# pyGenomeViz



[](https://pypi.python.org/pypi/pygenomeviz)
[](https://anaconda.org/conda-forge/pygenomeviz)
[](https://github.com/moshi4/pyGenomeViz/actions/workflows/ci.yml)
> [!NOTE]
> A major version upgrade, pyGenomeViz **v1.0.0**, was released on 2024/05.
> Backward incompatible changes have been made between v1.0.0 and v0.X.X to make for a more sophisticated API/CLI design.
> Therefore, v0.X.X users should pin the version to v0.4.4 or update existing code for v1.0.0.
> Previous v0.4.4 documentation is available [here](https://moshi4.github.io/docs/pygenomeviz/v0.4.4/).
## Table of contents
- [Overview](#overview)
- [Installation](#installation)
- [API Examples](#api-examples)
- [CLI Examples](#cli-examples)
- [GUI (Web Application)](#gui-web-application)
- [HTML Viewer](#html-viewer)
- [Inspiration](#inspiration)
- [Circular Genome Visualization](#circular-genome-visualization)
- [Star History](#star-history)
## Overview
pyGenomeViz is a genome visualization python package for comparative genomics implemented based on matplotlib.
This package is developed for the purpose of easily and beautifully plotting genomic
features and sequence similarity comparison links between multiple genomes.
It supports genome visualization of Genbank/GFF format file and can be saved figure in various formats (JPG/PNG/SVG/PDF/HTML).
User can use pyGenomeViz for interactive genome visualization figure plotting on jupyter notebook,
or automatic genome visualization figure plotting in genome analysis scripts/workflow.
For more information, please see full documentation [here](https://moshi4.github.io/pyGenomeViz/).
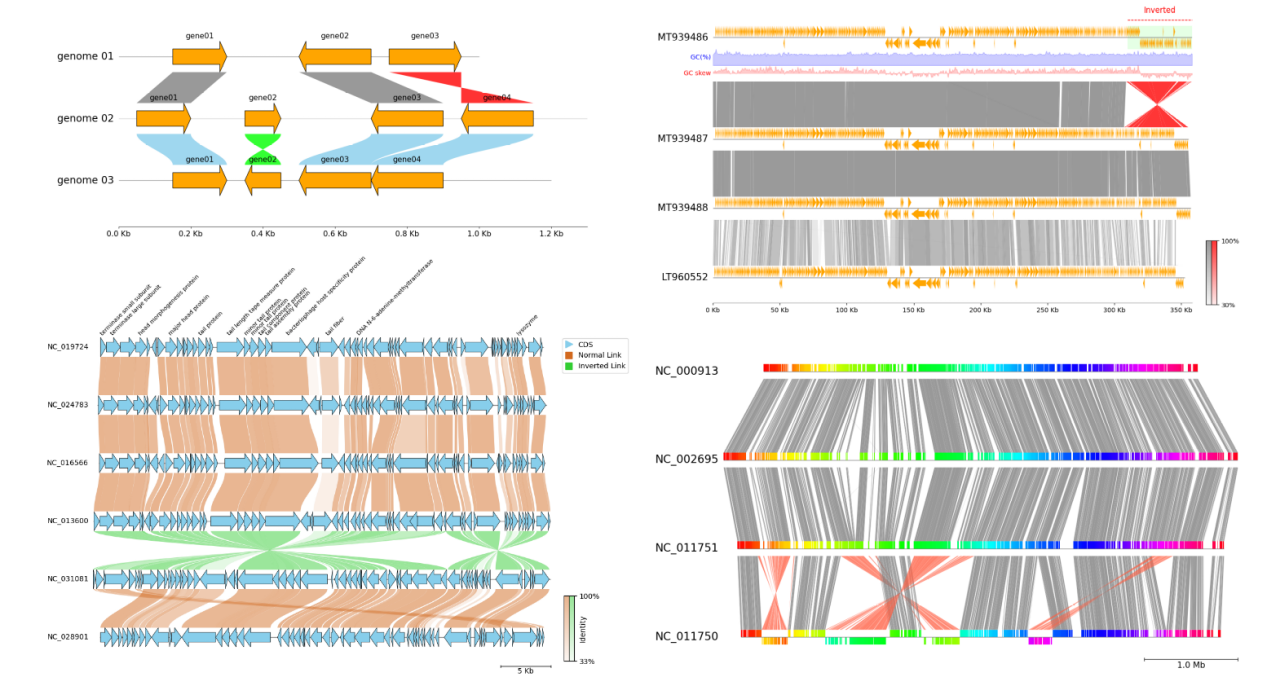
**Fig.1 pyGenomeViz example plot gallery**
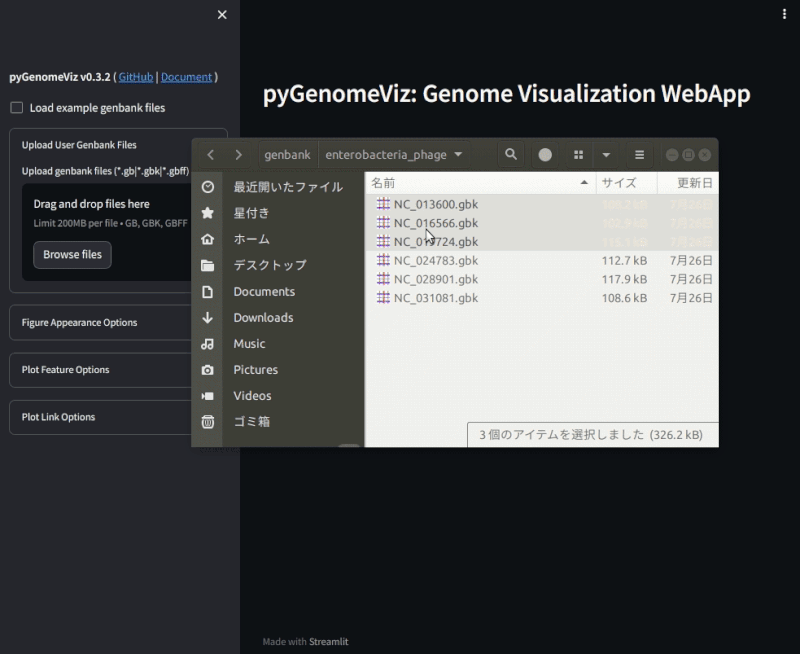
**Fig.2 pyGenomeViz web application example ([Demo Page](https://pygenomeviz.streamlit.app))**
## Installation
`Python 3.9 or later` is required for installation.
**Install PyPI package:**
pip install pygenomeviz
**Install conda-forge package:**
conda install -c conda-forge pygenomeviz
**Use Docker ([Image Registry](https://github.com/moshi4/pyGenomeViz/pkgs/container/pygenomeviz)):**
docker run -it --rm -p 8501:8501 ghcr.io/moshi4/pygenomeviz:latest pgv-gui -h
## API Examples
Jupyter notebooks containing code examples below is available [here](https://github.com/moshi4/pyGenomeViz/blob/main/notebooks/example.ipynb).
### Features
```python
from pygenomeviz import GenomeViz
gv = GenomeViz()
gv.set_scale_xticks(ymargin=0.5)
track = gv.add_feature_track("tutorial", 1000)
track.add_sublabel()
track.add_feature(50, 200, 1)
track.add_feature(250, 460, -1, fc="blue")
track.add_feature(500, 710, 1, fc="lime")
track.add_feature(750, 960, 1, fc="magenta", lw=1.0)
gv.savefig("features.png")
```

### Styled Features
```python
from pygenomeviz import GenomeViz
gv = GenomeViz()
gv.set_scale_bar(ymargin=0.5)
track = gv.add_feature_track("tutorial", (1000, 2000))
track.add_sublabel()
track.add_feature(1050, 1150, 1, label="arrow")
track.add_feature(1200, 1300, -1, plotstyle="bigarrow", label="bigarrow", fc="red", lw=1)
track.add_feature(1330, 1400, 1, plotstyle="bigbox", label="bigbox", fc="blue", text_kws=dict(rotation=0, hpos="center"))
track.add_feature(1420, 1500, 1, plotstyle="box", label="box", fc="limegreen", text_kws=dict(size=10, color="blue"))
track.add_feature(1550, 1600, 1, plotstyle="bigrbox", label="bigrbox", fc="magenta", ec="blue", lw=1, text_kws=dict(rotation=0, vpos="bottom", hpos="center"))
track.add_feature(1650, 1750, -1, plotstyle="rbox", label="rbox", fc="grey", text_kws=dict(rotation=-45, vpos="bottom"))
track.add_feature(1780, 1880, 1, fc="lime", hatch="o", arrow_shaft_ratio=0.2, label="arrow shaft\n0.2", text_kws=dict(rotation=0, hpos="center"))
track.add_feature(1890, 1990, 1, fc="lime", hatch="/", arrow_shaft_ratio=1.0, label="arrow shaft\n1.0", text_kws=dict(rotation=0, hpos="center"))
gv.savefig("styled_features.png")
```
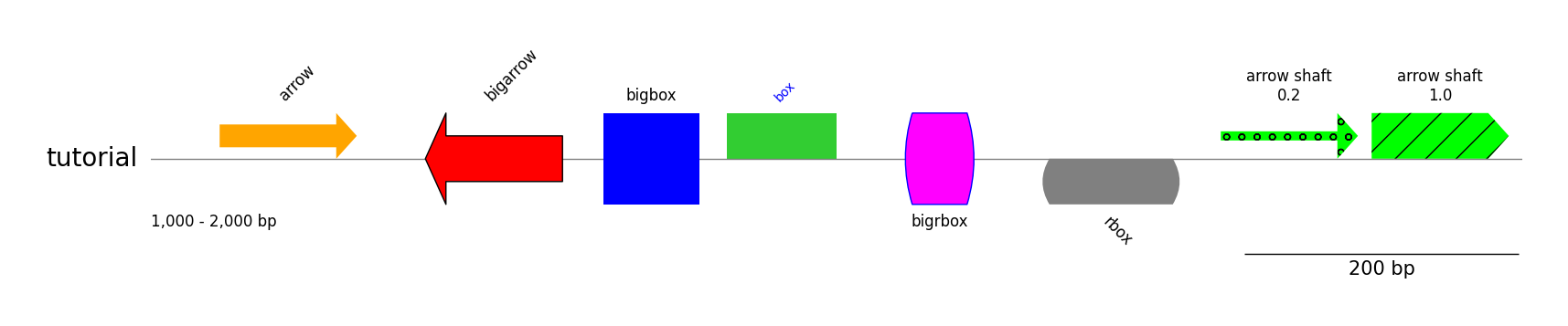
### Tracks & Links
```python
from pygenomeviz import GenomeViz
genome_list = [
dict(name="genome 01", size=1000, features=((150, 300, 1), (500, 700, -1), (750, 950, 1))),
dict(name="genome 02", size=1300, features=((50, 200, 1), (350, 450, 1), (700, 900, -1), (950, 1150, -1))),
dict(name="genome 03", size=1200, features=((150, 300, 1), (350, 450, -1), (500, 700, -1), (700, 900, -1))),
]
gv = GenomeViz(track_align_type="center")
gv.set_scale_bar()
for genome in genome_list:
name, size, features = genome["name"], genome["size"], genome["features"]
track = gv.add_feature_track(name, size)
track.add_sublabel()
for idx, feature in enumerate(features, 1):
start, end, strand = feature
track.add_feature(start, end, strand, plotstyle="bigarrow", lw=1, label=f"gene{idx:02d}", text_kws=dict(rotation=0, vpos="top", hpos="center"))
# Add links between "genome 01" and "genome 02"
gv.add_link(("genome 01", 150, 300), ("genome 02", 50, 200))
gv.add_link(("genome 01", 700, 500), ("genome 02", 900, 700))
gv.add_link(("genome 01", 750, 950), ("genome 02", 1150, 950))
# Add links between "genome 02" and "genome 03"
gv.add_link(("genome 02", 50, 200), ("genome 03", 150, 300), color="skyblue", inverted_color="lime", curve=True)
