# quick_trade
[](https://vshymanskyy.github.io/StandWithUkraine)
[](https://pepy.tech/project/quick-trade)
[)](https://pepy.tech/project/quick-trade)

```
Dependencies:
├──ta (Bukosabino https://github.com/bukosabino/ta (by Darío López Padial))
├──plotly (https://github.com/plotly/plotly.py)
├──pandas (https://github.com/pandas-dev/pandas)
├──numpy (https://github.com/numpy/numpy)
├──tqdm (https://github.com/tqdm/tqdm)
├──scikit-learn (https://github.com/scikit-learn/scikit-learn)
└──ccxt (https://github.com/ccxt/ccxt)
```
* **Documentation:** 🚧 https://quick-trade.github.io/quick_trade/#/ 🚧
* **Twitter:** [@quick_trade_tw](https://twitter.com/quick_trade_tw)
* **Discord**: [quick_trade](https://discord.gg/SkRg9mzuB5)
## Installation:
Quick install:
```commandline
$ pip3 install quick-trade
```
For development:
```commandline
$ git clone https://github.com/quick-trade/quick_trade.git
$ pip3 install -r quick_trade/requirements.txt
$ cd quick_trade
$ python3 setup.py install
$ cd ..
```
## Customize your strategy!
```python
from quick_trade.plots import TraderGraph, make_trader_figure
import ccxt
from quick_trade import strategy, TradingClient, Trader
from quick_trade.utils import TradeSide
class MyTrader(qtr.Trader):
@strategy
def strategy_sell_and_hold(self):
ret = []
for i in self.df['Close'].values:
ret.append(TradeSide.SELL)
self.returns = ret
self.set_credit_leverages(2) # if you want to use a leverage
self.set_open_stop_and_take(stop)
# or... set a stop loss with only one line of code
return ret
client = TradingClient(ccxt.binance())
df = client.get_data_historical("BTC/USDT")
trader = MyTrader("BTC/USDT", df=df)
trader.connect_graph(TraderGraph(make_trader_figure()))
trader.set_client(client)
trader.strategy_sell_and_hold()
trader.backtest()
```
## Find the best strategy!
```python
import quick_trade as qtr
import ccxt
from quick_trade.tuner import *
from quick_trade import TradingClient
class Test(qtr.ExampleStrategies):
@strategy
def strategy_supertrend1(self, plot: bool = False, *st_args, **st_kwargs):
self.strategy_supertrend(plot=plot, *st_args, **st_kwargs)
self.convert_signal() # only long trades
return self.returns
@strategy
def macd(self, histogram=False, **kwargs):
if not histogram:
self.strategy_macd(**kwargs)
