[](https://github.com/pyamg/pyamg/actions?query=workflow%3ACI-test+branch%3Amain)
[](https://github.com/pyamg/pyamg/actions?query=workflow%3ACI-lint+branch%3Amain)
[](https://pypi.org/project/pyamg/)
[](https://pypi.org/project/pyamg/)
[](https://app.codecov.io/gh/pyamg/pyamg)
[](https://doi.org/10.21105/joss.05495)
# Installation
PyAMG requires `numpy` and `scipy`
```
pip install pyamg
```
or from source:
```
pip install .
```
(`python setup.py install` will no longer work)
or with conda (see details below)
```
conda config --add channels conda-forge
conda install pyamg
```
# Introduction
PyAMG is a library of **Algebraic Multigrid (AMG)** solvers with a convenient Python interface.
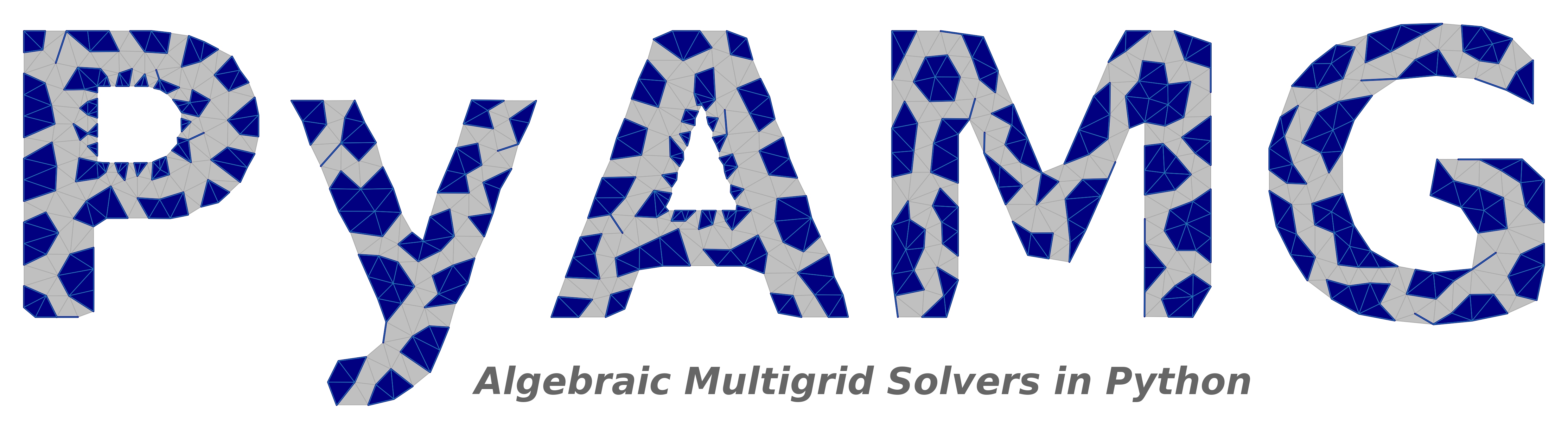
PyAMG is currently developed and maintained by
[Luke Olson](http://lukeo.cs.illinois.edu),
[Jacob Schroder](https://www.unm.edu/~jbschroder), and
[Ben Southworth](https://arxiv.org/a/southworth_b_1.html).
The organization of the project can be found in [`organization.md`](organization.md) and
examples of use can be found in [`pyamg-examples`](https://github.com/pyamg/pyamg-examples).
**Acknowledgements:**
PyAMG was created by
[Nathan Bell](http://wnbell.com/),
[Luke Olson](http://lukeo.cs.illinois.edu), and
[Jacob Schroder](https://www.unm.edu/~jbschroder).
Portions of the project were partially supported by the NSF under award DMS-0612448.
# Citing
If you use PyAMG in your work, please consider using the following citation:
<pre>
@article{pyamg2023,
author = {Nathan Bell and Luke N. Olson and Jacob Schroder and Ben Southworth},
title = {{PyAMG}: Algebraic Multigrid Solvers in Python},
journal = {Journal of Open Source Software},
year = {2023},
publisher = {The Open Journal},
volume = {8},
number = {87},
pages = {5495},
doi = {10.21105/joss.05495},
url = {https://doi.org/10.21105/joss.05495},
}
</pre>
# Getting Help
- For documentation see [http://pyamg.readthedocs.io/en/latest/](http://pyamg.readthedocs.io/en/latest/).
- Create an [issue](https://github.com/pyamg/pyamg/issues).
