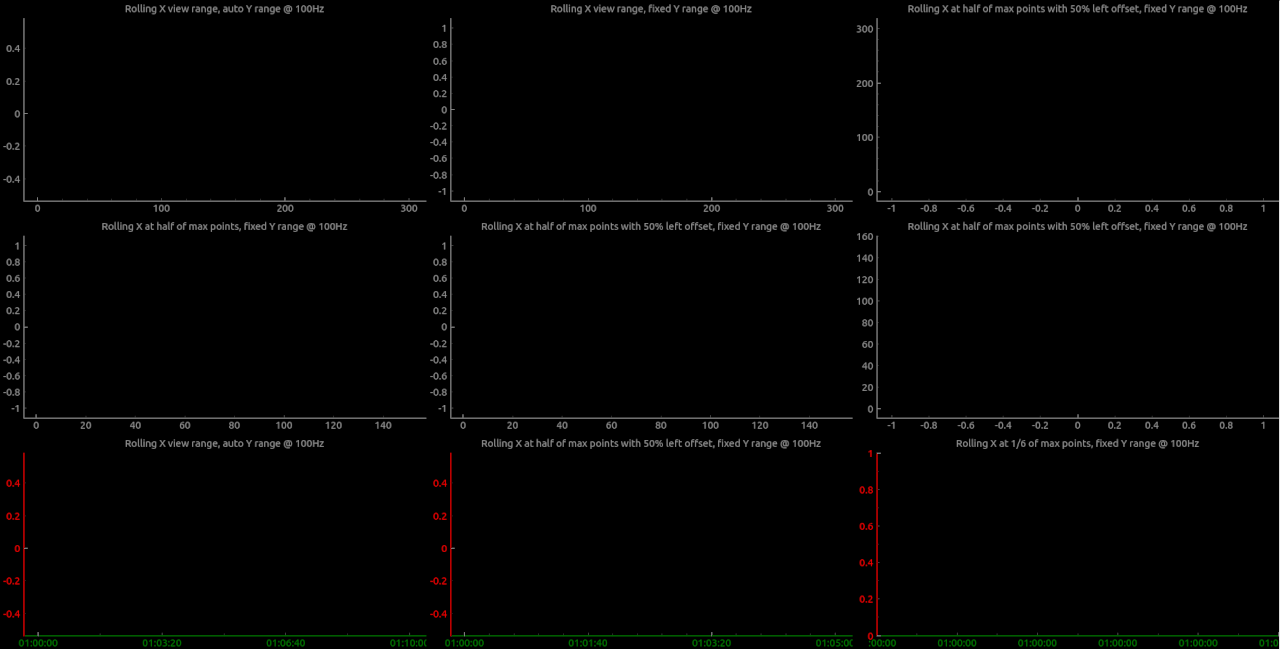\n\nIn case you want to plot wider area with LiveAxisRange you can use `crop_offset_to_data` flag.\nFor example, you want to store 60 seconds, display 30 seconds in a view and move view every 1 second.\nYou will end up with big empty space to the left if `crop_offset_to_data = False`.\nTake a look into [crop_offset_to_data.py](https://github.com/domarm-comat/pglive/blob/main/pglive/examples_pyqt6/crop_offset_to_data.py) example.\n\n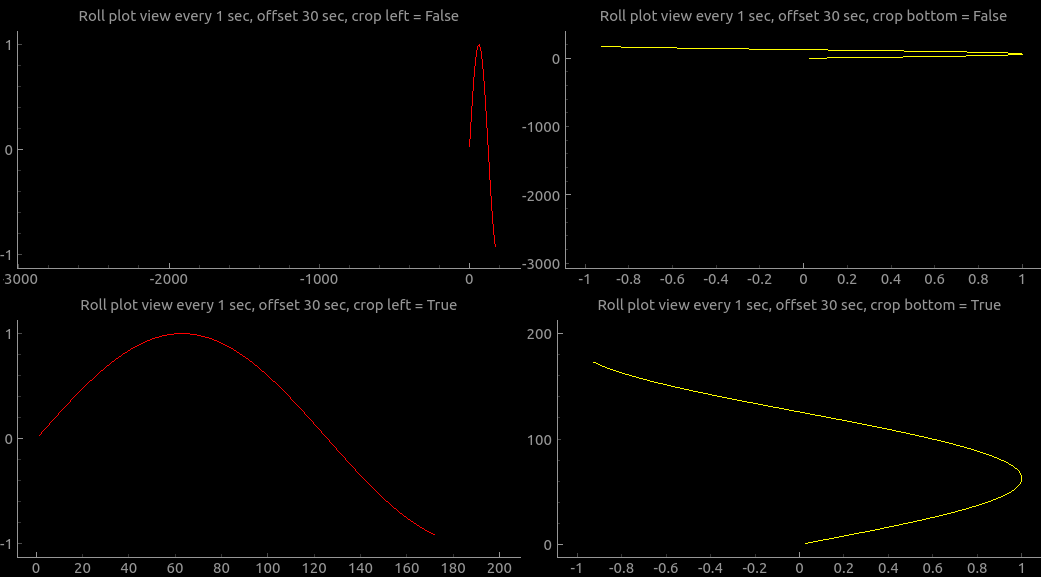\n\nIntroduced in *v0.4.0*\n\n# Crosshair #\n\nPglive comes with built-in Crosshair as well. Take a look at [crosshair.py](https://github.com/domarm-comat/pglive/blob/main/pglive/examples_pyqt6/crosshair.py) example.\n\n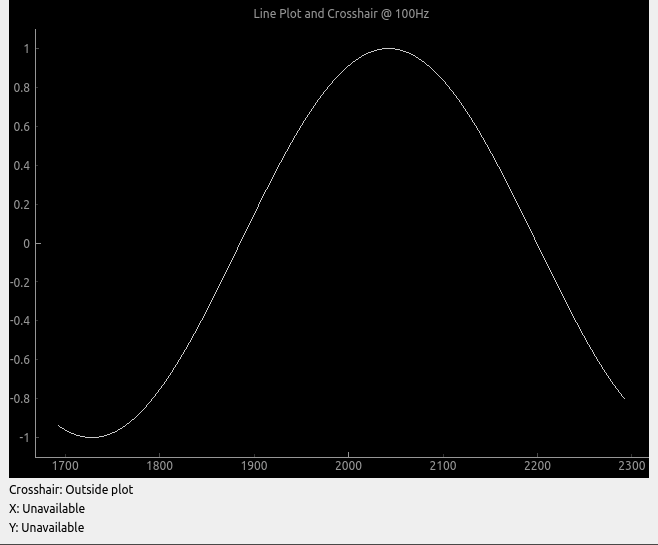\n\n# Leading lines #\n\nLeading line displays horizontal or vertical line (or both) at the last plotted point. \nYou can choose its color and which axis value is displayed along with it. \nExample at [leading_line.py](https://github.com/domarm-comat/pglive/blob/main/pglive/examples_pyqt6/leading_line.py)\n\n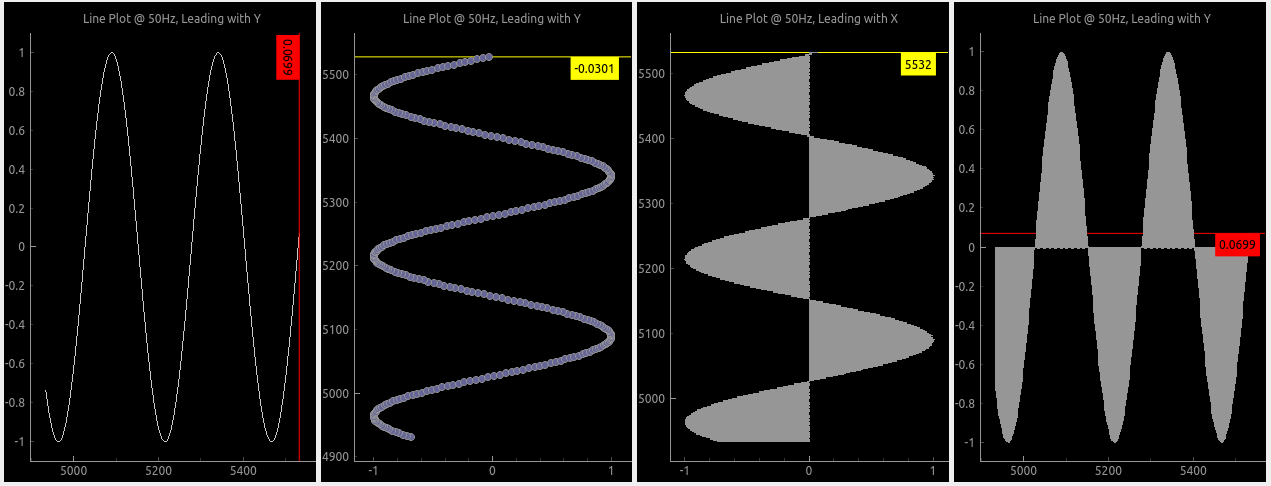\n\n# Axis #\n\nTo make life easier, pglive includes a few axis improvements:\n\n- Colored axis line using new `axisPen` attribute\n- Time and DateTime tick format, converting timestamp into human-readable format\n- Use `tick_angle` attribute to change tick angle from 0 default degree \n\nExample at [axis.py](https://github.com/domarm-comat/pglive/blob/main/pglive/examples_pyqt6/axis.py)\n\n[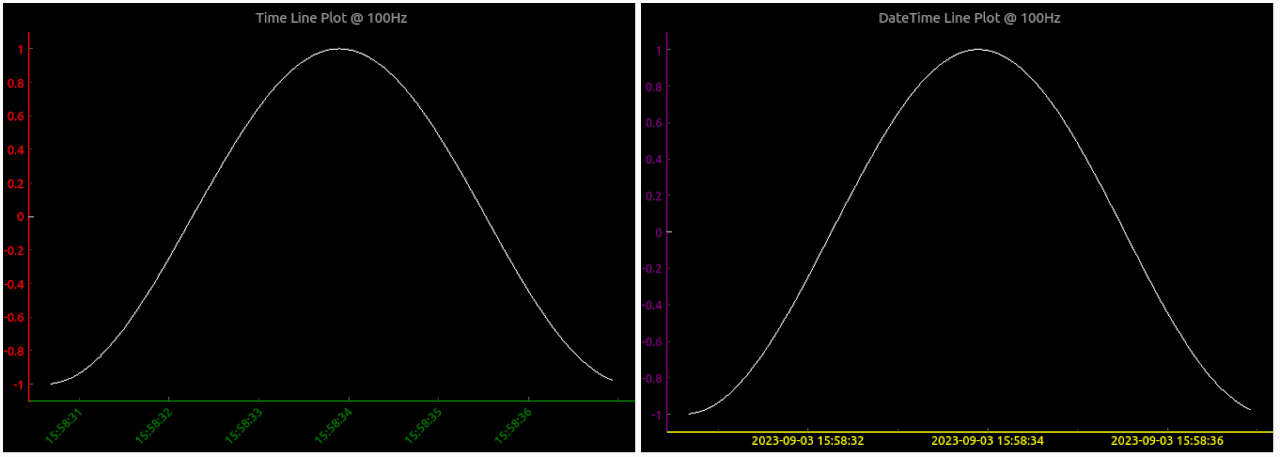](https://postimg.cc/RqBy04V6)\n\n# Summary #\n\n- With Pglive You've got an easy Thread-safe live plot implementation in Pyqt5, Pyqt6 or PySide6\n- You can use all `kwargs` that works in [pyqtgraph](https://pyqtgraph.readthedocs.io/en/latest/getting_started/index.html#getting-started-ref)\n- Use your plots with `DataConnector` directly\n- It works with Python3.9, 3.10, 3.11 and 3.12 as well\n- Multiple optimized plot types\n- Many examples for easy start\n\nIf you find PgLive helpful, please consider [supporting me](https://ko-fi.com/domarmcomatsk), it helps a lot! \nThanks to all contributors, feel free to suggest missing feature or any bug on [GitHub](https://github.com/domarm-comat/pglive/issues).\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Live plot for PyqtGraph",
"version": "0.8.1",
"project_urls": {
"Homepage": "https://github.com/domarm-comat/pglive",
"Repository": "https://github.com/domarm-comat/pglive"
},
"split_keywords": [
"pyqtgraph",
" realtime",
" live",
" plotting",
" pyqt5",
" pyqt6",
" pyside6"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "dcda198ab922952954b212ab98dbc70526fa04d495021cf9136d6975dd28100e",
"md5": "6915e282ea28e74240d45fc6c759e6ae",
"sha256": "d202f50694fbe7d4d278e7dfaa4b49c934f8e627197fe38f29a429a51c15f7a0"
},
"downloads": -1,