gv.add_link(("genome 02", 350, 450), ("genome 03", 450, 350), color="skyblue", inverted_color="lime", curve=True)
gv.add_link(("genome 02", 900, 700), ("genome 03", 700, 500), color="skyblue", inverted_color="lime", curve=True)
gv.add_link(("genome 03", 900, 700), ("genome 02", 1150, 950), color="skyblue", inverted_color="lime", curve=True)
gv.savefig("tracks_and_links.png")
```
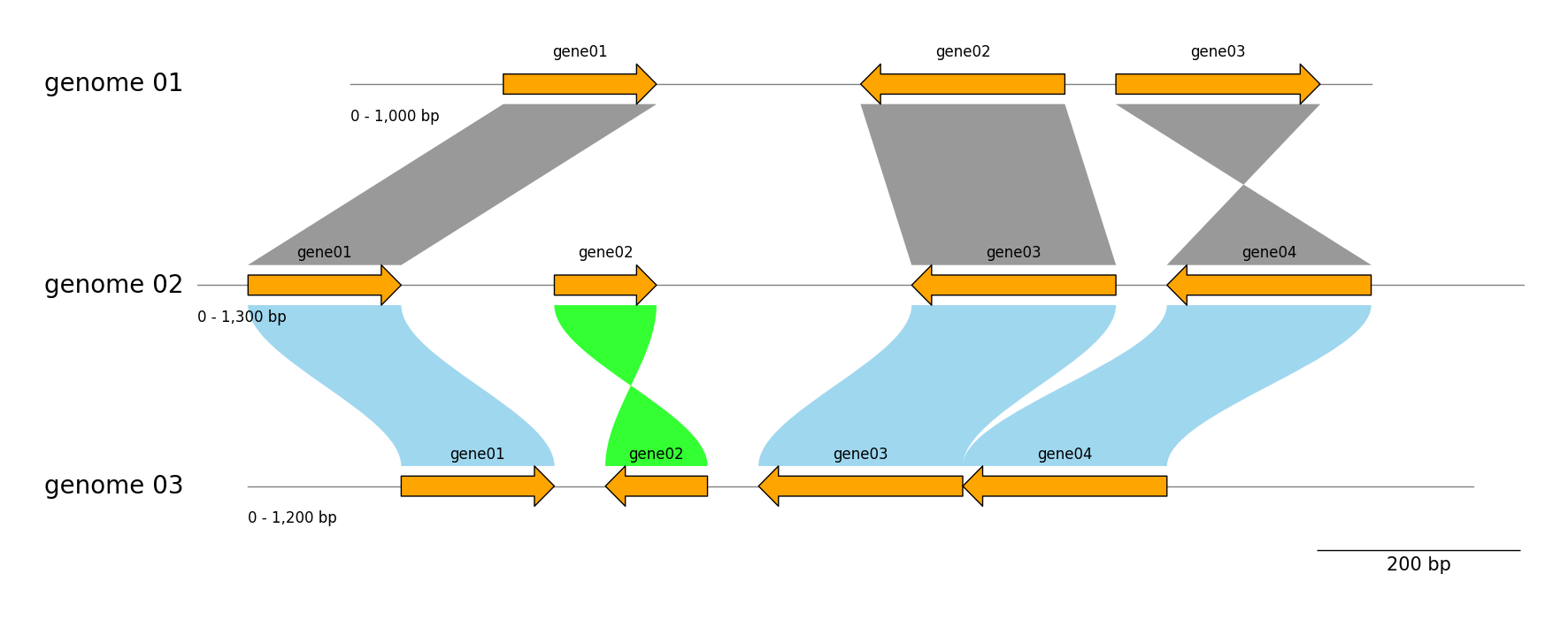
### Exon Features
```python
from pygenomeviz import GenomeViz
exon_regions1 = [(0, 210), (300, 480), (590, 800), (850, 1000), (1030, 1300)]
exon_regions2 = [(1500, 1710), (2000, 2480), (2590, 2800)]
exon_regions3 = [(3000, 3300), (3400, 3690), (3800, 4100), (4200, 4620)]
gv = GenomeViz()
track = gv.add_feature_track("Exon Features", 5000)
track.add_exon_feature(exon_regions1, strand=1, plotstyle="box", label="box", text_kws=dict(rotation=0, hpos="center"))
track.add_exon_feature(exon_regions2, strand=-1, plotstyle="arrow", label="arrow", text_kws=dict(rotation=0, vpos="bottom", hpos="center"), patch_kws=dict(fc="darkgrey"), intron_patch_kws=dict(ec="red"))
track.add_exon_feature(exon_regions3, strand=1, plotstyle="bigarrow", label="bigarrow", text_kws=dict(rotation=0, hpos="center"), patch_kws=dict(fc="lime", lw=1))
gv.savefig("exon_features.png")
```

### Genbank Features
```python
from pygenomeviz import GenomeViz
from pygenomeviz.parser import Genbank
from pygenomeviz.utils import load_example_genbank_dataset
gbk_files = load_example_genbank_dataset("yersinia_phage")
gbk = Genbank(gbk_files[0])
gv = GenomeViz()
gv.set_scale_bar(ymargin=0.5)
track = gv.add_feature_track(gbk.name, gbk.genome_length)
track.add_sublabel()
features = gbk.extract_features()
track.add_features(features)
gv.savefig("genbank_features.png")
```

### GFF Features
```python
from pygenomeviz import GenomeViz
from pygenomeviz.parser import Gff
from pygenomeviz.utils import load_example_gff_file
gff_file = load_example_gff_file("escherichia_coli.gff.gz")
gff = Gff(gff_file)
gv = GenomeViz()
gv.set_scale_bar(ymargin=0.5)
target_ranges = ((220000, 230000), (300000, 310000))
track = gv.add_feature_track(name=gff.name, segments=target_ranges)
track.set_segment_sep(symbol="//")
for segment in track.segments:
segment.add_sublabel()
# Plot CDS features
cds_features = gff.extract_features(feature_type="CDS", target_range=segment.range)
segment.add_features(cds_features, label_type="gene", fc="skyblue", lw=1.0)
# Plot rRNA features
rrna_features = gff.extract_features(feature_type="rRNA", target_range=segment.range)
segment.add_features(rrna_features, label_type="product", hatch="//", fc="lime", lw=1.0)
gv.savefig("gff_features.png")
```
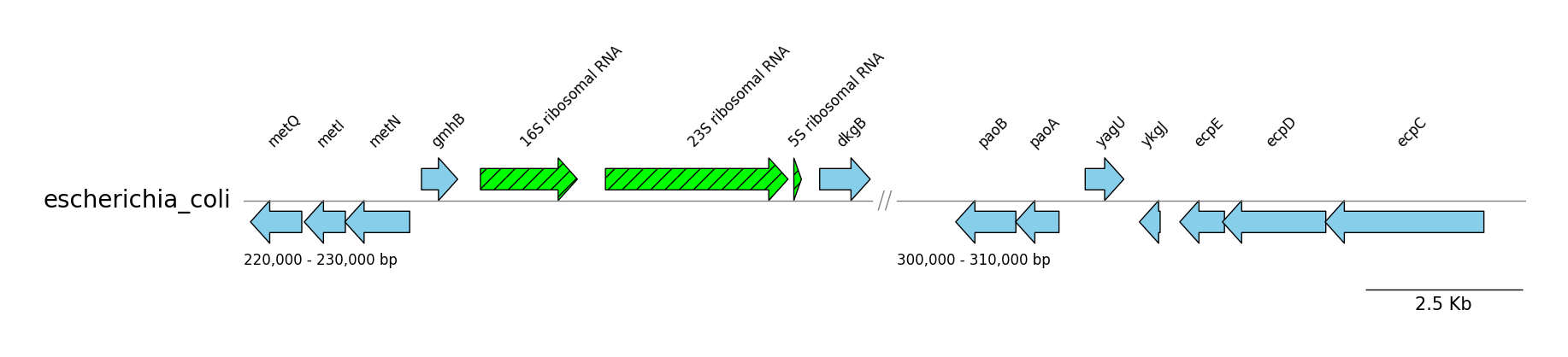
### GFF Contigs
```python
from pygenomeviz import GenomeViz
from pygenomeviz.parser import Gff
from pygenomeviz.utils import load_example_gff_file, is_pseudo_feature
gff_file = load_example_gff_file("mycoplasma_mycoides.gff")
gff = Gff(gff_file)
gv = GenomeViz(fig_track_height=0.5, feature_track_ratio=0.5)
gv.set_scale_xticks(labelsize=10)
# Plot CDS, rRNA features for each contig to tracks
for seqid, size in gff.get_seqid2size().items():
track = gv.add_feature_track(seqid, size, labelsize=15)
track.add_sublabel(size=10, color="grey")
cds_features = gff.get_seqid2features(feature_type="CDS")[seqid]
# CDS: blue, CDS(pseudo): grey
for cds_feature in cds_features:
color = "grey" if is_pseudo_feature(cds_feature) else "blue"
track.add_features(cds_feature, color=color)
# rRNA: lime
rrna_features = gff.get_seqid2features(feature_type="rRNA")[seqid]
track.add_features(rrna_features, color="lime")
gv.savefig("gff_contigs.png")
```
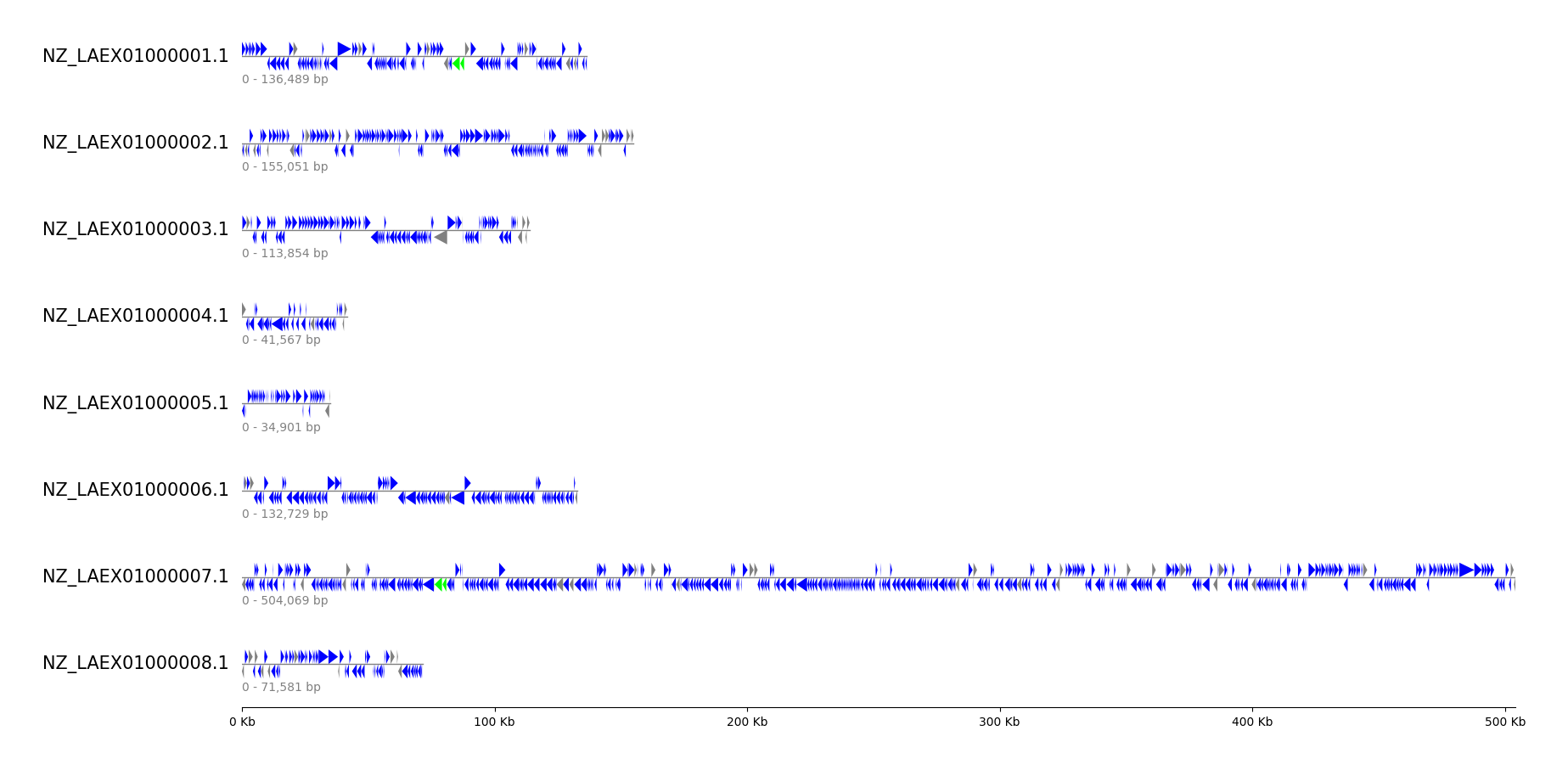
### Genbank Comparison by BLAST
```python
from pygenomeviz import GenomeViz
from pygenomeviz.parser import Genbank
from pygenomeviz.utils import load_example_genbank_dataset
from pygenomeviz.align import Blast, AlignCoord
gbk_files = load_example_genbank_dataset("yersinia_phage")
gbk_list = list(map(Genbank, gbk_files))
gv = GenomeViz(track_align_type="center")
gv.set_scale_bar()
# Plot CDS features
for gbk in gbk_list:
track = gv.add_feature_track(gbk.name, gbk.get_seqid2size(), align_label=False)
for seqid, features in gbk.get_seqid2features("CDS").items():
segment = track.get_segment(seqid)
segment.add_features(features, plotstyle="bigarrow", fc="limegreen", lw=0.5)
# Run BLAST alignment & filter by user-defined threshold
align_coords = Blast(gbk_list, seqtype="protein").run()
align_coords = AlignCoord.filter(align_coords, length_thr=100, identity_thr=30)
# Plot BLAST alignment links
if len(align_coords) > 0:
min_ident = int(min([ac.identity for ac in align_coords if ac.identity]))
color, inverted_color = "grey", "red"
for ac in align_coords:
gv.add_link(ac.query_link, ac.ref_link, color=color, inverted_color=inverted_color, v=ac.identity, vmin=min_ident)
gv.set_colorbar([color, inverted_color], vmin=min_ident)
gv.savefig("genbank_comparison_by_blast.png")
```
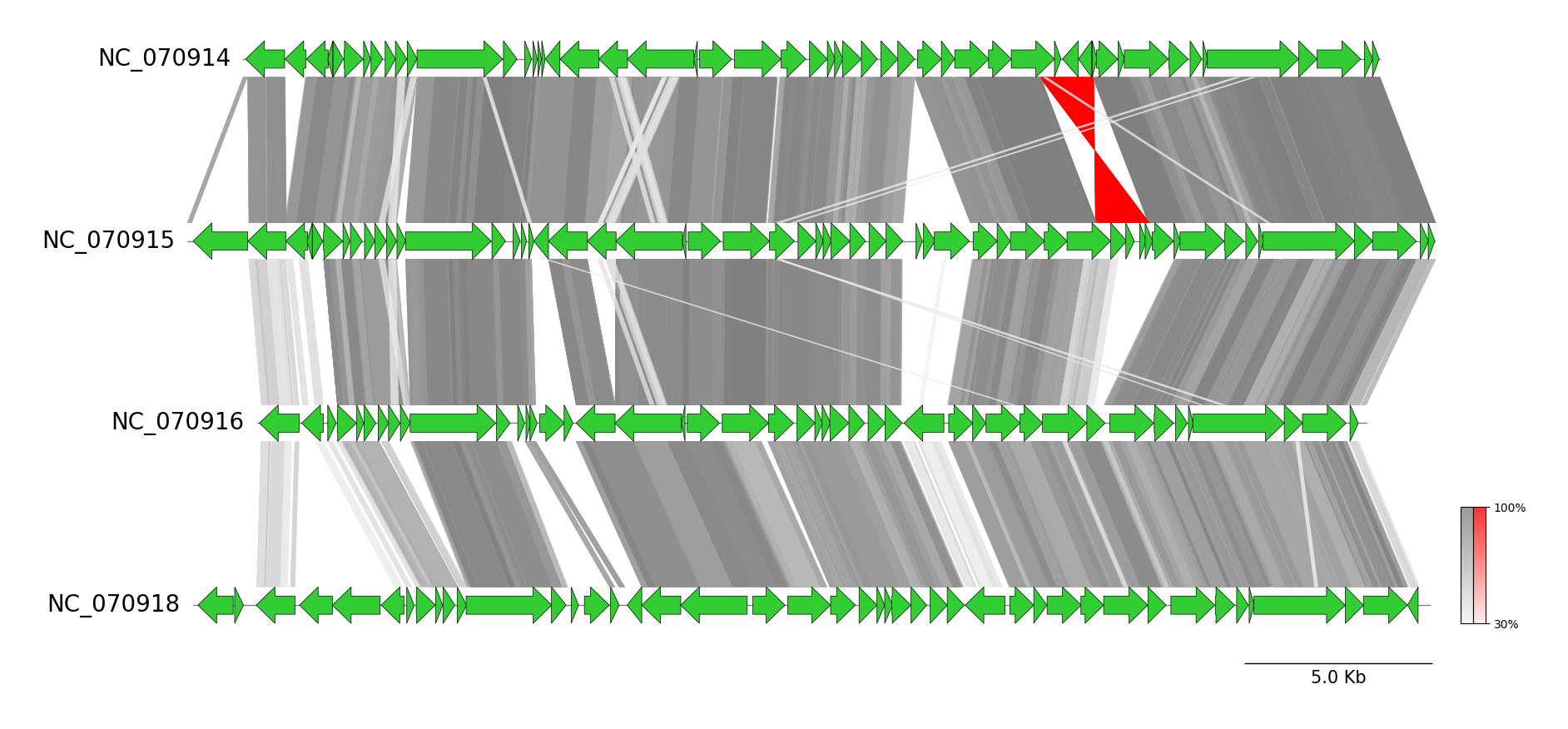
## CLI Examples
pyGenomeViz provides CLI workflows for genome alignment result visualization of
Genbank genomes using `BLAST` / `MUMmer` / `MMseqs` / `progressiveMauve`, respectively.