- Look at the [Tutorial](https://github.com/pyamg/pyamg/wiki/Tutorial) or the [examples](https://github.com/pyamg/pyamg-examples) (for instance the [0_start_here](https://github.com/pyamg/pyamg-examples/blob/main/0_start_here/demo.py) example).
- Run the unit tests (`pip install pytest`):
- With PyAMG installed and from a non-source directory:
```python
import pyamg
pyamg.test()
```
- From the PyAMG source directory and installed (e.g. with `pip install -e .`):
```python
pytest .
```
# What is AMG?
AMG is a multilevel technique for solving large-scale linear systems with optimal or near-optimal efficiency. Unlike geometric multigrid, AMG requires little or no geometric information about the underlying problem and develops a sequence of coarser grids directly from the input matrix. This feature is especially important for problems discretized on unstructured meshes and irregular grids.
# PyAMG Features
PyAMG features implementations of:
- **Ruge-Stuben (RS)** or *Classical AMG*
- AMG based on **Smoothed Aggregation (SA)**
and experimental support for:
- **Adaptive Smoothed Aggregation (αSA)**
- **Compatible Relaxation (CR)**
The predominant portion of PyAMG is written in Python with a smaller amount of supporting C++ code for performance critical operations.
# Example Usage
PyAMG is easy to use! The following code constructs a two-dimensional Poisson problem and solves the resulting linear system with Classical AMG.
````python
import pyamg
import numpy as np
A = pyamg.gallery.poisson((500,500), format='csr') # 2D Poisson problem on 500x500 grid
ml = pyamg.ruge_stuben_solver(A) # construct the multigrid hierarchy
print(ml) # print hierarchy information
b = np.random.rand(A.shape[0]) # pick a random right hand side
x = ml.solve(b, tol=1e-10) # solve Ax=b to a tolerance of 1e-10
print("residual: ", np.linalg.norm(b-A*x)) # compute norm of residual vector
````
Program output:
<pre>
multilevel_solver
Number of Levels: 9
Operator Complexity: 2.199
Grid Complexity: 1.667
Coarse Solver: 'pinv2'
level unknowns nonzeros
0 250000 1248000 [45.47%]
1 125000 1121002 [40.84%]
2 31252 280662 [10.23%]
3 7825 70657 [ 2.57%]
4 1937 17971 [ 0.65%]
5 483 4725 [ 0.17%]
6 124 1352 [ 0.05%]
7 29 293 [ 0.01%]
8 7 41 [ 0.00%]
residual: 1.24748994988e-08
</pre>
# Conda
More information can be found at [conda-forge/pyamg-feedstock](https://github.com/conda-forge/pyamg-feedstock).
*Linux:*
[](https://circleci.com/gh/conda-forge/pyamg-feedstock)
*OSX:*
[](https://travis-ci.org/conda-forge/pyamg-feedstock)
*Windows:*
[](https://ci.appveyor.com/project/conda-forge/pyamg-feedstock/branch/master)
*Version:*
[](https://anaconda.org/conda-forge/pyamg)
*Downloads:*
[](https://anaconda.org/conda-forge/pyamg)
Installing `pyamg` from the `conda-forge` channel can be achieved by adding `conda-forge` to your channels with:
```
conda config --add channels conda-forge
```
Once the `conda-forge` channel has been enabled, `pyamg` can be installed with:
```
conda install pyamg
```
It is possible to list all of the versions of `pyamg` available on your platform with:
```
conda search pyamg --channel conda-forge
```
Raw data
{
"_id": null,
"home_page": "https://github.com/pyamg/pyamg",
"name": "pyamg",
"maintainer": "Luke Olson",
"docs_url": null,
"requires_python": ">=3.9",
"maintainer_email": "luke.olson@gmail.com",
"keywords": "algebraic multigrid, AMG, sparse matrix, preconditioning",
"author": "Nathan Bell, Luke Olson, and Jacob Schroder",
"author_email": "luke.olson@gmail.com",
"download_url": "https://github.com/pyamg/pyamg/releases",
"platform": null,