else:
self.strategy_macd_histogram_diff(**kwargs)
self.convert_signal()
return self.returns
@strategy
def psar(self, **kwargs):
self.strategy_parabolic_SAR(plot=False, **kwargs)
self.convert_signal()
return self.returns
params = {
'strategy_supertrend1':
[
{
'multiplier': Linspace(0.5, 22, 5)
}
],
'macd':
[
{
'slow': Linspace(10, 100, 3),
'fast': Linspace(3, 60, 3),
'histogram': Choise([False, True])
}
],
'psar':
[
{
'step': 0.01,
'max_step': 0.1
},
{
'step': 0.02,
'max_step': 0.2
}
]
}
tuner = QuickTradeTuner(
TradingClient(ccxt.binance()),
['BTC/USDT', 'OMG/USDT', 'XRP/USDT'],
['15m', '5m'],
[1000, 700, 800, 500],
params
)
tuner.tune(Test)
print(tuner.sort_tunes())
tuner.save_tunes('quick-trade-tunes.json') # save tunes as JSON
```
You can also set rules for arranging arguments for each strategy by using `_RULES_` and `kwargs` to access the values of the arguments:
```python
params = {
'strategy_3_sma':
[
dict(
plot=False,
slow=Choise([2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597]),
fast=Choise([2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597]),
mid=Choise([2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597]),
_RULES_='kwargs["slow"] > kwargs["mid"] > kwargs["fast"]'
)
],
}
```
## User's code example (backtest)
```python
from quick_trade import brokers
from quick_trade import trading_sys as qtr
from quick_trade.plots import *
import ccxt
from numpy import inf
client = brokers.TradingClient(ccxt.binance())
df = client.get_data_historical('BTC/USDT', '15m', 1000)
trader = qtr.ExampleStrategies('BTC/USDT', df=df, interval='15m')
trader.set_client(client)
trader.connect_graph(TraderGraph(make_trader_figure(height=731, width=1440, row_heights=[10, 5, 2])))
trader.strategy_2_sma(55, 21)
trader.backtest(deposit=1000, commission=0.075, bet=inf) # backtest on one pair
```
## Output plotly chart:
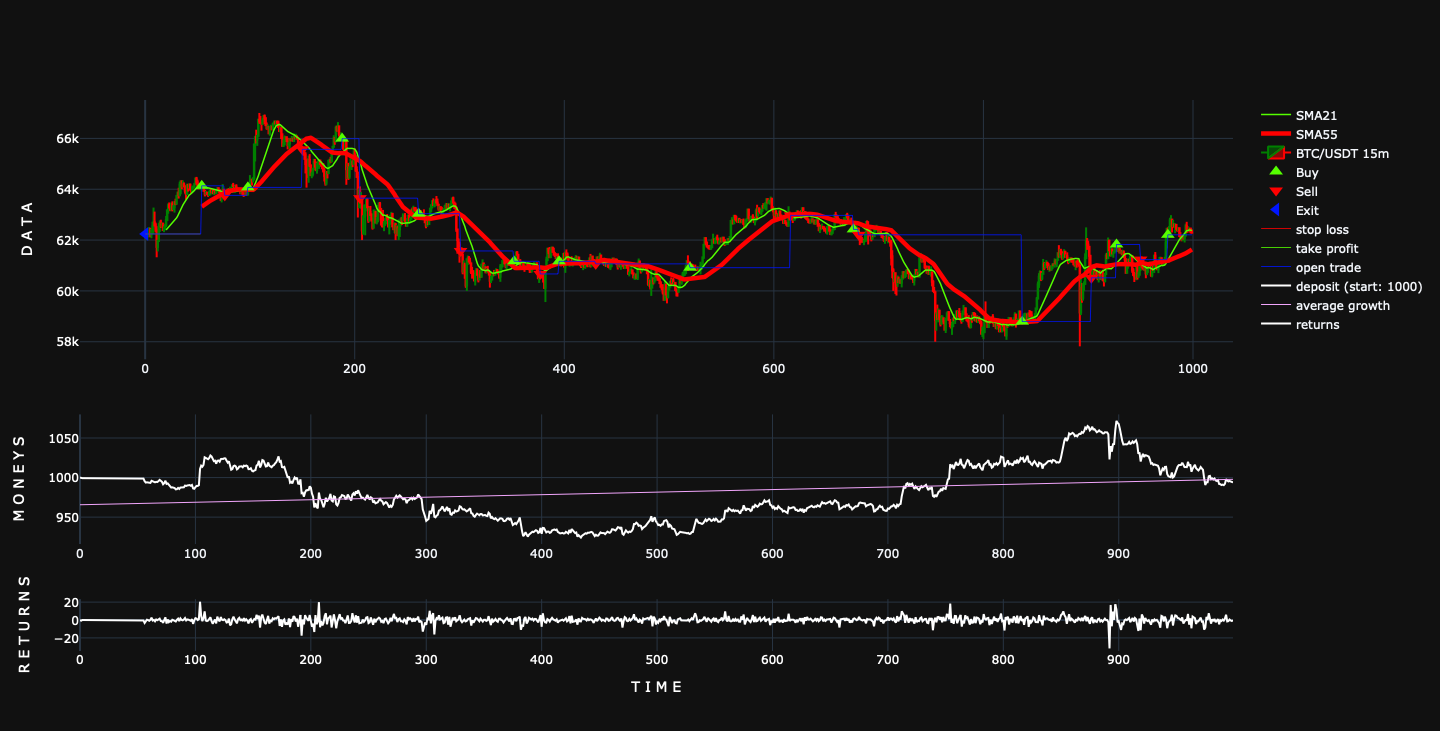
## Output print
```commandline
losses: 12
trades: 20
profits: 8
mean year percentage profit: 215.1878652911773%
winrate: 40.0%
mean deviation: 2.917382949881604%
Sharpe ratio: 0.02203412259055281
Sortino ratio: 0.02774402450236864
calmar ratio: 21.321078596349782
max drawdown: 10.092728860725552%
```
## Run strategy
Use the strategy on real moneys. YES, IT'S FULLY AUTOMATED!