### BLAST CLI Workflow
See [pgv-blast document](https://moshi4.github.io/pyGenomeViz/cli-docs/pgv-blast/) for details.
```shell
# Download example dataset
pgv-download yersinia_phage
# Run BLAST CLI workflow
pgv-blast NC_070914.gbk NC_070915.gbk NC_070916.gbk NC_070918.gbk \
-o pgv-blast_example --seqtype protein --show_scale_bar --curve \
--feature_linewidth 0.3 --length_thr 100 --identity_thr 30
```
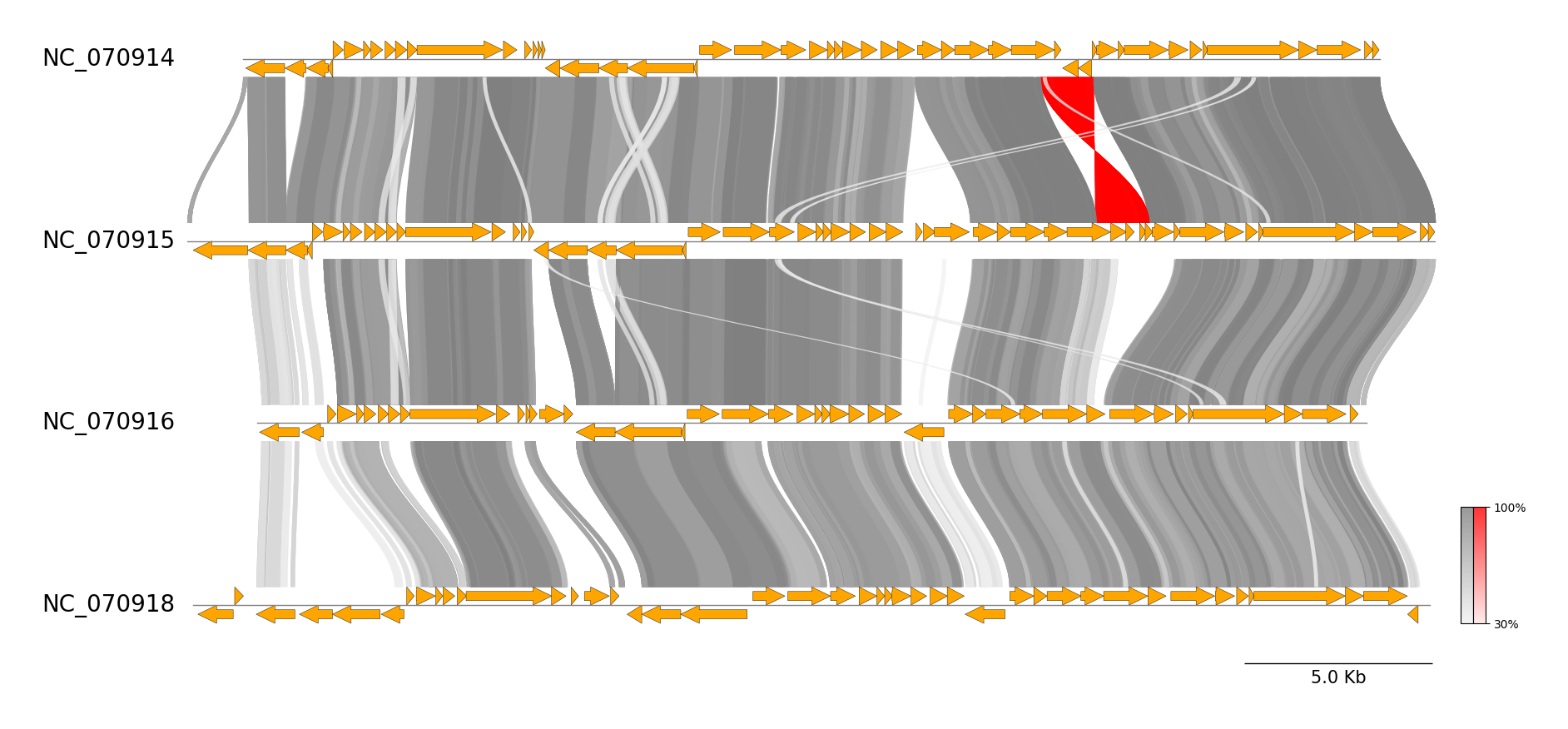
### MUMmer CLI Workflow
See [pgv-mummer document](https://moshi4.github.io/pyGenomeViz/cli-docs/pgv-mummer/) for details.
```shell
# Download example dataset
pgv-download mycoplasma_mycoides
# Run MUMmer CLI workflow
pgv-mummer GCF_000023685.1.gbff GCF_000800785.1.gbff GCF_000959055.1.gbff GCF_000959065.1.gbff \
-o pgv-mummer_example --show_scale_bar --curve \
--feature_type2color CDS:blue rRNA:lime tRNA:magenta
```
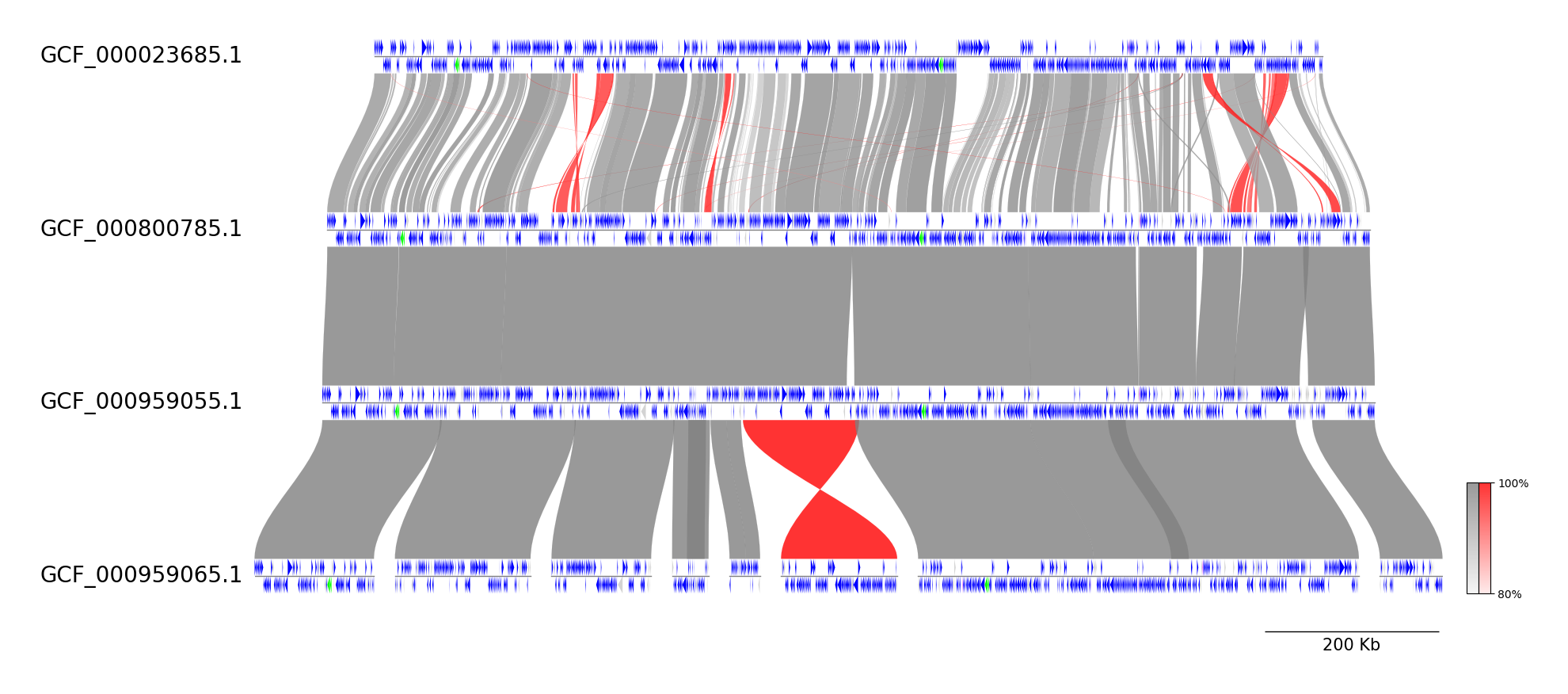
### MMseqs CLI Workflow
See [pgv-mmseqs document](https://moshi4.github.io/pyGenomeViz/cli-docs/pgv-mmseqs/) for details.
```shell
# Download example dataset
pgv-download enterobacteria_phage
# Run MMseqs CLI workflow
pgv-mmseqs NC_013600.gbk NC_016566.gbk NC_019724.gbk NC_024783.gbk NC_028901.gbk NC_031081.gbk \
-o pgv-mmseqs_example --show_scale_bar --curve --feature_linewidth 0.3 \
--feature_type2color CDS:skyblue --normal_link_color chocolate --inverted_link_color limegreen
```
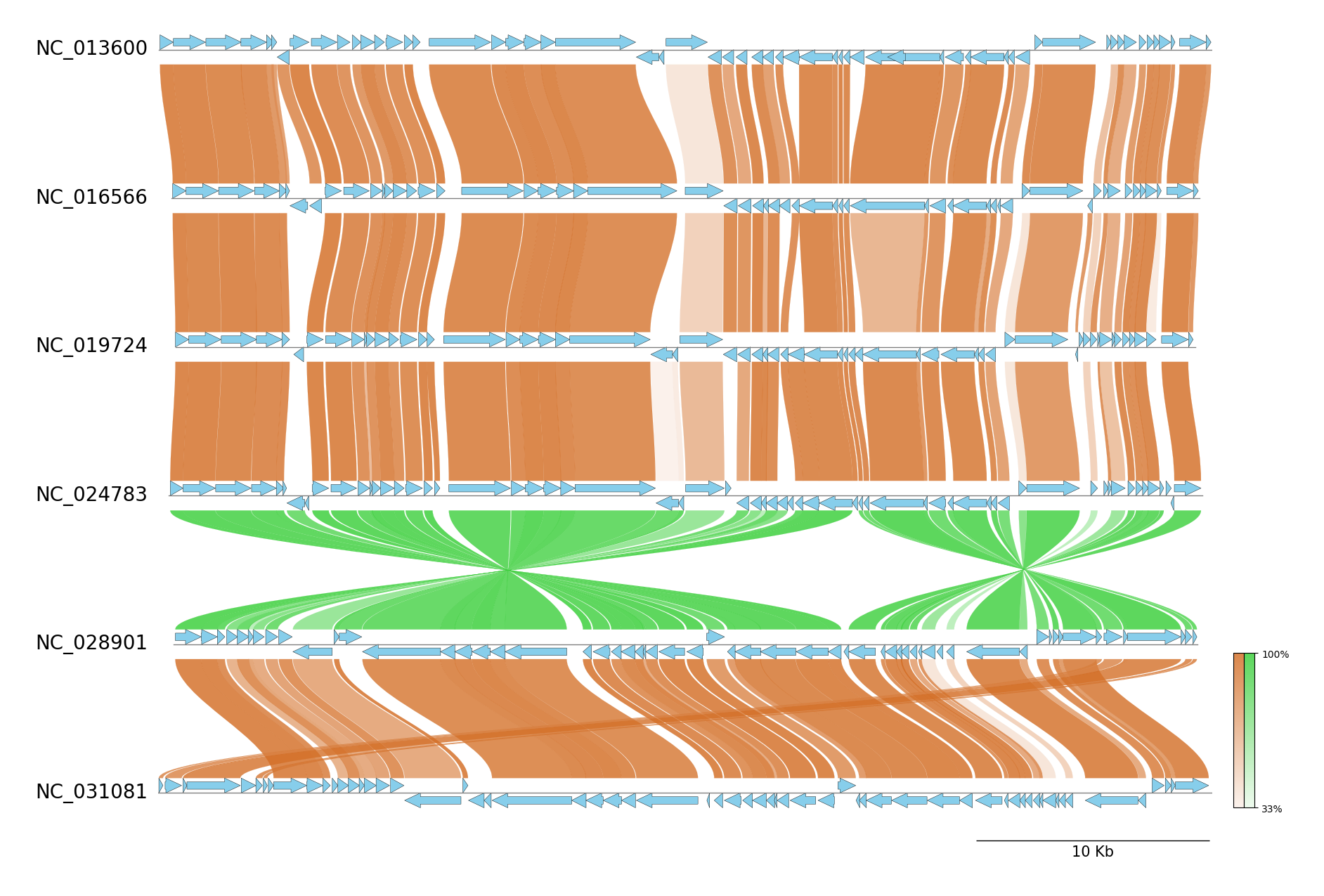
### progressiveMauve CLI Workflow
See [pgv-pmauve document](https://moshi4.github.io/pyGenomeViz/cli-docs/pgv-pmauve/) for details.