"description": "[](https://github.com/pyamg/pyamg/actions?query=workflow%3ACI-test+branch%3Amain)\n[](https://github.com/pyamg/pyamg/actions?query=workflow%3ACI-lint+branch%3Amain)\n[](https://pypi.org/project/pyamg/)\n[](https://pypi.org/project/pyamg/)\n[](https://app.codecov.io/gh/pyamg/pyamg)\n[](https://doi.org/10.21105/joss.05495)\n\n# Installation\nPyAMG requires `numpy` and `scipy`\n\n```\npip install pyamg\n```\n\nor from source:\n\n```\npip install .\n```\n\n(`python setup.py install` will no longer work)\n\nor with conda (see details below)\n\n```\nconda config --add channels conda-forge\nconda install pyamg\n```\n\n# Introduction\n\nPyAMG is a library of **Algebraic Multigrid (AMG)** solvers with a convenient Python interface.\n\n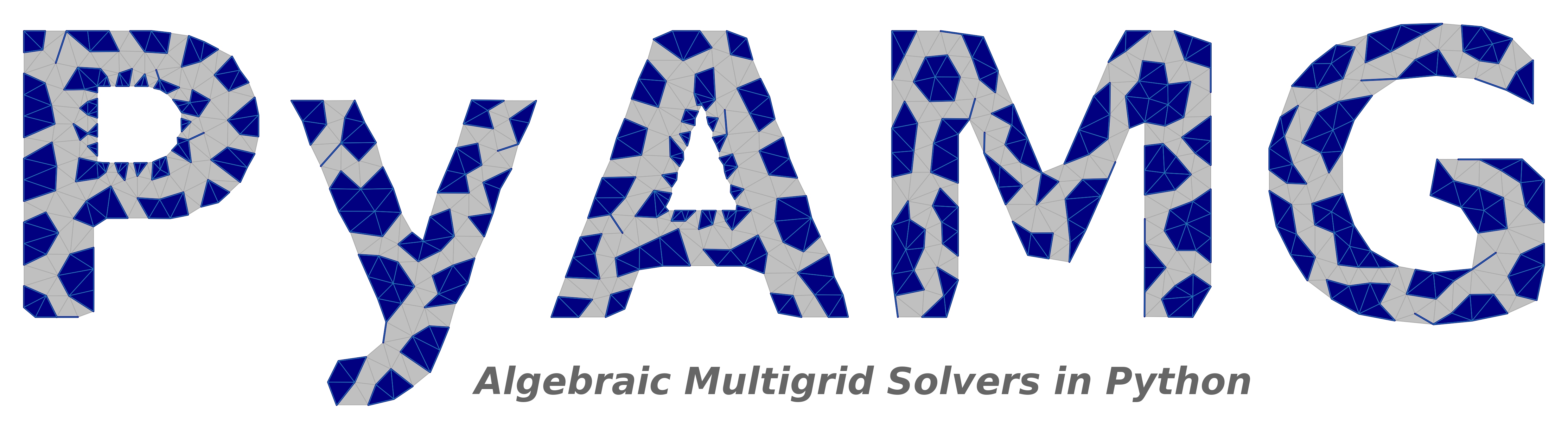\n\nPyAMG is currently developed and maintained by\n[Luke Olson](http://lukeo.cs.illinois.edu),\n[Jacob Schroder](https://www.unm.edu/~jbschroder), and\n[Ben Southworth](https://arxiv.org/a/southworth_b_1.html).\nThe organization of the project can be found in [`organization.md`](organization.md) and\nexamples of use can be found in [`pyamg-examples`](https://github.com/pyamg/pyamg-examples).\n\n**Acknowledgements:**\nPyAMG was created by\n[Nathan Bell](http://wnbell.com/), \n[Luke Olson](http://lukeo.cs.illinois.edu), and\n[Jacob Schroder](https://www.unm.edu/~jbschroder).\nPortions of the project were partially supported by the NSF under award DMS-0612448.\n\n# Citing\n\nIf you use PyAMG in your work, please consider using the following citation:\n\n<pre>\n@article{pyamg2023,\n author = {Nathan Bell and Luke N. Olson and Jacob Schroder and Ben Southworth},\n title = {{PyAMG}: Algebraic Multigrid Solvers in Python},\n journal = {Journal of Open Source Software},\n year = {2023},\n publisher = {The Open Journal},\n volume = {8},\n number = {87},\n pages = {5495},\n doi = {10.21105/joss.05495},\n url = {https://doi.org/10.21105/joss.05495},\n}\n</pre>\n\n# Getting Help\n\n- For documentation see [http://pyamg.readthedocs.io/en/latest/](http://pyamg.readthedocs.io/en/latest/).\n\n- Create an [issue](https://github.com/pyamg/pyamg/issues).\n\n- Look at the [Tutorial](https://github.com/pyamg/pyamg/wiki/Tutorial) or the [examples](https://github.com/pyamg/pyamg-examples) (for instance the [0_start_here](https://github.com/pyamg/pyamg-examples/blob/main/0_start_here/demo.py) example).\n\n- Run the unit tests (`pip install pytest`):\n - With PyAMG installed and from a non-source directory:\n ```python\n import pyamg\n pyamg.test()\n ```\n - From the PyAMG source directory and installed (e.g. with `pip install -e .`):\n ```python\n pytest .\n ```\n\n# What is AMG?\n\n AMG is a multilevel technique for solving large-scale linear systems with optimal or near-optimal efficiency. Unlike geometric multigrid, AMG requires little or no geometric information about the underlying problem and develops a sequence of coarser grids directly from the input matrix. This feature is especially important for problems discretized on unstructured meshes and irregular grids.\n\n# PyAMG Features\n\nPyAMG features implementations of:\n\n- **Ruge-Stuben (RS)** or *Classical AMG*\n- AMG based on **Smoothed Aggregation (SA)**\n\nand experimental support for:\n\n- **Adaptive Smoothed Aggregation (\u03b1SA)**\n- **Compatible Relaxation (CR)**\n\nThe predominant portion of PyAMG is written in Python with a smaller amount of supporting C++ code for performance critical operations.\n\n# Example Usage\n\nPyAMG is easy to use! The following code constructs a two-dimensional Poisson problem and solves the resulting linear system with Classical AMG.