"filename": "pglive-0.8.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "6915e282ea28e74240d45fc6c759e6ae",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<3.13,>=3.9",
"size": 79311,
"upload_time": "2025-02-14T09:42:31",
"upload_time_iso_8601": "2025-02-14T09:42:31.767979Z",
"url": "https://files.pythonhosted.org/packages/dc/da/198ab922952954b212ab98dbc70526fa04d495021cf9136d6975dd28100e/pglive-0.8.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "38646ccc234fdff1965125d610aca5733165f24c4508b9ae0a623f923a941068",
"md5": "457391e29e18db1719fb43412a08fa00",
"sha256": "c388a8a8d48a850c997092c78e53fd17bcefdddb4d6cd099363fd3573ce548d2"
},
"downloads": -1,
"filename": "pglive-0.8.1.tar.gz",
"has_sig": false,
"md5_digest": "457391e29e18db1719fb43412a08fa00",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<3.13,>=3.9",
"size": 45838,
"upload_time": "2025-02-14T09:42:33",
"upload_time_iso_8601": "2025-02-14T09:42:33.619291Z",
"url": "https://files.pythonhosted.org/packages/38/64/6ccc234fdff1965125d610aca5733165f24c4508b9ae0a623f923a941068/pglive-0.8.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-02-14 09:42:33",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "domarm-comat",
"github_project": "pglive",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [
{
"name": "numpy",
"specs": [
[
"==",
"1.26.4"
]
]
},
{
"name": "pyqtgraph",
"specs": [
[
"==",
"0.13.7"
]
]
}
],
"lcname": "pglive"
}