# Pomice
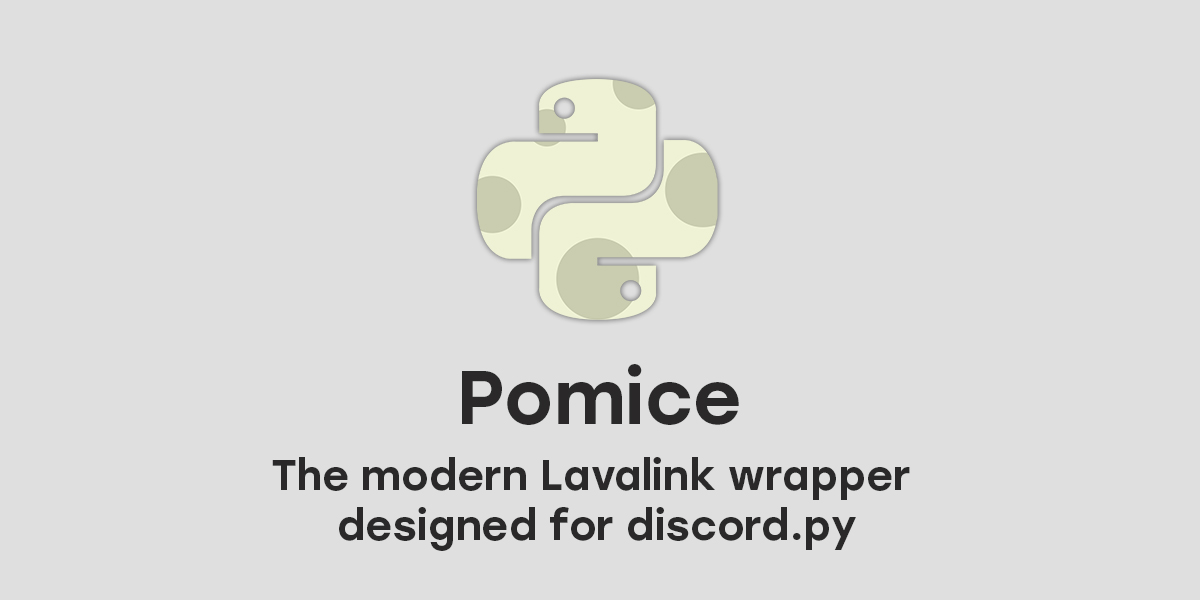
[](https://github.com/cloudwithax/pomice/blob/main/LICENSE)  [](https://github.com/psf/black)
[](https://discord.gg/r64qjTSHG8) [](https://pomice.readthedocs.io/en/latest/)
Pomice is a fully asynchronous Python library designed for communicating with [Lavalink](https://github.com/freyacodes/Lavalink) seamlessly within the [discord.py](https://github.com/Rapptz/discord.py) library. It features 100% coverage of the [Lavalink](https://github.com/freyacodes/Lavalink) spec that can be accessed with easy-to-understand functions along with Spotify and Apple Music querying capabilities using built-in custom clients, making it easier to develop your next big music bot.
## Quick Links
- [Discord Server](https://discord.gg/r64qjTSHG8)
- [Read the Docs](https://pomice.readthedocs.io/en/latest/)
- [PyPI Homepage](https://pypi.org/project/pomice/)
# Install
To install the library, you need the lastest version of pip and minimum Python 3.8
> Stable version
```
pip install pomice
```
> Unstable version (this one gets more frequent changes)
```
pip install git+https://github.com/cloudwithax/pomice
```
# Support And Documentation
The official documentation is [here](https://pomice.readthedocs.io/en/latest/)
You can join our support server [here](https://discord.gg/r64qjTSHG8)
# Examples
In-depth examples are located in the [examples folder](https://github.com/cloudwithax/pomice/tree/main/examples)
Here's a quick example:
```py
import pomice
import discord
import re
from discord.ext import commands
URL_REG = re.compile(r'https?://(?:www\.)?.+')
class MyBot(commands.Bot):
def __init__(self) -> None:
super().__init__(command_prefix='!', activity=discord.Activity(type=discord.ActivityType.listening, name='to music!'))