```shell
# Download example dataset
pgv-download escherichia_coli
# Run progressiveMauve CLI workflow
pgv-pmauve NC_000913.gbk.gz NC_002695.gbk.gz NC_011751.gbk.gz NC_011750.gbk.gz \
-o pgv-pmauve_example --show_scale_bar
```
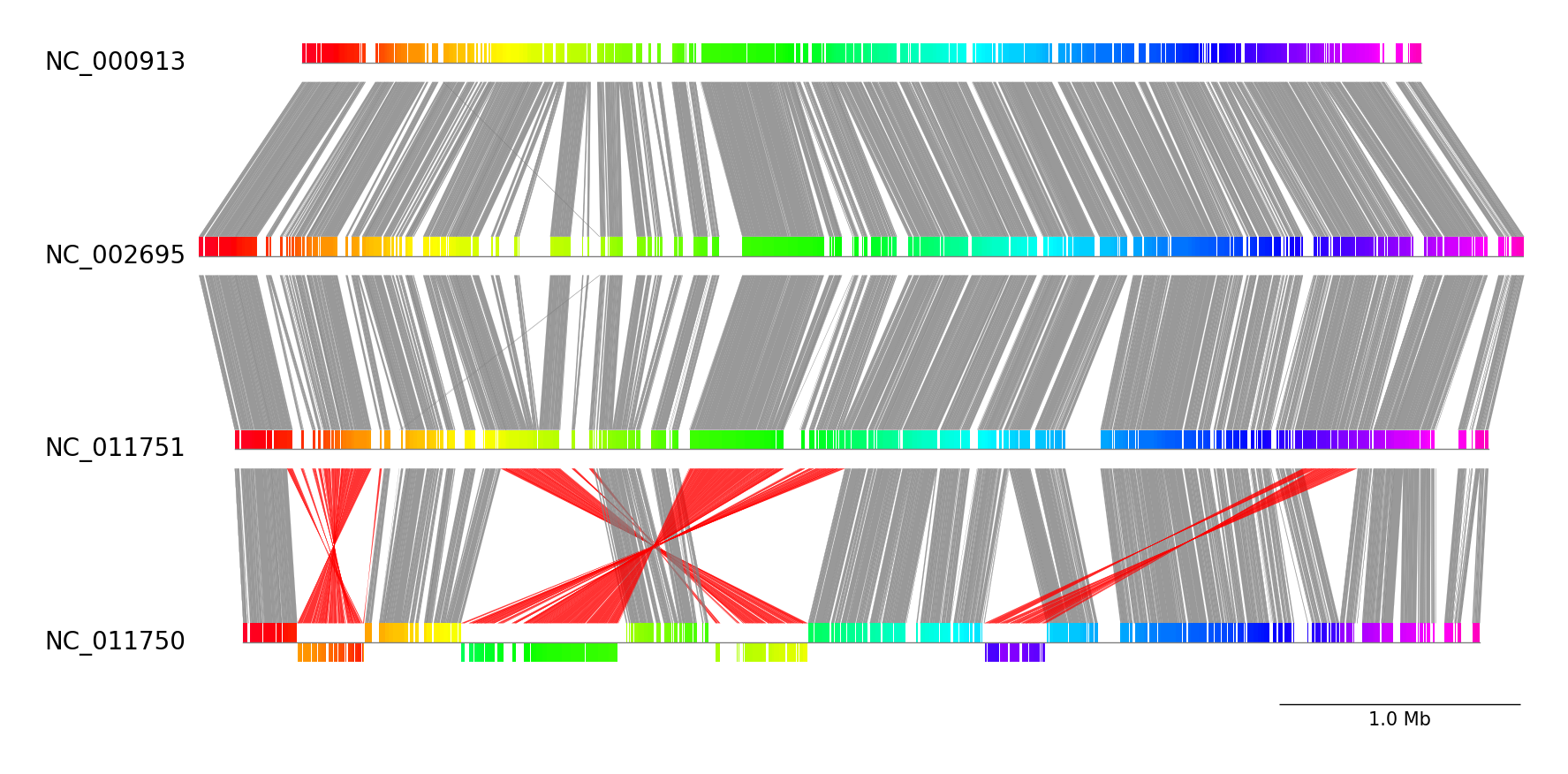
## GUI (Web Application)
pyGenomeViz implements GUI (Web Application) functionality using [streamlit](https://github.com/streamlit/streamlit) as an option.
Users can easily visualize the genomic features in Genbank files and their comparison results with GUI ([Demo Page](https://pygenomeviz.streamlit.app)).
See [pgv-gui document](https://moshi4.github.io/pyGenomeViz/gui-docs/pgv-gui/) for details.
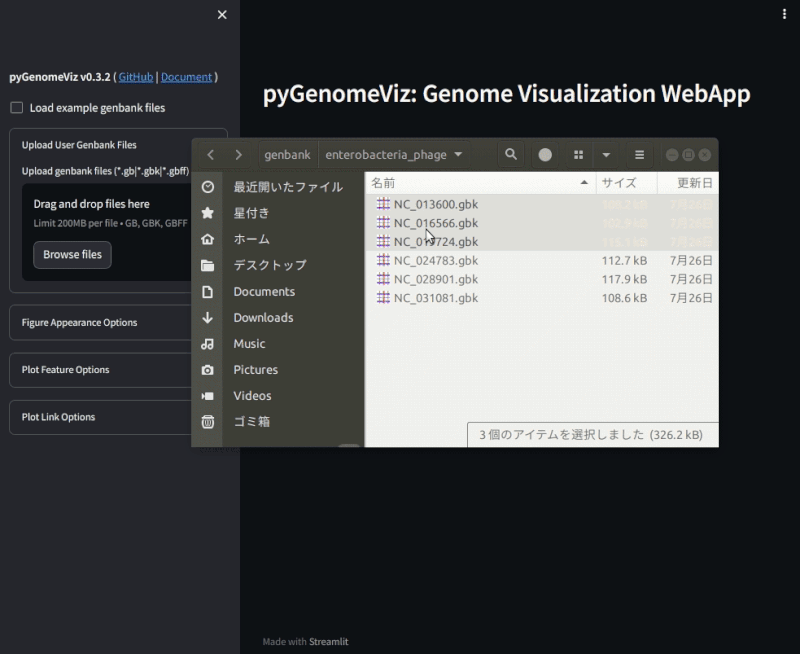
## HTML Viewer
pyGenomeViz implements HTML viewer output functionality for interactive data visualization.
In API, HTML file can be output using `savefig_html` method. In CLI, user can select HTML file output option.
As shown below, pan/zoom, tooltip display, object color change, text change, etc are available in HTML viewer
([Demo Page1](https://moshi4.github.io/pyGenomeViz/images/pgv-viewer_demo1.html), [Demo Page2](https://moshi4.github.io/pyGenomeViz/images/pgv-viewer_demo2.html)).
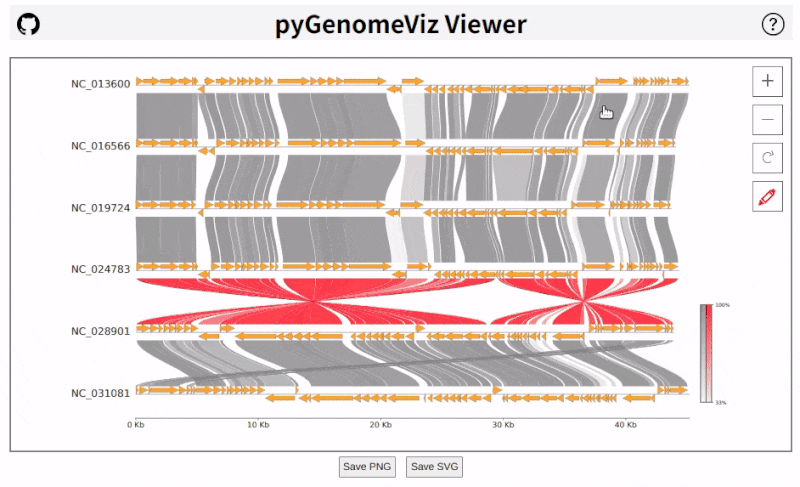
Following libraries were used to implement HTML viewer.
- [Spectrum](https://github.com/bgrins/spectrum): Colorpicker
- [Panzoom](https://github.com/timmywil/panzoom): SVG panning and zooming
- [Tabulator](https://github.com/olifolkerd/tabulator): Interactive Table
- [Micromodal](https://github.com/Ghosh/micromodal): Modal dialog
- [Tippy.js](https://github.com/atomiks/tippyjs): Tooltip
## Inspiration
pyGenomeViz was inspired by
- [GenomeDiagram (BioPython)](https://github.com/biopython/biopython)
- [Easyfig](http://mjsull.github.io/Easyfig/)
- [genoplotR](https://genoplotr.r-forge.r-project.org/)
- [gggenomes](https://github.com/thackl/gggenomes)
## Circular Genome Visualization
pyGenomeViz is a python package designed for linear genome visualization.
If you are interested in circular genome visualization, check out my other python package [pyCirclize](https://github.com/moshi4/pyCirclize).
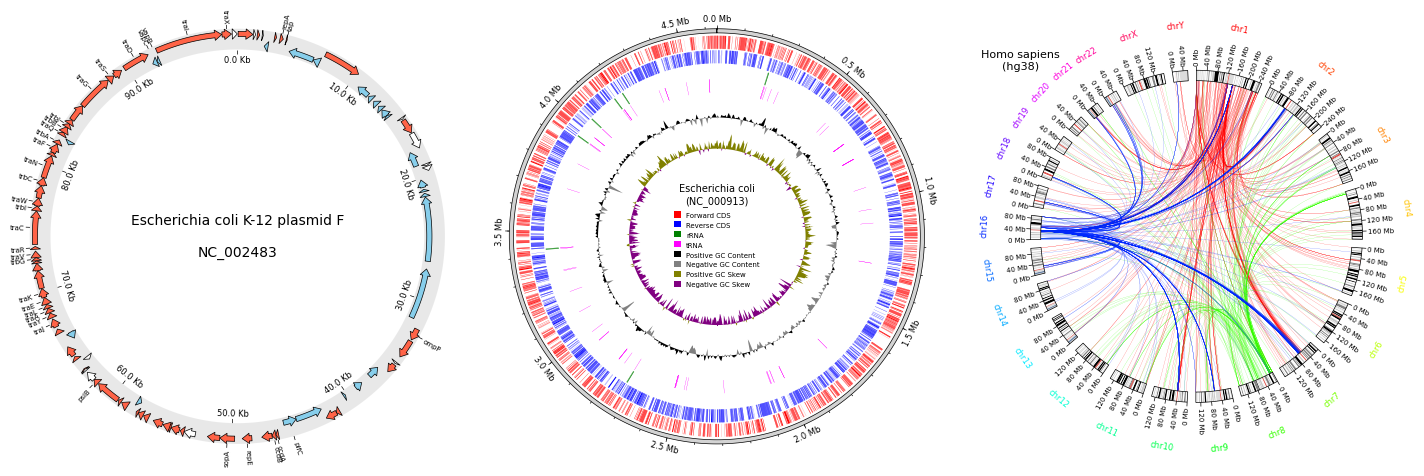
**Fig. pyCirclize example plot gallery**
## Star History
[](https://star-history.com/#moshi4/pyGenomeViz&Date)
Raw data
{
"_id": null,
"home_page": null,
"name": "pygenomeviz",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.9",
"maintainer_email": null,
"keywords": "bioinformatics, comparative-genomics, genomics, matplotlib, visualization",
"author": "moshi4",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/5a/25/8807ec8a918f3f776b159518f1aa4a9dc5bd04090d891b0b0daed2ebb651/pygenomeviz-1.5.0.tar.gz",
"platform": null,
"description": "# pyGenomeViz\n\n\n\n\n[](https://pypi.python.org/pypi/pygenomeviz)\n[](https://anaconda.org/conda-forge/pygenomeviz)\n[](https://github.com/moshi4/pyGenomeViz/actions/workflows/ci.yml)\n\n> [!NOTE]\n> A major version upgrade, pyGenomeViz **v1.0.0**, was released on 2024/05.\n> Backward incompatible changes have been made between v1.0.0 and v0.X.X to make for a more sophisticated API/CLI design.\n> Therefore, v0.X.X users should pin the version to v0.4.4 or update existing code for v1.0.0.\n> Previous v0.4.4 documentation is available [here](https://moshi4.github.io/docs/pygenomeviz/v0.4.4/).\n\n## Table of contents\n\n- [Overview](#overview)\n- [Installation](#installation)\n- [API Examples](#api-examples)\n- [CLI Examples](#cli-examples)\n- [GUI (Web Application)](#gui-web-application)\n- [HTML Viewer](#html-viewer)\n- [Inspiration](#inspiration)\n- [Circular Genome Visualization](#circular-genome-visualization)\n- [Star History](#star-history)\n\n## Overview\n\npyGenomeViz is a genome visualization python package for comparative genomics implemented based on matplotlib.\nThis package is developed for the purpose of easily and beautifully plotting genomic\nfeatures and sequence similarity comparison links between multiple genomes.