\n\n````python\nimport pyamg\nimport numpy as np\nA = pyamg.gallery.poisson((500,500), format='csr') # 2D Poisson problem on 500x500 grid\nml = pyamg.ruge_stuben_solver(A) # construct the multigrid hierarchy\nprint(ml) # print hierarchy information\nb = np.random.rand(A.shape[0]) # pick a random right hand side\nx = ml.solve(b, tol=1e-10) # solve Ax=b to a tolerance of 1e-10\nprint(\"residual: \", np.linalg.norm(b-A*x)) # compute norm of residual vector\n````\n\nProgram output:\n\n<pre>\nmultilevel_solver\nNumber of Levels: 9\nOperator Complexity: 2.199\nGrid Complexity: 1.667\nCoarse Solver: 'pinv2'\n level unknowns nonzeros\n 0 250000 1248000 [45.47%]\n 1 125000 1121002 [40.84%]\n 2 31252 280662 [10.23%]\n 3 7825 70657 [ 2.57%]\n 4 1937 17971 [ 0.65%]\n 5 483 4725 [ 0.17%]\n 6 124 1352 [ 0.05%]\n 7 29 293 [ 0.01%]\n 8 7 41 [ 0.00%]\n\nresidual: 1.24748994988e-08\n</pre>\n\n# Conda\n\nMore information can be found at [conda-forge/pyamg-feedstock](https://github.com/conda-forge/pyamg-feedstock).\n\n*Linux:*\n[](https://circleci.com/gh/conda-forge/pyamg-feedstock)\n\n*OSX:*\n[](https://travis-ci.org/conda-forge/pyamg-feedstock)\n\n*Windows:*\n[](https://ci.appveyor.com/project/conda-forge/pyamg-feedstock/branch/master)\n\n*Version:*\n[](https://anaconda.org/conda-forge/pyamg)\n\n*Downloads:*\n[](https://anaconda.org/conda-forge/pyamg)\n\nInstalling `pyamg` from the `conda-forge` channel can be achieved by adding `conda-forge` to your channels with:\n\n```\nconda config --add channels conda-forge\n```\n\nOnce the `conda-forge` channel has been enabled, `pyamg` can be installed with:\n\n```\nconda install pyamg\n```\n\nIt is possible to list all of the versions of `pyamg` available on your platform with:\n\n```\nconda search pyamg --channel conda-forge\n```\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "PyAMG: Algebraic Multigrid Solvers in Python",
"version": "5.2.1",
"project_urls": {
"Download": "https://github.com/pyamg/pyamg/releases",
"Homepage": "https://github.com/pyamg/pyamg"
},
"split_keywords": [
"algebraic multigrid",
" amg",
" sparse matrix",
" preconditioning"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "71d29e296528ea5ff0bfedf5fdf79851bac99fdd7b3286d355de68cc1c39ba43",
"md5": "d6048b8f86f52019ba0ae2edac30d24f",
"sha256": "486a2b58b53aa6dc606fae5b9f3aebb5dad635a2113cea4f300da16983749716"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp310-cp310-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "d6048b8f86f52019ba0ae2edac30d24f",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.9",
"size": 1716541,
"upload_time": "2024-06-27T04:45:28",
"upload_time_iso_8601": "2024-06-27T04:45:28.387995Z",
"url": "https://files.pythonhosted.org/packages/71/d2/9e296528ea5ff0bfedf5fdf79851bac99fdd7b3286d355de68cc1c39ba43/pyamg-5.2.1-cp310-cp310-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "3f188b6512e02cb620e121b75e9291c4ad8c8257cb49cd21b5771f57bd5dc513",
"md5": "ff2f2bb312bbf5b7f34ad825c45dcdf6",
"sha256": "5d45d02e21a63e92b29b108ab4ec111e699367116e8541318304c16f52ffa71a"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp310-cp310-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "ff2f2bb312bbf5b7f34ad825c45dcdf6",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.9",
"size": 1658872,
"upload_time": "2024-06-27T04:45:30",
"upload_time_iso_8601": "2024-06-27T04:45:30.736823Z",
"url": "https://files.pythonhosted.org/packages/3f/18/8b6512e02cb620e121b75e9291c4ad8c8257cb49cd21b5771f57bd5dc513/pyamg-5.2.1-cp310-cp310-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7f11d7f8dcc51f28b6b8d65e154b8f8c66217fd942718afe58088b4423501bdc",
"md5": "54f7d249668effecec0f59d929c712ad",
"sha256": "22ec55aeabb88724a01751150005d1209fb6edb6de9d8cb6e4129294774b8487"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "54f7d249668effecec0f59d929c712ad",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.9",
"size": 1954830,
"upload_time": "2024-06-27T04:45:32",