self.add_cog(Music(self))
async def on_ready(self) -> None:
print("I'm online!")
await self.cogs["Music"].start_nodes()
class Music(commands.Cog):
def __init__(self, bot) -> None:
self.bot = bot
self.pomice = pomice.NodePool()
async def start_nodes(self):
await self.pomice.create_node(bot=self.bot, host='127.0.0.1', port='3030',
password='youshallnotpass', identifier='MAIN')
print(f"Node is ready!")
@commands.command(name='join', aliases=['connect'])
async def join(self, ctx: commands.Context, *, channel: discord.TextChannel = None) -> None:
if not channel:
channel = getattr(ctx.author.voice, 'channel', None)
if not channel:
raise commands.CheckFailure('You must be in a voice channel to use this command'
'without specifying the channel argument.')
await ctx.author.voice.channel.connect(cls=pomice.Player)
await ctx.send(f'Joined the voice channel `{channel}`')
@commands.command(name='play')
async def play(self, ctx, *, search: str) -> None:
if not ctx.voice_client:
await ctx.invoke(self.join)
player = ctx.voice_client
results = await player.get_tracks(query=f'{search}')
if not results:
raise commands.CommandError('No results were found for that search term.')
if isinstance(results, pomice.Playlist):
await player.play(track=results.tracks[0])
else:
await player.play(track=results[0])
bot = MyBot()
bot.run("token here")
```
# FAQ
Why is it saying "Cannot connect to host"?
- You need to have a Lavalink node setup before you can use this library. Download it [here](https://github.com/freyacodes/Lavalink/releases/latest)
What experience do I need?
- This library requires that you have some experience with Python, asynchronous programming and the discord.py library.
Why is it saying "No module named pomice found"?
- You need to [install](#Install) the package before you can use it
# Contributors
- Thanks to [vveeps](https://github.com/vveeps) for implementing some features I wasn't able to do myself
Raw data
{
"_id": null,
"home_page": "https://github.com/cloudwithax/pomice",
"name": "pomice",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "pomice, lavalink, discord.py",
"author": "cloudwithax",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/3e/9e/00236cfa40496b105bce07b759ca53fe65355f5fdefe87d1e31e2c3b7ffd/pomice-2.9.2.tar.gz",
"platform": null,
"description": "# Pomice\r\n\r\n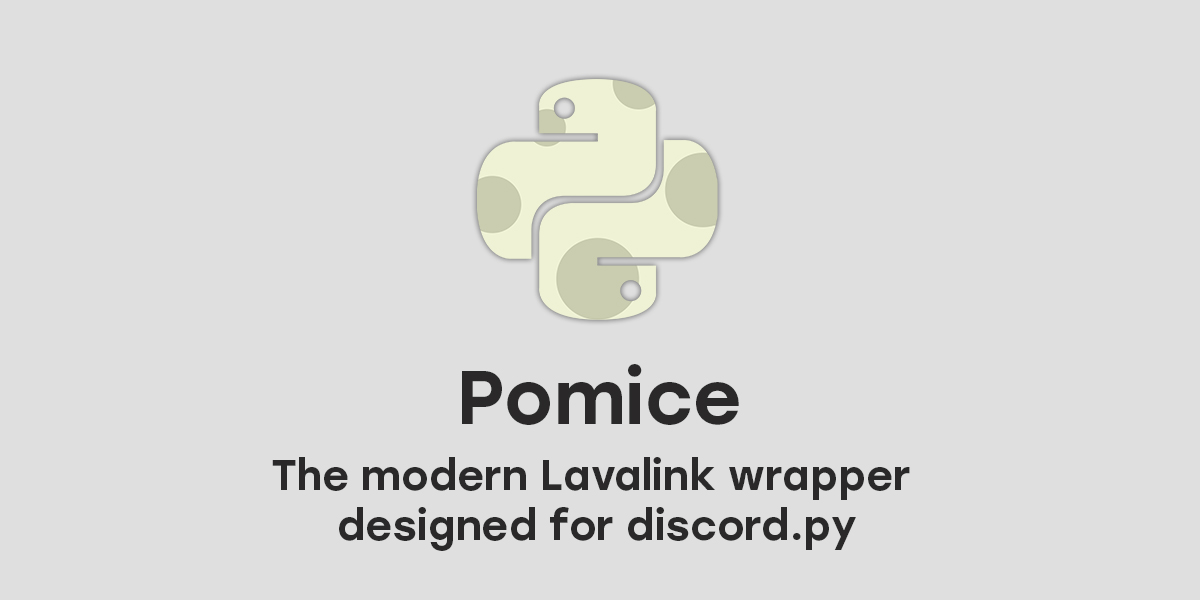\r\n\r\n\r\n[](https://github.com/cloudwithax/pomice/blob/main/LICENSE)  [](https://github.com/psf/black)\r\n[](https://discord.gg/r64qjTSHG8) [](https://pomice.readthedocs.io/en/latest/)\r\n\r\n\r\nPomice is a fully asynchronous Python library designed for communicating with [Lavalink](https://github.com/freyacodes/Lavalink) seamlessly within the [discord.py](https://github.com/Rapptz/discord.py) library. It features 100% coverage of the [Lavalink](https://github.com/freyacodes/Lavalink) spec that can be accessed with easy-to-understand functions along with Spotify and Apple Music querying capabilities using built-in custom clients, making it easier to develop your next big music bot.