\nIt supports genome visualization of Genbank/GFF format file and can be saved figure in various formats (JPG/PNG/SVG/PDF/HTML).\nUser can use pyGenomeViz for interactive genome visualization figure plotting on jupyter notebook,\nor automatic genome visualization figure plotting in genome analysis scripts/workflow.\n\nFor more information, please see full documentation [here](https://moshi4.github.io/pyGenomeViz/).\n\n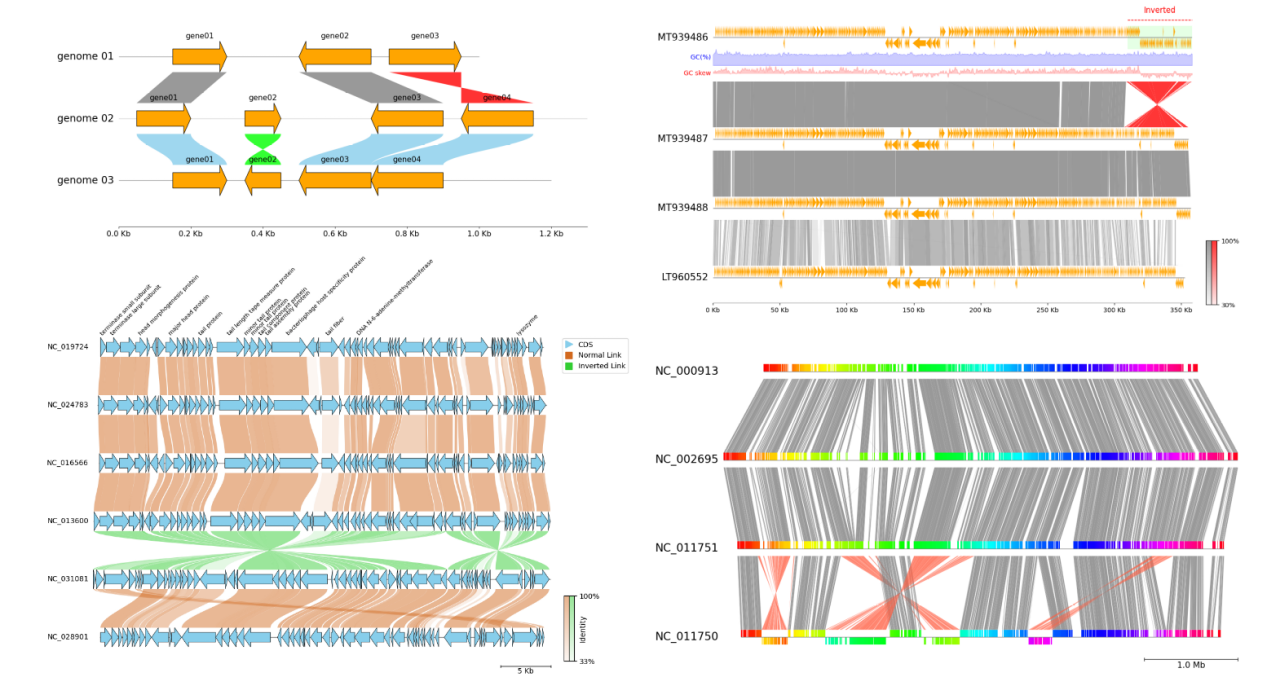 \n**Fig.1 pyGenomeViz example plot gallery**\n\n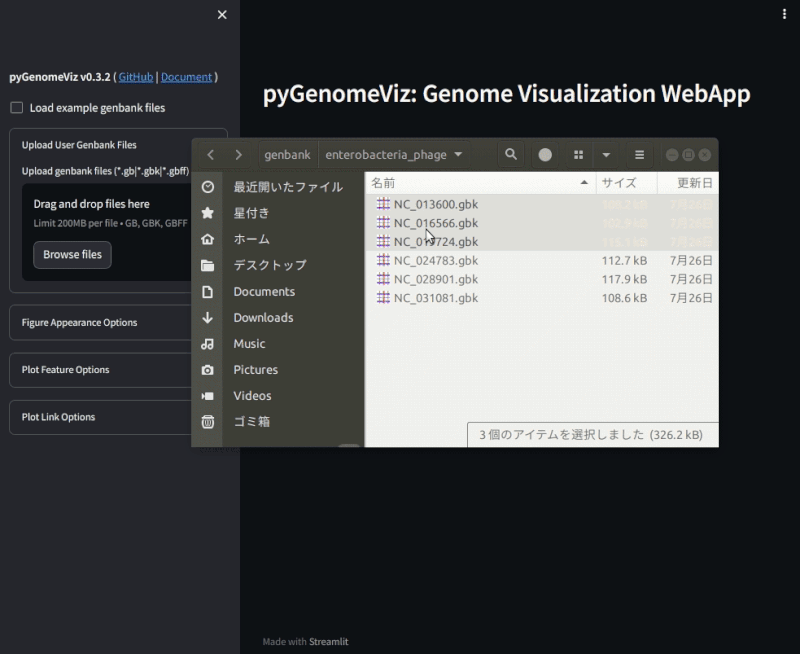\n**Fig.2 pyGenomeViz web application example ([Demo Page](https://pygenomeviz.streamlit.app))**\n\n## Installation\n\n`Python 3.9 or later` is required for installation.\n\n**Install PyPI package:**\n\n pip install pygenomeviz\n\n**Install conda-forge package:**\n\n conda install -c conda-forge pygenomeviz\n\n**Use Docker ([Image Registry](https://github.com/moshi4/pyGenomeViz/pkgs/container/pygenomeviz)):**\n\n docker run -it --rm -p 8501:8501 ghcr.io/moshi4/pygenomeviz:latest pgv-gui -h\n\n## API Examples\n\nJupyter notebooks containing code examples below is available [here](https://github.com/moshi4/pyGenomeViz/blob/main/notebooks/example.ipynb).\n\n### Features\n\n```python\nfrom pygenomeviz import GenomeViz\n\ngv = GenomeViz()\ngv.set_scale_xticks(ymargin=0.5)\n\ntrack = gv.add_feature_track(\"tutorial\", 1000)\ntrack.add_sublabel()\n\ntrack.add_feature(50, 200, 1)\ntrack.add_feature(250, 460, -1, fc=\"blue\")\ntrack.add_feature(500, 710, 1, fc=\"lime\")\ntrack.add_feature(750, 960, 1, fc=\"magenta\", lw=1.0)\n\ngv.savefig(\"features.png\")\n```\n\n\n\n### Styled Features\n\n```python\nfrom pygenomeviz import GenomeViz\n\ngv = GenomeViz()\ngv.set_scale_bar(ymargin=0.5)\n\ntrack = gv.add_feature_track(\"tutorial\", (1000, 2000))\ntrack.add_sublabel()\n\ntrack.add_feature(1050, 1150, 1, label=\"arrow\")\ntrack.add_feature(1200, 1300, -1, plotstyle=\"bigarrow\", label=\"bigarrow\", fc=\"red\", lw=1)\ntrack.add_feature(1330, 1400, 1, plotstyle=\"bigbox\", label=\"bigbox\", fc=\"blue\", text_kws=dict(rotation=0, hpos=\"center\"))\ntrack.add_feature(1420, 1500, 1, plotstyle=\"box\", label=\"box\", fc=\"limegreen\", text_kws=dict(size=10, color=\"blue\"))\ntrack.add_feature(1550, 1600, 1, plotstyle=\"bigrbox\", label=\"bigrbox\", fc=\"magenta\", ec=\"blue\", lw=1, text_kws=dict(rotation=0, vpos=\"bottom\", hpos=\"center\"))\ntrack.add_feature(1650, 1750, -1, plotstyle=\"rbox\", label=\"rbox\", fc=\"grey\", text_kws=dict(rotation=-45, vpos=\"bottom\"))\ntrack.add_feature(1780, 1880, 1, fc=\"lime\", hatch=\"o\", arrow_shaft_ratio=0.2, label=\"arrow shaft\\n0.2\", text_kws=dict(rotation=0, hpos=\"center\"))\ntrack.add_feature(1890, 1990, 1, fc=\"lime\", hatch=\"/\", arrow_shaft_ratio=1.0, label=\"arrow shaft\\n1.0\", text_kws=dict(rotation=0, hpos=\"center\"))\n\ngv.savefig(\"styled_features.png\")\n```\n\n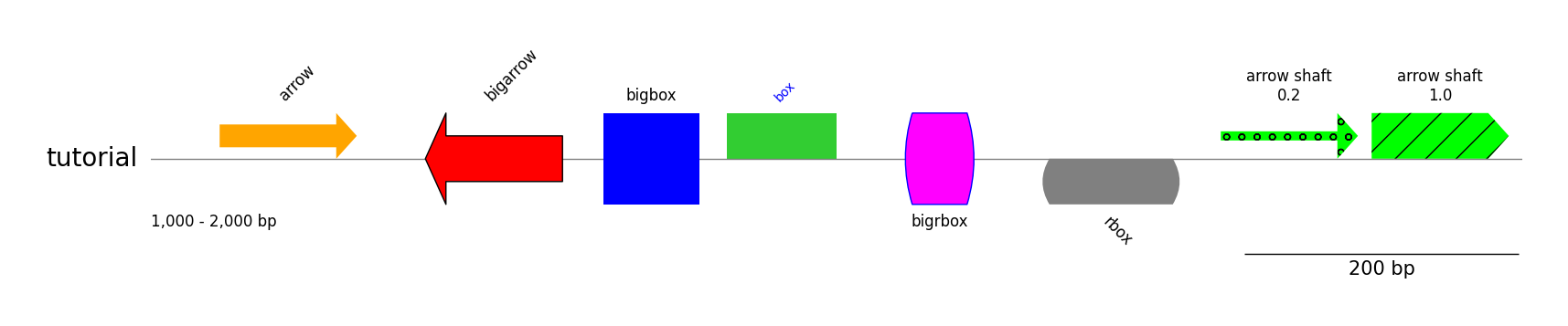\n\n### Tracks & Links\n\n```python\nfrom pygenomeviz import GenomeViz\n\ngenome_list = [\n dict(name=\"genome 01\", size=1000, features=((150, 300, 1), (500, 700, -1), (750, 950, 1))),\n dict(name=\"genome 02\", size=1300, features=((50, 200, 1), (350, 450, 1), (700, 900, -1), (950, 1150, -1))),\n dict(name=\"genome 03\", size=1200, features=((150, 300, 1), (350, 450, -1), (500, 700, -1), (700, 900, -1))),\n]\n\ngv = GenomeViz(track_align_type=\"center\")\ngv.set_scale_bar()\n\nfor genome in genome_list:\n name, size, features = genome[\"name\"], genome[\"size\"], genome[\"features\"]\n track = gv.add_feature_track(name, size)\n track.add_sublabel()\n for idx, feature in enumerate(features, 1):\n start, end, strand = feature\n track.add_feature(start, end, strand, plotstyle=\"bigarrow\", lw=1, label=f\"gene{idx:02d}\", text_kws=dict(rotation=0, vpos=\"top\", hpos=\"center\"))\n\n# Add links between \"genome 01\" and \"genome 02\"\ngv.add_link((\"genome 01\", 150, 300), (\"genome 