"upload_time_iso_8601": "2024-06-27T04:45:32.843806Z",
"url": "https://files.pythonhosted.org/packages/7f/11/d7f8dcc51f28b6b8d65e154b8f8c66217fd942718afe58088b4423501bdc/pyamg-5.2.1-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "34d2bd943fefd7f3a2bbe6f0a0ecf5b8433e85ac3fa53a33f5a6bcbd2c3e0639",
"md5": "1f4340a545c2827f6543f77de0a4f7e1",
"sha256": "585723f8e78048da5e6f6ae6336aa343b318154f02365a529867c741c49d529b"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp310-cp310-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "1f4340a545c2827f6543f77de0a4f7e1",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.9",
"size": 2766570,
"upload_time": "2024-06-27T04:45:34",
"upload_time_iso_8601": "2024-06-27T04:45:34.883423Z",
"url": "https://files.pythonhosted.org/packages/34/d2/bd943fefd7f3a2bbe6f0a0ecf5b8433e85ac3fa53a33f5a6bcbd2c3e0639/pyamg-5.2.1-cp310-cp310-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7e50b2dd5ed70d033e50c0f2f260754c2ca64e67d8aaa3d2fe04cc1e6028ae2b",
"md5": "5d2ce87baa18016c4ec77049eca9998b",
"sha256": "b14476eb193b83c4a2537be245b37919b4b5cc49cf4e85e232ae804b02a8f97c"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp310-cp310-win32.whl",
"has_sig": false,
"md5_digest": "5d2ce87baa18016c4ec77049eca9998b",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.9",
"size": 1449685,
"upload_time": "2024-06-27T04:45:36",
"upload_time_iso_8601": "2024-06-27T04:45:36.386272Z",
"url": "https://files.pythonhosted.org/packages/7e/50/b2dd5ed70d033e50c0f2f260754c2ca64e67d8aaa3d2fe04cc1e6028ae2b/pyamg-5.2.1-cp310-cp310-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2d32de2e9116e4cc313fb5dffd29c687fddd30ef24caec7b618a7e4d8804fc77",
"md5": "656f485bd74a342957309e99bff1dd2b",
"sha256": "f7cb7d4d43e13d67f5e7ad23ea20fa052cb310a186717e763a16b7c176248868"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp310-cp310-win_amd64.whl",
"has_sig": false,
"md5_digest": "656f485bd74a342957309e99bff1dd2b",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.9",
"size": 1579171,
"upload_time": "2024-06-27T04:45:38",
"upload_time_iso_8601": "2024-06-27T04:45:38.293308Z",
"url": "https://files.pythonhosted.org/packages/2d/32/de2e9116e4cc313fb5dffd29c687fddd30ef24caec7b618a7e4d8804fc77/pyamg-5.2.1-cp310-cp310-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ae0f5e725b26add355e52abcc9e2f065a85faaabe77e1b0926568743493230bb",
"md5": "28a33725a3efa6cfd9a26d2970bf649d",
"sha256": "60d02ce8e1190292cf43710dd45403ba0860255392830140da874492b755a498"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp311-cp311-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "28a33725a3efa6cfd9a26d2970bf649d",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.9",
"size": 1728275,
"upload_time": "2024-06-27T04:45:40",
"upload_time_iso_8601": "2024-06-27T04:45:40.345966Z",
"url": "https://files.pythonhosted.org/packages/ae/0f/5e725b26add355e52abcc9e2f065a85faaabe77e1b0926568743493230bb/pyamg-5.2.1-cp311-cp311-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a823fb5e9ae91de2a9d723f1ba6b484e7b4145d0cd15f4953b6f1ce89f39a602",
"md5": "ac2fd15d55c3b647f535ac48bad0e66c",
"sha256": "75d68fac5a7ddb3fb490efa66a5e47902532770bc357fbf5a5a7be613b8d493d"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp311-cp311-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "ac2fd15d55c3b647f535ac48bad0e66c",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.9",
"size": 1670008,
"upload_time": "2024-06-27T04:45:41",
"upload_time_iso_8601": "2024-06-27T04:45:41.576653Z",
"url": "https://files.pythonhosted.org/packages/a8/23/fb5e9ae91de2a9d723f1ba6b484e7b4145d0cd15f4953b6f1ce89f39a602/pyamg-5.2.1-cp311-cp311-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d3e86898b3b791f369605012e896ed903b6626f3bd1208c6a647d7219c070209",