\r\n\r\n## Quick Links\r\n- [Discord Server](https://discord.gg/r64qjTSHG8)\r\n- [Read the Docs](https://pomice.readthedocs.io/en/latest/)\r\n- [PyPI Homepage](https://pypi.org/project/pomice/)\r\n\r\n\r\n# Install\r\nTo install the library, you need the lastest version of pip and minimum Python 3.8\r\n\r\n> Stable version\r\n```\r\npip install pomice\r\n```\r\n\r\n> Unstable version (this one gets more frequent changes)\r\n```\r\npip install git+https://github.com/cloudwithax/pomice\r\n```\r\n\r\n# Support And Documentation\r\n\r\nThe official documentation is [here](https://pomice.readthedocs.io/en/latest/)\r\n\r\nYou can join our support server [here](https://discord.gg/r64qjTSHG8)\r\n\r\n\r\n# Examples\r\nIn-depth examples are located in the [examples folder](https://github.com/cloudwithax/pomice/tree/main/examples)\r\n\r\nHere's a quick example:\r\n\r\n```py\r\nimport pomice\r\nimport discord\r\nimport re\r\n\r\nfrom discord.ext import commands\r\n\r\nURL_REG = re.compile(r'https?://(?:www\\.)?.+')\r\n\r\nclass MyBot(commands.Bot):\r\n\r\n def __init__(self) -> None:\r\n super().__init__(command_prefix='!', activity=discord.Activity(type=discord.ActivityType.listening, name='to music!'))\r\n\r\n self.add_cog(Music(self))\r\n\r\n async def on_ready(self) -> None:\r\n print(\"I'm online!\")\r\n await self.cogs[\"Music\"].start_nodes()\r\n\r\n\r\nclass Music(commands.Cog):\r\n\r\n def __init__(self, bot) -> None:\r\n self.bot = bot\r\n\r\n self.pomice = pomice.NodePool()\r\n\r\n async def start_nodes(self):\r\n await self.pomice.create_node(bot=self.bot, host='127.0.0.1', port='3030',\r\n password='youshallnotpass', identifier='MAIN')\r\n print(f\"Node is ready!\")\r\n\r\n\r\n\r\n @commands.command(name='join', aliases=['connect'])\r\n async def join(self, ctx: commands.Context, *, channel: discord.TextChannel = None) -> None:\r\n\r\n if not channel:\r\n channel = getattr(ctx.author.voice, 'channel', None)\r\n if not channel:\r\n raise commands.CheckFailure('You must be in a voice channel to use this command'\r\n 'without specifying the channel argument.')\r\n\r\n\r\n await ctx.author.voice.channel.connect(cls=pomice.Player)\r\n await ctx.send(f'Joined the voice channel `{channel}`')\r\n\r\n @commands.command(name='play')\r\n async def play(self, ctx, *, search: str) -> None:\r\n\r\n if not ctx.voice_client:\r\n await ctx.invoke(self.join)\r\n\r\n player = ctx.voice_client\r\n\r\n results = await player.get_tracks(query=f'{search}')\r\n\r\n if not results:\r\n raise commands.CommandError('No results were found for that search term.')