02\", 50, 200))\ngv.add_link((\"genome 01\", 700, 500), (\"genome 02\", 900, 700))\ngv.add_link((\"genome 01\", 750, 950), (\"genome 02\", 1150, 950))\n# Add links between \"genome 02\" and \"genome 03\"\ngv.add_link((\"genome 02\", 50, 200), (\"genome 03\", 150, 300), color=\"skyblue\", inverted_color=\"lime\", curve=True)\ngv.add_link((\"genome 02\", 350, 450), (\"genome 03\", 450, 350), color=\"skyblue\", inverted_color=\"lime\", curve=True)\ngv.add_link((\"genome 02\", 900, 700), (\"genome 03\", 700, 500), color=\"skyblue\", inverted_color=\"lime\", curve=True)\ngv.add_link((\"genome 03\", 900, 700), (\"genome 02\", 1150, 950), color=\"skyblue\", inverted_color=\"lime\", curve=True)\n\ngv.savefig(\"tracks_and_links.png\")\n```\n\n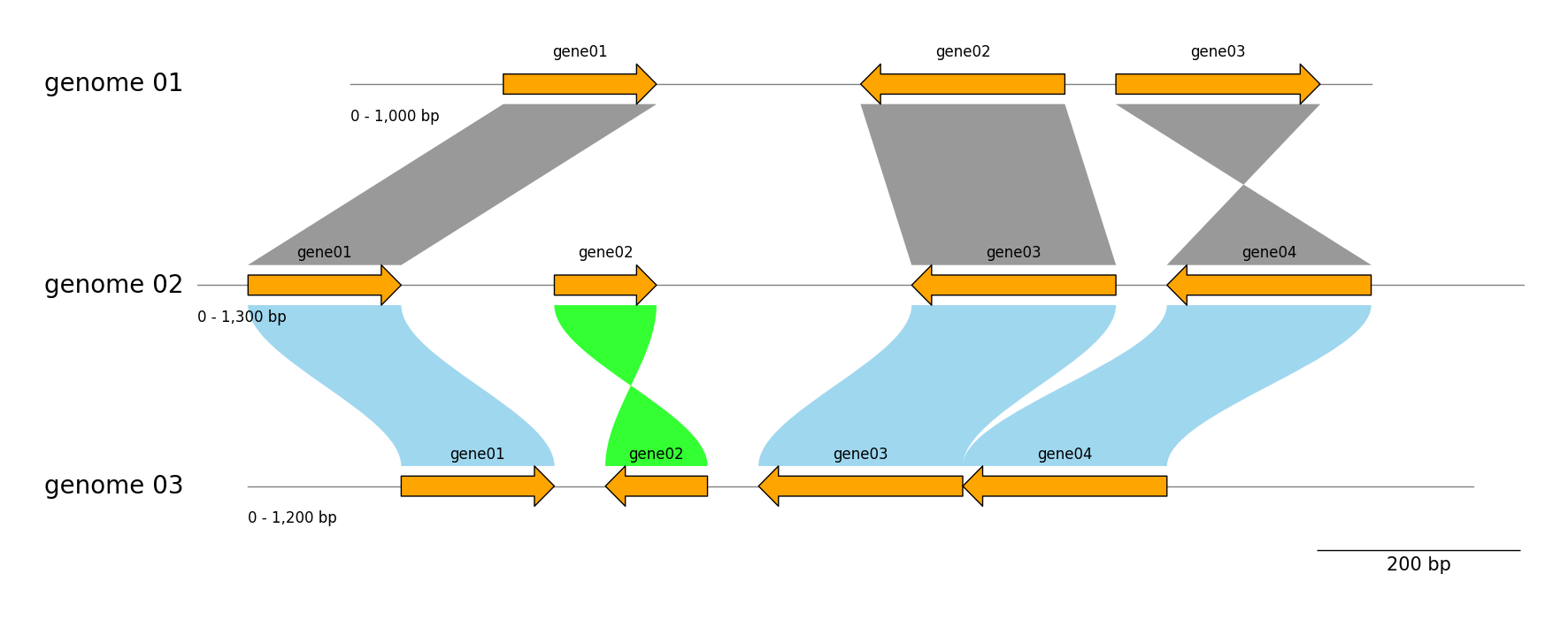\n\n### Exon Features\n\n```python\nfrom pygenomeviz import GenomeViz\n\nexon_regions1 = [(0, 210), (300, 480), (590, 800), (850, 1000), (1030, 1300)]\nexon_regions2 = [(1500, 1710), (2000, 2480), (2590, 2800)]\nexon_regions3 = [(3000, 3300), (3400, 3690), (3800, 4100), (4200, 4620)]\n\ngv = GenomeViz()\ntrack = gv.add_feature_track(\"Exon Features\", 5000)\ntrack.add_exon_feature(exon_regions1, strand=1, plotstyle=\"box\", label=\"box\", text_kws=dict(rotation=0, hpos=\"center\"))\ntrack.add_exon_feature(exon_regions2, strand=-1, plotstyle=\"arrow\", label=\"arrow\", text_kws=dict(rotation=0, vpos=\"bottom\", hpos=\"center\"), patch_kws=dict(fc=\"darkgrey\"), intron_patch_kws=dict(ec=\"red\"))\ntrack.add_exon_feature(exon_regions3, strand=1, plotstyle=\"bigarrow\", label=\"bigarrow\", text_kws=dict(rotation=0, hpos=\"center\"), patch_kws=dict(fc=\"lime\", lw=1))\n\ngv.savefig(\"exon_features.png\")\n```\n\n\n\n### Genbank Features\n\n```python\nfrom pygenomeviz import GenomeViz\nfrom pygenomeviz.parser import Genbank\nfrom pygenomeviz.utils import load_example_genbank_dataset\n\ngbk_files = load_example_genbank_dataset(\"yersinia_phage\")\ngbk = Genbank(gbk_files[0])\n\ngv = GenomeViz()\ngv.set_scale_bar(ymargin=0.5)\n\ntrack = gv.add_feature_track(gbk.name, gbk.genome_length)\ntrack.add_sublabel()\n\nfeatures = gbk.extract_features()\ntrack.add_features(features)\n\ngv.savefig(\"genbank_features.png\")\n```\n\n\n\n### GFF Features\n\n```python\nfrom pygenomeviz import GenomeViz\nfrom pygenomeviz.parser import Gff\nfrom pygenomeviz.utils import load_example_gff_file\n\ngff_file = load_example_gff_file(\"escherichia_coli.gff.gz\")\ngff = Gff(gff_file)\n\ngv = GenomeViz()\ngv.set_scale_bar(ymargin=0.5)\n\ntarget_ranges = ((220000, 230000), (300000, 310000))\ntrack = gv.add_feature_track(name=gff.name, segments=target_ranges)\ntrack.set_segment_sep(symbol=\"//\")\n\nfor segment in track.segments:\n segment.add_sublabel()\n # Plot CDS features\n cds_features = gff.extract_features(feature_type=\"CDS\", target_range=segment.range)\n segment.add_features(cds_features, label_type=\"gene\", fc=\"skyblue\", lw=1.0)\n # Plot rRNA features\n rrna_features = gff.extract_features(feature_type=\"rRNA\", target_range=segment.range)\n segment.add_features(rrna_features, label_type=\"product\", hatch=\"//\", fc=\"lime\", lw=1.0)\n\ngv.savefig(\"gff_features.png\")\n```\n\n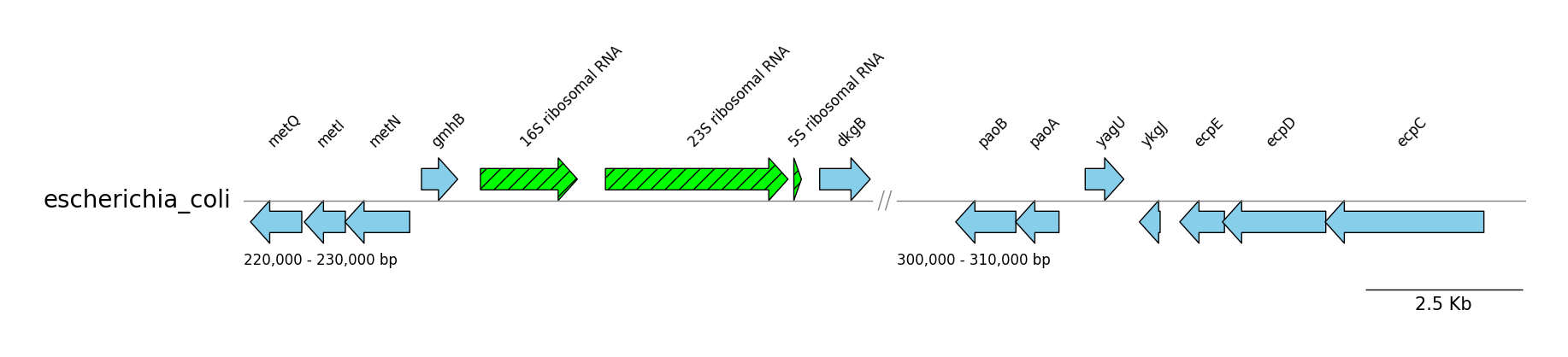\n\n### GFF Contigs\n\n```python\nfrom pygenomeviz import GenomeViz\nfrom pygenomeviz.parser import Gff\nfrom pygenomeviz.utils import load_example_gff_file, is_pseudo_feature\n\ngff_file = load_example_gff_file(\"mycoplasma_mycoides.gff\")\ngff = Gff(gff_file)\n\ngv = GenomeViz(fig_track_height=0.5, feature_track_ratio=0.5)\ngv.set_scale_xticks(labelsize=10)\n\n# Plot CDS, rRNA features for each contig to tracks\nfor seqid, size in gff.get_seqid2size().items():\n track = gv.add_feature_track(seqid, size, labelsize=15)\n track.add_sublabel(size=10, color=\"grey\")\n cds_features = gff.get_seqid2features(feature_type=\"CDS\")[seqid]\n # CDS: blue, CDS(pseudo): grey\n for cds_feature in cds_features:\n color = \"grey\" if