"md5": "7a0d54c82a3df6439cc30bcdfd3f6d19",
"sha256": "679a5904eac3a4880288c8c0e6a29f110a2627ea15a443a4e9d5997c7dc5fab6"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "7a0d54c82a3df6439cc30bcdfd3f6d19",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.9",
"size": 1967667,
"upload_time": "2024-06-27T04:45:42",
"upload_time_iso_8601": "2024-06-27T04:45:42.987442Z",
"url": "https://files.pythonhosted.org/packages/d3/e8/6898b3b791f369605012e896ed903b6626f3bd1208c6a647d7219c070209/pyamg-5.2.1-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d8aedf3ef37e5ab6a21bb93e301eccbc9bb201bf247cfb2533c681a5070edf2f",
"md5": "bdc7afd44d119e18569b3ce2c2be9b64",
"sha256": "91a0f8d5f39debbe62ab191c9354fce6c547bd78955961f88cb7ab2ea7a14dc1"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp311-cp311-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "bdc7afd44d119e18569b3ce2c2be9b64",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.9",
"size": 2773740,
"upload_time": "2024-06-27T04:45:44",
"upload_time_iso_8601": "2024-06-27T04:45:44.459644Z",
"url": "https://files.pythonhosted.org/packages/d8/ae/df3ef37e5ab6a21bb93e301eccbc9bb201bf247cfb2533c681a5070edf2f/pyamg-5.2.1-cp311-cp311-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2af7c3c708cde09268eec830c29f28d569fcde2e164f28a39aa725f636396f38",
"md5": "6d32c721461fbaa10e20c3417b17a6ae",
"sha256": "0b2762b24ad61d900ac835120158e64ceea6e497a8a3edb69c3b04776ddde329"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp311-cp311-win32.whl",
"has_sig": false,
"md5_digest": "6d32c721461fbaa10e20c3417b17a6ae",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.9",
"size": 1458501,
"upload_time": "2024-06-27T04:45:46",
"upload_time_iso_8601": "2024-06-27T04:45:46.500324Z",
"url": "https://files.pythonhosted.org/packages/2a/f7/c3c708cde09268eec830c29f28d569fcde2e164f28a39aa725f636396f38/pyamg-5.2.1-cp311-cp311-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d2dee7638d7e9cef62e59480f2ac80ae63e31f7537367031dc9d571427a8d1c6",
"md5": "63c61a03950339bd843b98e8bf5e40e0",
"sha256": "487c41ed3c2987b19771854e84415268033a8c3dba681ecac996ddbf1ce4c3be"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp311-cp311-win_amd64.whl",
"has_sig": false,
"md5_digest": "63c61a03950339bd843b98e8bf5e40e0",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.9",
"size": 1588292,
"upload_time": "2024-06-27T04:45:48",
"upload_time_iso_8601": "2024-06-27T04:45:48.462295Z",
"url": "https://files.pythonhosted.org/packages/d2/de/e7638d7e9cef62e59480f2ac80ae63e31f7537367031dc9d571427a8d1c6/pyamg-5.2.1-cp311-cp311-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d65dc603b5e5fe0b871095691fcc6453e6f666b4006475f94220f01fba376178",
"md5": "47ecabbb62b6f1a964089a340c36d173",
"sha256": "30ae9f374c03897cb257b8eabbf8ceb32007e6df1c0a7ab2a84eb1ed0550087d"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp312-cp312-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "47ecabbb62b6f1a964089a340c36d173",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.9",
"size": 1721956,
"upload_time": "2024-06-27T04:45:49",
"upload_time_iso_8601": "2024-06-27T04:45:49.762056Z",
"url": "https://files.pythonhosted.org/packages/d6/5d/c603b5e5fe0b871095691fcc6453e6f666b4006475f94220f01fba376178/pyamg-5.2.1-cp312-cp312-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0a7c30faaa543a3f5da647deb604f0a7c6f70e62ba157b1b0a7ca977a89cc814",
"md5": "e9a87e07b5911c4db3222b3b41ad46c8",
"sha256": "5f1645e50ed8d93d73c7546ca6e77c856dc3ccddf93bf9ded3f97654e600ca1b"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp312-cp312-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "e9a87e07b5911c4db3222b3b41ad46c8",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.9",