\r\n\r\n if isinstance(results, pomice.Playlist):\r\n await player.play(track=results.tracks[0])\r\n else:\r\n await player.play(track=results[0])\r\n\r\n\r\nbot = MyBot()\r\nbot.run(\"token here\")\r\n ```\r\n\r\n# FAQ\r\nWhy is it saying \"Cannot connect to host\"?\r\n\r\n- You need to have a Lavalink node setup before you can use this library. Download it [here](https://github.com/freyacodes/Lavalink/releases/latest)\r\n\r\nWhat experience do I need?\r\n\r\n- This library requires that you have some experience with Python, asynchronous programming and the discord.py library.\r\n\r\nWhy is it saying \"No module named pomice found\"?\r\n\r\n- You need to [install](#Install) the package before you can use it\r\n\r\n# Contributors\r\n\r\n- Thanks to [vveeps](https://github.com/vveeps) for implementing some features I wasn't able to do myself\r\n",
"bugtrack_url": null,
"license": "GPL",
"summary": "The modern Lavalink wrapper designed for Discord.py",
"version": "2.9.2",
"project_urls": {
"Homepage": "https://github.com/cloudwithax/pomice"
},
"split_keywords": [
"pomice",
" lavalink",
" discord.py"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "63e1aef0f593f1911f4a922fa343a8dc77f2621972f7d3e383a2f79274e7d1e6",
"md5": "a5d819fabe285f8430e70147ce49b712",
"sha256": "6c2d97e6e1780f7ba86337db954d4171d93f35cc0d48a76226a4313f6d6a1353"
},
"downloads": -1,
"filename": "pomice-2.9.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "a5d819fabe285f8430e70147ce49b712",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 54065,
"upload_time": "2024-11-22T02:12:13",
"upload_time_iso_8601": "2024-11-22T02:12:13.131959Z",
"url": "https://files.pythonhosted.org/packages/63/e1/aef0f593f1911f4a922fa343a8dc77f2621972f7d3e383a2f79274e7d1e6/pomice-2.9.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "3e9e00236cfa40496b105bce07b759ca53fe65355f5fdefe87d1e31e2c3b7ffd",
"md5": "30029af548390a8dc03daf4c966dfd26",
"sha256": "d2933c5fde3ea40158ff97a7347780f84214fb3f64d6ae6ad80cfd0b9e99104a"
},
"downloads": -1,
"filename": "pomice-2.9.2.tar.gz",
"has_sig": false,
"md5_digest": "30029af548390a8dc03daf4c966dfd26",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 50740,
"upload_time": "2024-11-22T02:12:14",
"upload_time_iso_8601": "2024-11-22T02:12:14.270257Z",
"url": "https://files.pythonhosted.org/packages/3e/9e/00236cfa40496b105bce07b759ca53fe65355f5fdefe87d1e31e2c3b7ffd/pomice-2.9.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-11-22 02:12:14",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "cloudwithax",
"github_project": "pomice",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "pomice"
}