is_pseudo_feature(cds_feature) else \"blue\"\n track.add_features(cds_feature, color=color)\n # rRNA: lime\n rrna_features = gff.get_seqid2features(feature_type=\"rRNA\")[seqid]\n track.add_features(rrna_features, color=\"lime\")\n\ngv.savefig(\"gff_contigs.png\")\n```\n\n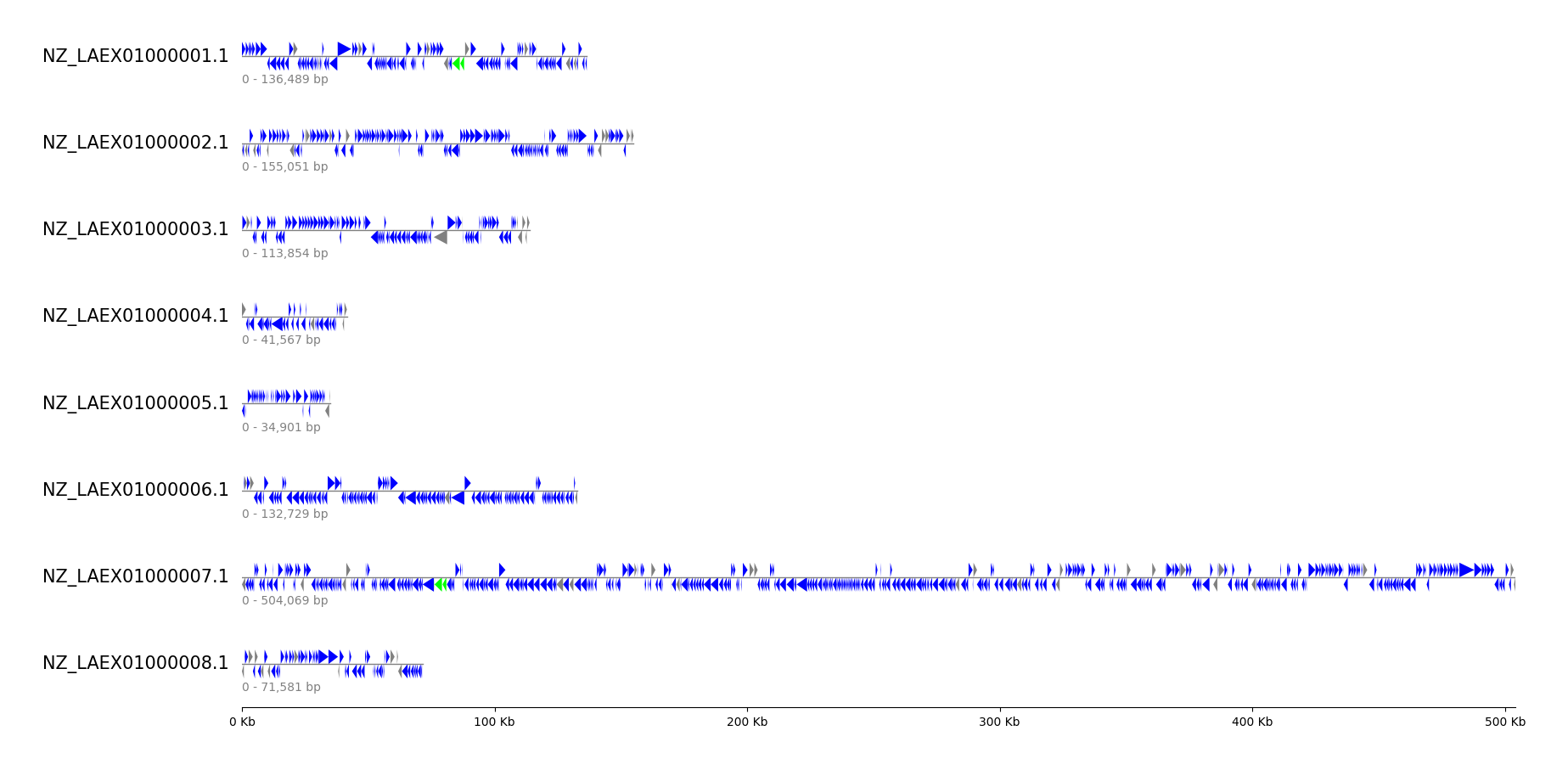\n\n### Genbank Comparison by BLAST\n\n```python\nfrom pygenomeviz import GenomeViz\nfrom pygenomeviz.parser import Genbank\nfrom pygenomeviz.utils import load_example_genbank_dataset\nfrom pygenomeviz.align import Blast, AlignCoord\n\ngbk_files = load_example_genbank_dataset(\"yersinia_phage\")\ngbk_list = list(map(Genbank, gbk_files))\n\ngv = GenomeViz(track_align_type=\"center\")\ngv.set_scale_bar()\n\n# Plot CDS features\nfor gbk in gbk_list:\n track = gv.add_feature_track(gbk.name, gbk.get_seqid2size(), align_label=False)\n for seqid, features in gbk.get_seqid2features(\"CDS\").items():\n segment = track.get_segment(seqid)\n segment.add_features(features, plotstyle=\"bigarrow\", fc=\"limegreen\", lw=0.5)\n\n# Run BLAST alignment & filter by user-defined threshold\nalign_coords = Blast(gbk_list, seqtype=\"protein\").run()\nalign_coords = AlignCoord.filter(align_coords, length_thr=100, identity_thr=30)\n\n# Plot BLAST alignment links\nif len(align_coords) > 0:\n min_ident = int(min([ac.identity for ac in align_coords if ac.identity]))\n color, inverted_color = \"grey\", \"red\"\n for ac in align_coords:\n gv.add_link(ac.query_link, ac.ref_link, color=color, inverted_color=inverted_color, v=ac.identity, vmin=min_ident)\n gv.set_colorbar([color, inverted_color], vmin=min_ident)\n\ngv.savefig(\"genbank_comparison_by_blast.png\")\n```\n\n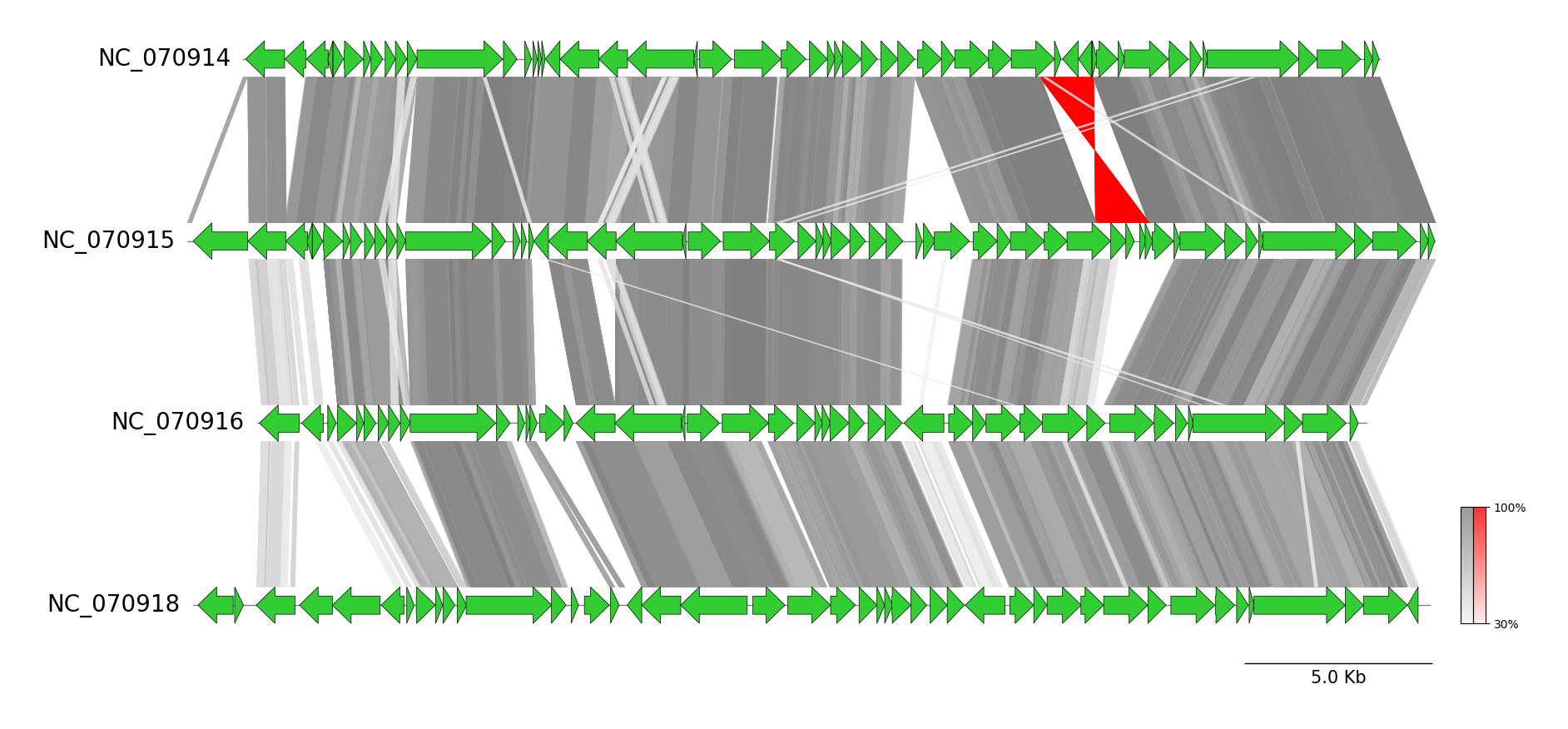\n\n## CLI Examples\n\npyGenomeViz provides CLI workflows for genome alignment result visualization of\nGenbank genomes using `BLAST` / `MUMmer` / `MMseqs` / `progressiveMauve`, respectively.\n\n### BLAST CLI Workflow\n\nSee [pgv-blast document](https://moshi4.github.io/pyGenomeViz/cli-docs/pgv-blast/) for details.\n\n```shell\n# Download example dataset\npgv-download yersinia_phage\n# Run BLAST CLI workflow\npgv-blast NC_070914.gbk NC_070915.gbk NC_070916.gbk NC_070918.gbk \\\n -o pgv-blast_example --seqtype protein --show_scale_bar --curve \\\n --feature_linewidth 0.3 --length_thr 100 --identity_thr 30\n```\n\n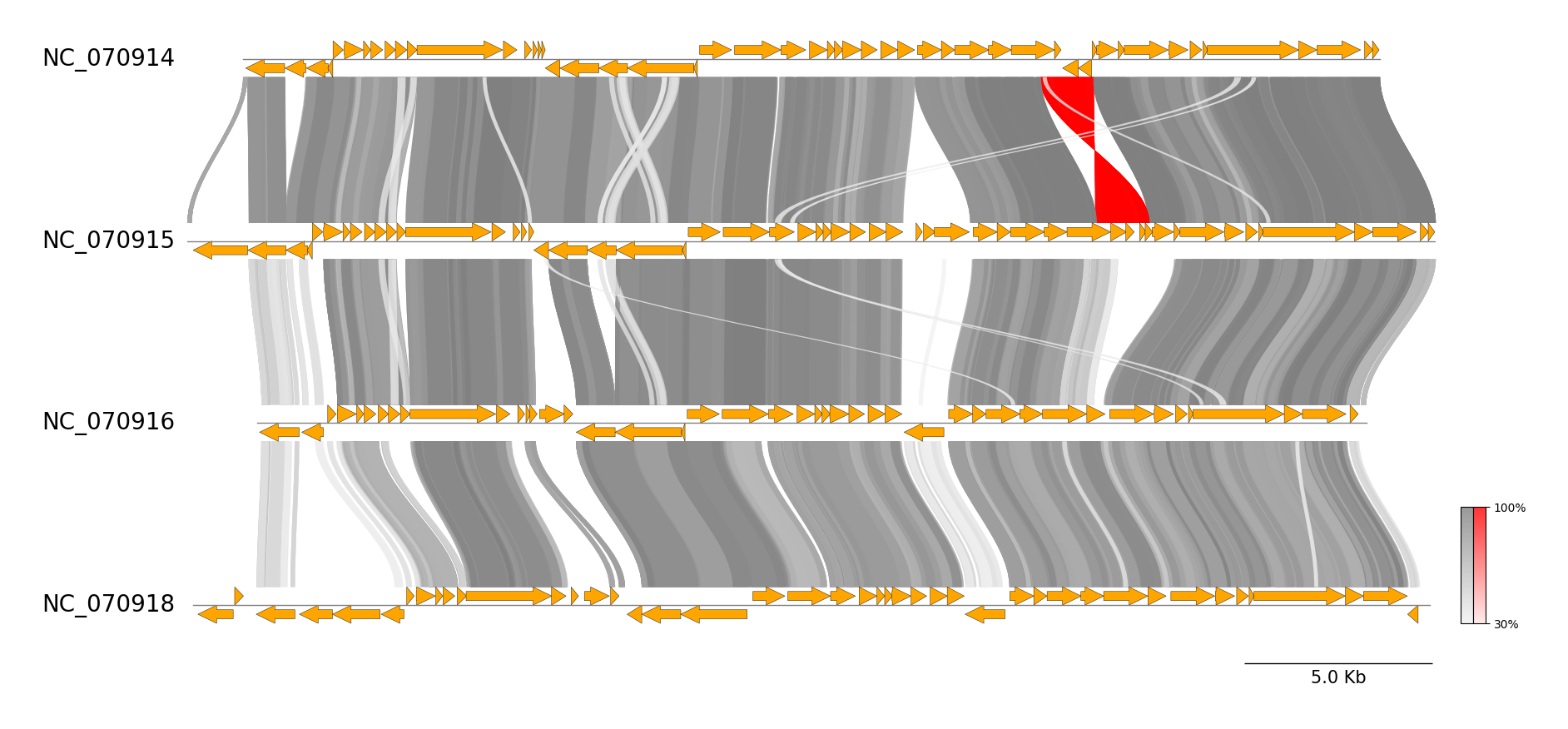 \n\n### MUMmer CLI Workflow\n\nSee [pgv-mummer document](https://moshi4.github.io/pyGenomeViz/cli-docs/pgv-mummer/) for details.\n\n```shell\n# Download example dataset\npgv-download mycoplasma_mycoides\n# Run MUMmer CLI workflow\npgv-mummer GCF_000023685.1.gbff GCF_000800785.1.gbff GCF_000959055.1.gbff GCF_000959065.1.gbff \\\n -o pgv-mummer_example --show_scale_bar --curve \\\n --feature_type2color CDS:blue rRNA:lime tRNA:magenta\n```\n\n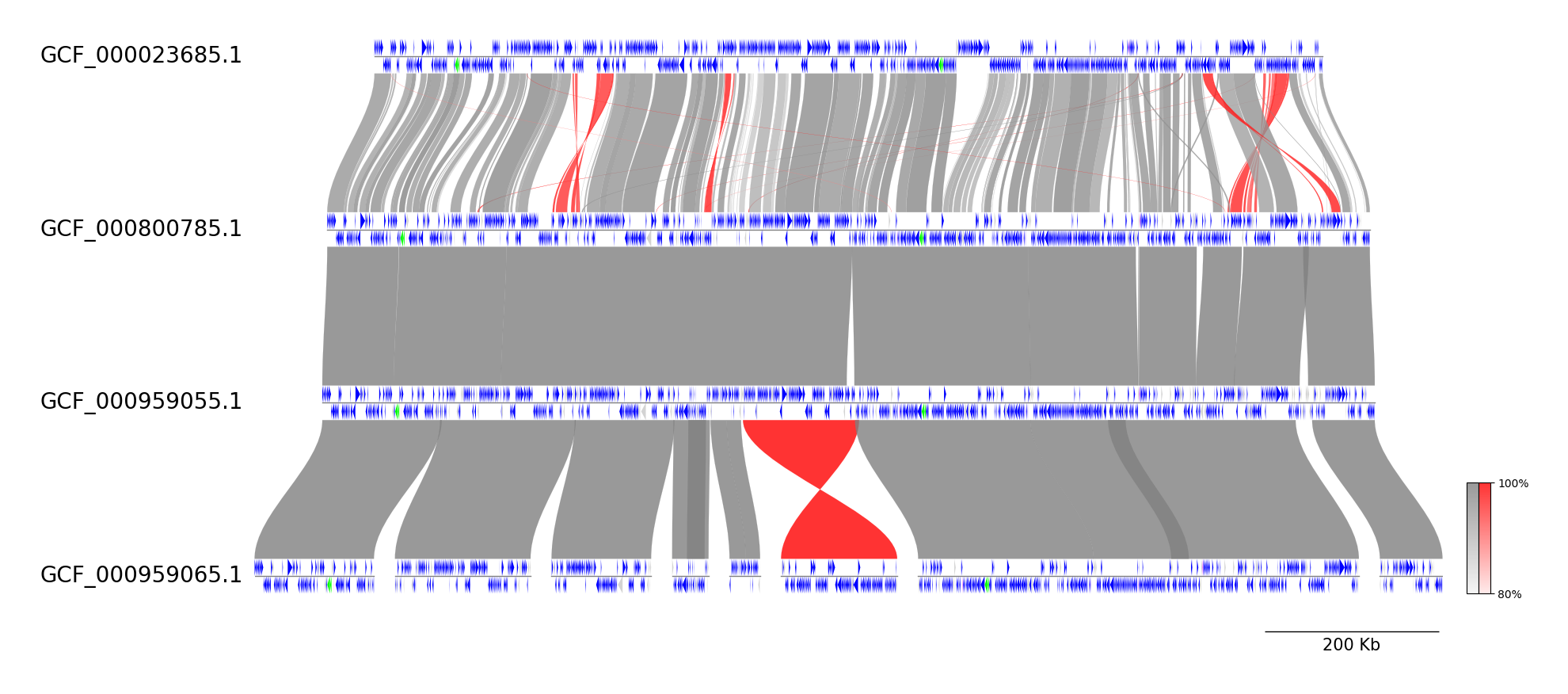 \n\n### MMseqs CLI Workflow\n\nSee [pgv-mmseqs document](https://moshi4.github.io/pyGenomeViz/cli-docs/pgv-mmseqs/) for details.\n\n```shell\n# Download example dataset\npgv-download enterobacteria_phage\n# Run MMseqs CLI workflow\npgv-mmseqs NC_013600.gbk NC_016566.gbk NC_019724.gbk NC_024783.gbk NC_028901.gbk NC_031081.gbk \\\n -o pgv-mmseqs_example --show_scale_bar --curve --feature_linewidth 0.3 \\\n --feature_type2color CDS:skyblue --normal_link_color chocolate --inverted_link_color limegreen\n```\n\n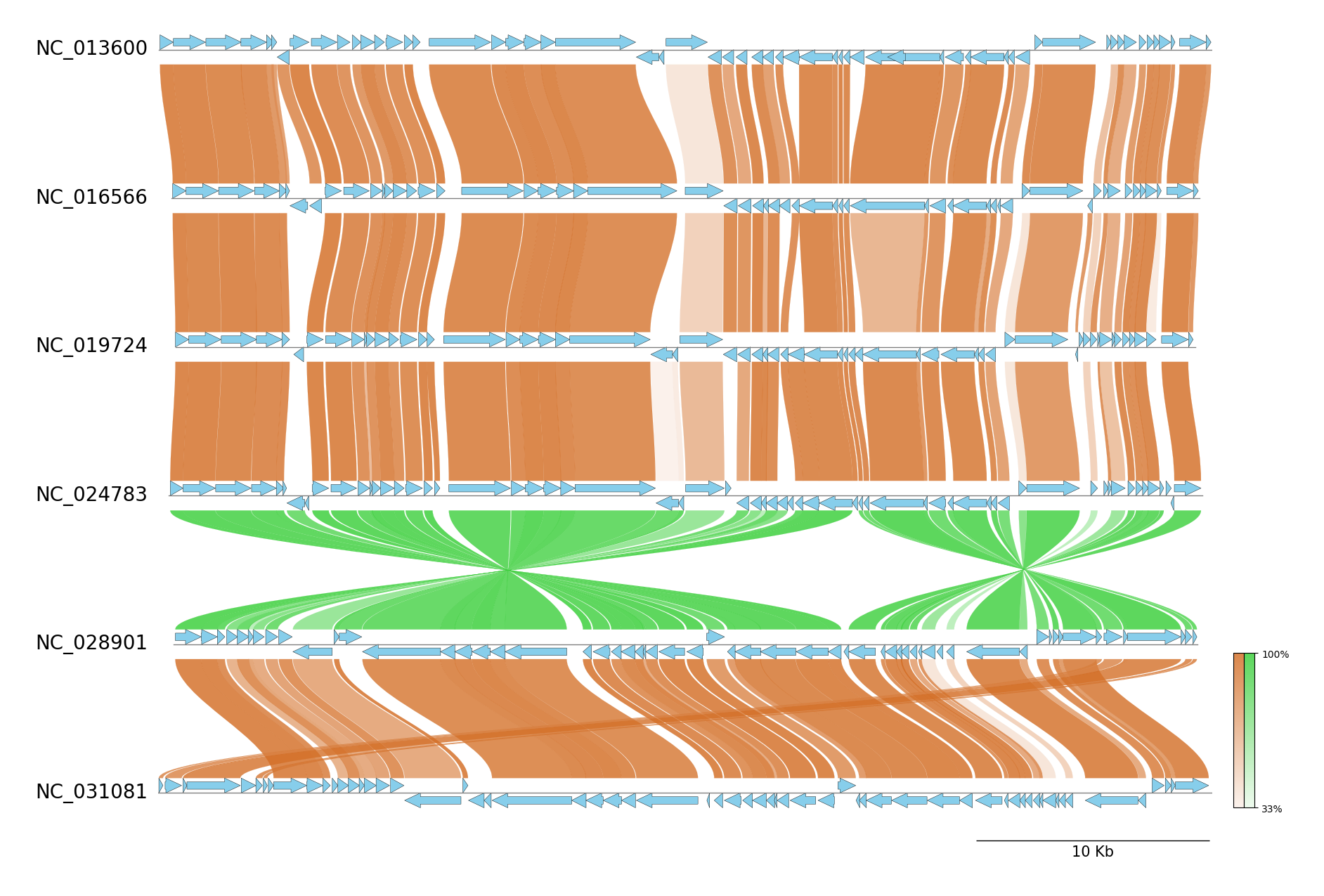 \n\n### progressiveMauve CLI Workflow\n\nSee [pgv-pmauve document](https://moshi4.github.io/pyGenomeViz/cli-docs/pgv-pmauve/) for details.\n\n```shell\n# Download example dataset\npgv-download escherichia_coli\n# Run progressiveMauve CLI workflow\npgv-pmauve NC_000913.gbk.gz NC_002695.gbk.gz NC_011751.gbk.gz NC_011750.gbk.gz \\\n -o pgv-pmauve_example --show_scale_bar\n```\n\n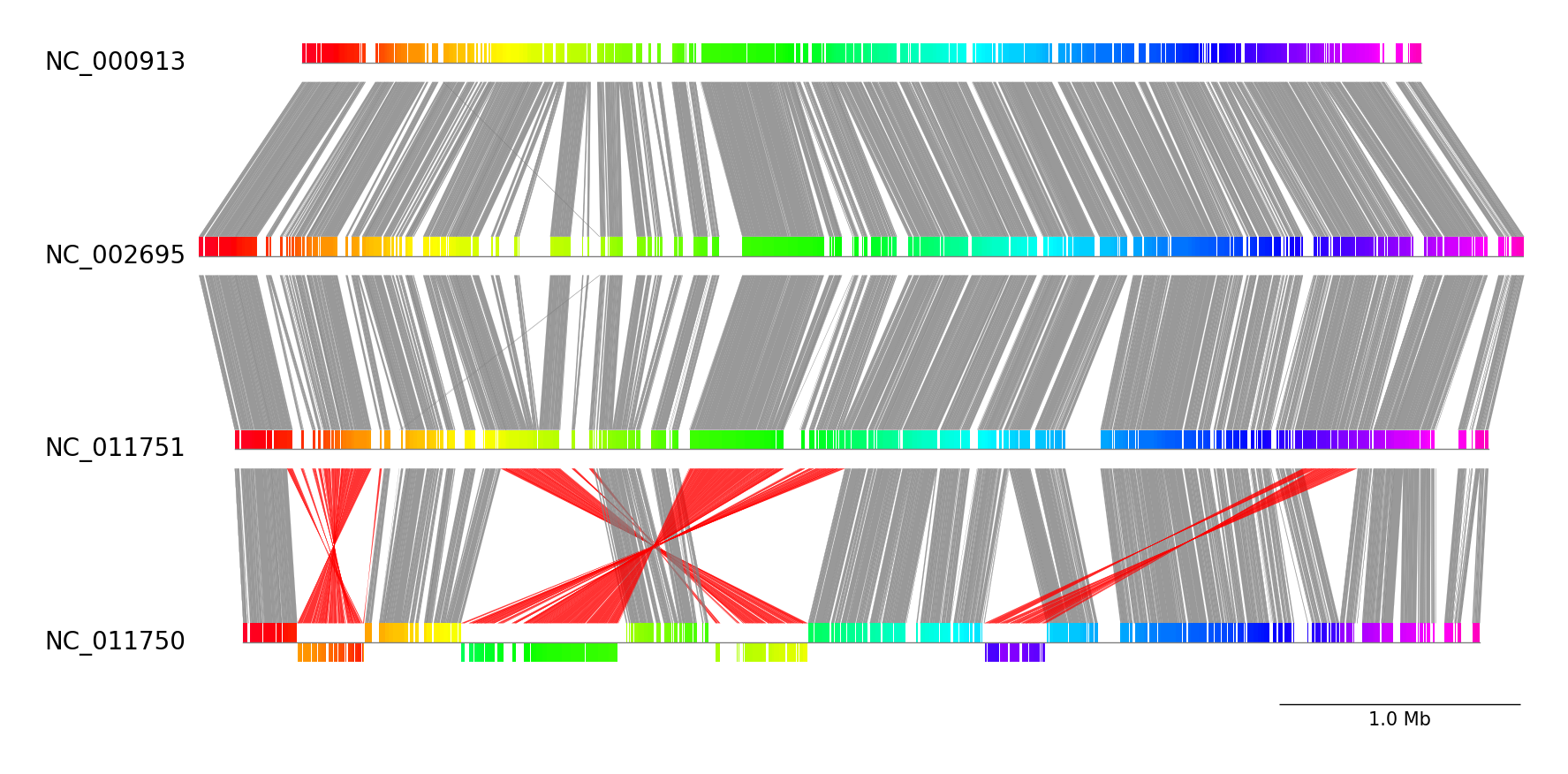 \n\n## GUI (Web Application)\n\npyGenomeViz implements GUI (Web Application) functionality using [streamlit](https://github.com/streamlit/streamlit) as an option.