"size": 1663104,
"upload_time": "2024-06-27T04:45:51",
"upload_time_iso_8601": "2024-06-27T04:45:51.816292Z",
"url": "https://files.pythonhosted.org/packages/0a/7c/30faaa543a3f5da647deb604f0a7c6f70e62ba157b1b0a7ca977a89cc814/pyamg-5.2.1-cp312-cp312-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8cf3d2b4f5c265188bf6f540ecd1d5f208bac2931b332613c116cc33da4e4aa8",
"md5": "ad263bc901aa4acc857dfdf56dc4ca18",
"sha256": "1894898370f818be7999ed6872391bc7de4f8a2a95027754b67bac638f23a480"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "ad263bc901aa4acc857dfdf56dc4ca18",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.9",
"size": 1961942,
"upload_time": "2024-06-27T04:45:53",
"upload_time_iso_8601": "2024-06-27T04:45:53.356877Z",
"url": "https://files.pythonhosted.org/packages/8c/f3/d2b4f5c265188bf6f540ecd1d5f208bac2931b332613c116cc33da4e4aa8/pyamg-5.2.1-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "e4fc58c3efb48e8bc2cfdbb50c501ac15b67260309c4df5a80905e6ccca7397b",
"md5": "73dac7d1b212095273c0e54ccf9bd446",
"sha256": "1eabc5bd1aa21f0911021d17fdbb03d93216bfefaac0a9af357fed1510dd4f65"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp312-cp312-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "73dac7d1b212095273c0e54ccf9bd446",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.9",
"size": 2770007,
"upload_time": "2024-06-27T04:45:55",
"upload_time_iso_8601": "2024-06-27T04:45:55.296395Z",
"url": "https://files.pythonhosted.org/packages/e4/fc/58c3efb48e8bc2cfdbb50c501ac15b67260309c4df5a80905e6ccca7397b/pyamg-5.2.1-cp312-cp312-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "e487b4cc37648e728d4bb92a9956f4d81275cab39000f4fead94322e43e4dd9e",
"md5": "29e7c8a2f22ac484e1199b8aa5c1856e",
"sha256": "5ff6202143f0c5fa093b4f99b9de4e6e5195fb7fecaf001a1f6a5a21b1fe329d"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp312-cp312-win32.whl",
"has_sig": false,
"md5_digest": "29e7c8a2f22ac484e1199b8aa5c1856e",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.9",
"size": 1459728,
"upload_time": "2024-06-27T04:45:57",
"upload_time_iso_8601": "2024-06-27T04:45:57.244980Z",
"url": "https://files.pythonhosted.org/packages/e4/87/b4cc37648e728d4bb92a9956f4d81275cab39000f4fead94322e43e4dd9e/pyamg-5.2.1-cp312-cp312-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9ef3cb009732b4f467a63f53fb6be99206568c521d1b7c70fa9809457d7138b3",
"md5": "51d206a2fe9026799a849150a881832e",
"sha256": "dac4b0656188cf85e803355aa9065fd2d6d2237fc29bb5e55995331f75e0f616"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp312-cp312-win_amd64.whl",
"has_sig": false,
"md5_digest": "51d206a2fe9026799a849150a881832e",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.9",
"size": 1592216,
"upload_time": "2024-06-27T04:45:58",
"upload_time_iso_8601": "2024-06-27T04:45:58.514848Z",
"url": "https://files.pythonhosted.org/packages/9e/f3/cb009732b4f467a63f53fb6be99206568c521d1b7c70fa9809457d7138b3/pyamg-5.2.1-cp312-cp312-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "dbb254cf2d888d94a6d60ce2b2fa670f68429e442b625ae6f23f70e58082ca12",
"md5": "0c621a3911fb5e4f67083b65174667e0",
"sha256": "4159c8d13ea0c35d881ef76e14b94fa248e99d60bd9140a7762fcca972f34de7"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp39-cp39-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "0c621a3911fb5e4f67083b65174667e0",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.9",
"size": 1717634,
"upload_time": "2024-06-27T04:45:59",
"upload_time_iso_8601": "2024-06-27T04:45:59.988244Z",
"url": "https://files.pythonhosted.org/packages/db/b2/54cf2d888d94a6d60ce2b2fa670f68429e442b625ae6f23f70e58082ca12/pyamg-5.2.1-cp39-cp39-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1fbb50c6651ba042d2c196afbc1c5911b19293713794010b3b4ab8f85c3f7f77",