\nUsers can easily visualize the genomic features in Genbank files and their comparison results with GUI ([Demo Page](https://pygenomeviz.streamlit.app)).\nSee [pgv-gui document](https://moshi4.github.io/pyGenomeViz/gui-docs/pgv-gui/) for details.\n\n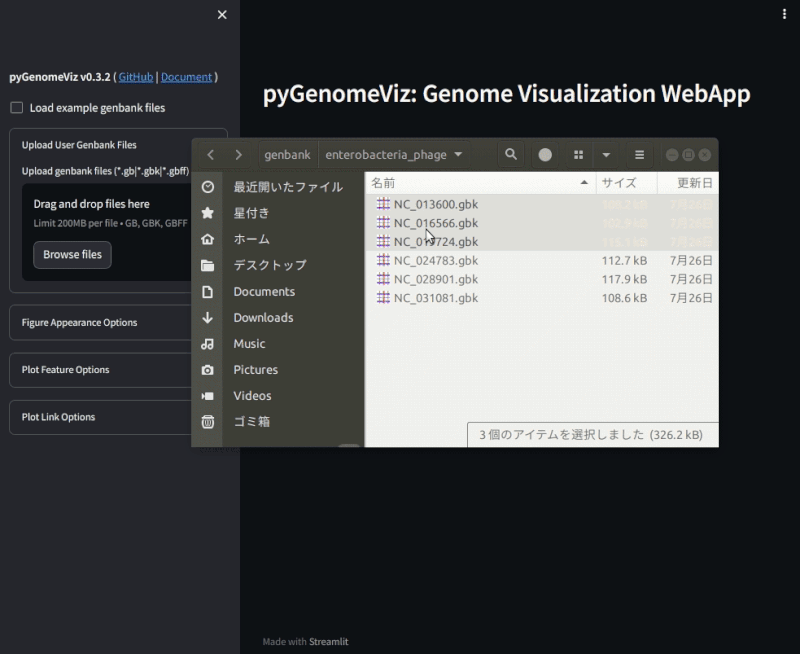\n\n## HTML Viewer\n\npyGenomeViz implements HTML viewer output functionality for interactive data visualization.\nIn API, HTML file can be output using `savefig_html` method. In CLI, user can select HTML file output option.\nAs shown below, pan/zoom, tooltip display, object color change, text change, etc are available in HTML viewer\n([Demo Page1](https://moshi4.github.io/pyGenomeViz/images/pgv-viewer_demo1.html), [Demo Page2](https://moshi4.github.io/pyGenomeViz/images/pgv-viewer_demo2.html)).\n\n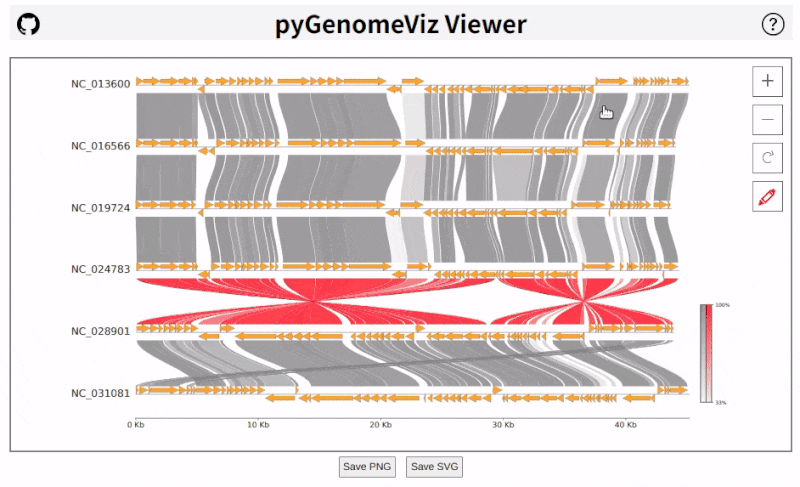\n\nFollowing libraries were used to implement HTML viewer. \n\n- [Spectrum](https://github.com/bgrins/spectrum): Colorpicker \n- [Panzoom](https://github.com/timmywil/panzoom): SVG panning and zooming \n- [Tabulator](https://github.com/olifolkerd/tabulator): Interactive Table\n- [Micromodal](https://github.com/Ghosh/micromodal): Modal dialog\n- [Tippy.js](https://github.com/atomiks/tippyjs): Tooltip\n\n## Inspiration\n\npyGenomeViz was inspired by\n\n- [GenomeDiagram (BioPython)](https://github.com/biopython/biopython)\n- [Easyfig](http://mjsull.github.io/Easyfig/)\n- [genoplotR](https://genoplotr.r-forge.r-project.org/)\n- [gggenomes](https://github.com/thackl/gggenomes)\n\n## Circular Genome Visualization\n\npyGenomeViz is a python package designed for linear genome visualization.\nIf you are interested in circular genome visualization, check out my other python package [pyCirclize](https://github.com/moshi4/pyCirclize).\n\n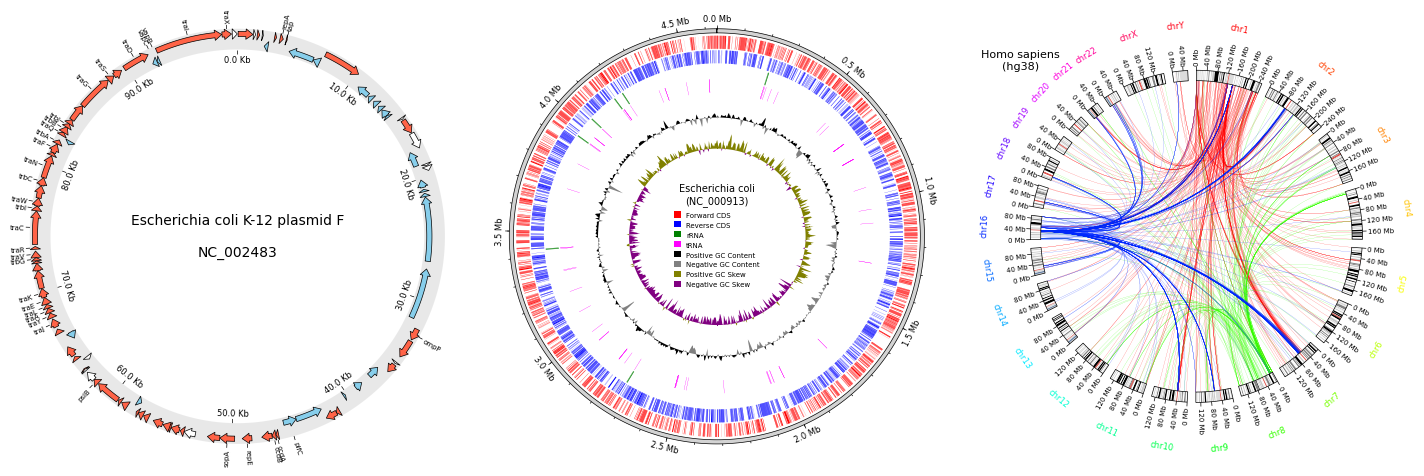 \n**Fig. pyCirclize example plot gallery**\n\n## Star History\n\n[](https://star-history.com/#moshi4/pyGenomeViz&Date)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A genome visualization python package for comparative genomics",
"version": "1.5.0",
"project_urls": {
"homepage": "https://moshi4.github.io/pyGenomeViz/",
"repository": "https://github.com/moshi4/pyGenomeViz/"
},
"split_keywords": [
"bioinformatics",
" comparative-genomics",
" genomics",
" matplotlib",
" visualization"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "79548efdbd8f26b6ffb1a41934d60b6a0cb0cae49347598d77fadcc9fe05bcba",
"md5": "ddbe8a69d1881a8c12884d3efa658425",
"sha256": "d5403c3a3f5500dfa964435dab41ac70fee6eb7c9832427ccbcef516f57e866a"
},
"downloads": -1,
"filename": "pygenomeviz-1.5.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "ddbe8a69d1881a8c12884d3efa658425",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.9",
"size": 1730302,
"upload_time": "2024-12-21T02:44:36",
"upload_time_iso_8601": "2024-12-21T02:44:36.538102Z",
"url": "https://files.pythonhosted.org/packages/79/54/8efdbd8f26b6ffb1a41934d60b6a0cb0cae49347598d77fadcc9fe05bcba/pygenomeviz-1.5.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5a258807ec8a918f3f776b159518f1aa4a9dc5bd04090d891b0b0daed2ebb651",
"md5": "1c07ad7a380227222d17d6db35f189bd",
"sha256": "3465d1148fcfaa21ec1b34985aeacb8a81c6d7e4adc2537f654baeb4c5b365de"
},
"downloads": -1,
"filename": "pygenomeviz-1.5.0.tar.gz",
"has_sig": false,
"md5_digest": "1c07ad7a380227222d17d6db35f189bd",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.9",
"size": 19568925,
"upload_time": "2024-12-21T02:44:56",
"upload_time_iso_8601": "2024-12-21T02:44:56.653742Z",
"url": "https://files.pythonhosted.org/packages/5a/25/8807ec8a918f3f776b159518f1aa4a9dc5bd04090d891b0b0daed2ebb651/pygenomeviz-1.5.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-12-21 02:44:56",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "moshi4",
"github_project": "pyGenomeViz",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "requirements.lock",
"specs": []
}
],
"lcname": "pygenomeviz"
}