"md5": "2bc4163aa7641323d00760d99a4a1031",
"sha256": "6da02369515d56647fadab8390933d510969ff36b396bf5d11eb6738484ce014"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp39-cp39-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "2bc4163aa7641323d00760d99a4a1031",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.9",
"size": 1659939,
"upload_time": "2024-06-27T04:46:02",
"upload_time_iso_8601": "2024-06-27T04:46:02.066801Z",
"url": "https://files.pythonhosted.org/packages/1f/bb/50c6651ba042d2c196afbc1c5911b19293713794010b3b4ab8f85c3f7f77/pyamg-5.2.1-cp39-cp39-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "deb6411b3de91fb23aebee8082af5c970f8345e06d8357ce1c33464837130770",
"md5": "6537f804570cde37d6a1f760038be22b",
"sha256": "40acf38eb733dd33f054f2b9a6aa3cee1786b7a5420b602787f2d38eca8051ac"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "6537f804570cde37d6a1f760038be22b",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.9",
"size": 1956522,
"upload_time": "2024-06-27T04:46:03",
"upload_time_iso_8601": "2024-06-27T04:46:03.453510Z",
"url": "https://files.pythonhosted.org/packages/de/b6/411b3de91fb23aebee8082af5c970f8345e06d8357ce1c33464837130770/pyamg-5.2.1-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "545b99aaae251b54d7fc5338cac33833e7f75ad19f1856af80eb1524f6550c1e",
"md5": "f088ed6bb58a6681826e95d18ffff223",
"sha256": "f297ecd26aa9130ca57c74fe52e40a7590e0f0aef50616b39ce7ab02ebc1a8a9"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp39-cp39-musllinux_1_2_x86_64.whl",
"has_sig": false,
"md5_digest": "f088ed6bb58a6681826e95d18ffff223",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.9",
"size": 2765286,
"upload_time": "2024-06-27T04:46:04",
"upload_time_iso_8601": "2024-06-27T04:46:04.928107Z",
"url": "https://files.pythonhosted.org/packages/54/5b/99aaae251b54d7fc5338cac33833e7f75ad19f1856af80eb1524f6550c1e/pyamg-5.2.1-cp39-cp39-musllinux_1_2_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "c90ba95880eddb43003dd7ce4e6080e0946b26eae27d4a04e6093cdab43264b6",
"md5": "77bd1e948795518e278a7823ed0898c9",
"sha256": "4b7ee45d5b3f96897146b55d2a1829d3f7c692cafb2bc99396e14e2bf1c645b3"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp39-cp39-win32.whl",
"has_sig": false,
"md5_digest": "77bd1e948795518e278a7823ed0898c9",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.9",
"size": 1451071,
"upload_time": "2024-06-27T04:46:06",
"upload_time_iso_8601": "2024-06-27T04:46:06.485293Z",
"url": "https://files.pythonhosted.org/packages/c9/0b/a95880eddb43003dd7ce4e6080e0946b26eae27d4a04e6093cdab43264b6/pyamg-5.2.1-cp39-cp39-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "f6db8fdfb4b251e5a2c0219f0339a9e749a458fd4cdbfe47d8a62848d17e0e2c",
"md5": "db69347c853d60ee190e4f86762d5392",
"sha256": "0bc04266f177bb92f4468af3d30b06b2925de54107e15b3cfc118a06c96a88cf"
},
"downloads": -1,
"filename": "pyamg-5.2.1-cp39-cp39-win_amd64.whl",
"has_sig": false,
"md5_digest": "db69347c853d60ee190e4f86762d5392",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.9",
"size": 1569058,
"upload_time": "2024-06-27T04:46:07",
"upload_time_iso_8601": "2024-06-27T04:46:07.911542Z",
"url": "https://files.pythonhosted.org/packages/f6/db/8fdfb4b251e5a2c0219f0339a9e749a458fd4cdbfe47d8a62848d17e0e2c/pyamg-5.2.1-cp39-cp39-win_amd64.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-27 04:45:28",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "pyamg",
"github_project": "pyamg",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "numpy",
"specs": []
},
{
"name": "scipy",
"specs": [
[
">=",
"1.11.0"
]
]
}
],
"lcname": "pyamg"
}