```python
import datetime
from quick_trade.trading_sys import ExampleStrategies
from quick_trade.brokers import TradingClient
from quick_trade.plots import TraderGraph, make_figure
import ccxt
ticker = 'MATIC/USDT'
start_time = datetime.datetime(2021, # year
6, # month
24, # day
5, # hour
16, # minute
57) # second (Leave a few seconds to download data from the exchange)
class MyTrade(ExampleStrategies):
@strategy
def strategy(self):
self.strategy_supertrend(multiplier=2, length=1, plot=False)
self.convert_signal()
self.set_credit_leverages(1)
self.sl_tp_adder(10)
return self.returns
keys = {'apiKey': 'your api key',
'secret': 'your secret key'}
client = TradingClient(ccxt.binance(config=keys)) # or any other exchange
trader = MyTrade(ticker=ticker,
interval='1m',
df=client.get_data_historical(ticker, limit=10))
fig = make_trader_figure()
graph = TraderGraph(figure=fig)
trader.connect_graph(graph)
trader.set_client(client)
trader.realtime_trading(
strategy=trader.strategy,
start_time=start_time,
ticker=ticker,
limit=100,
wait_sl_tp_checking=5
)
```

## Additional Resources
Old documentation (V3 doc): https://vladkochetov007.github.io/quick_trade.github.io
# License
<a rel="license" href="http://creativecommons.org/licenses/by-nc-sa/4.0/"><img alt="Creative Commons License" style="border-width:0" src="https://i.creativecommons.org/l/by-nc-sa/4.0/88x31.png" /></a><br /><span xmlns:dct="http://purl.org/dc/terms/" property="dct:title">quick_trade</span> by <a xmlns:cc="http://creativecommons.org/ns#" href="https://github.com/VladKochetov007" property="cc:attributionName" rel="cc:attributionURL">Vladyslav Kochetov</a> is licensed under a <a rel="license" href="http://creativecommons.org/licenses/by-nc-sa/4.0/">Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License</a>.<br />Permissions beyond the scope of this license may be available at <a xmlns:cc="http://creativecommons.org/ns#" href="vladyslavdrrragonkoch@gmail.com" rel="cc:morePermissions">vladyslavdrrragonkoch@gmail.com</a>.
Raw data
{
"_id": null,
"home_page": null,
"name": "quick-trade",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": null,
"keywords": "technical-analysis, python3, trading, trading-bot, trading, binance-trading, ccxt",
"author": "Vlad Kochetov",
"author_email": "vladyslavdrrragonkoch@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/68/9d/b81d5f8e005ed9e6a22b000b5c2b8b04a905f173fe41e30c866fd8947ff8/quick_trade-8.0.0.tar.gz",
"platform": null,
"description": "# quick_trade\n[](https://vshymanskyy.github.io/StandWithUkraine)\n[](https://pepy.tech/project/quick-trade)\n[)](https://pepy.tech/project/quick-trade)\n\n\n\n\n```\nDependencies:\n \u251c\u2500\u2500ta (Bukosabino https://github.com/bukosabino/ta (by Dar\u00edo L\u00f3pez Padial))\n \u251c\u2500\u2500plotly (https://github.com/plotly/plotly.py)\n \u251c\u2500\u2500pandas (https://github.com/pandas-dev/pandas)\n \u251c\u2500\u2500numpy (https://github.com/numpy/numpy)\n \u251c\u2500\u2500tqdm (https://github.com/tqdm/tqdm)\n \u251c\u2500\u2500scikit-learn (https://github.com/scikit-learn/scikit-learn)\n \u2514\u2500\u2500ccxt (https://github.com/ccxt/ccxt)\n```\n* **Documentation:** \ud83d\udea7 https://quick-trade.github.io/quick_trade/#/ \ud83d\udea7\n* **Twitter:** [@quick_trade_tw](https://twitter.com/quick_trade_tw)\n* **Discord**: [quick_trade](https://discord.gg/SkRg9mzuB5)\n\n## Installation:\n\nQuick install:\n\n```commandline\n$ pip3 install quick-trade\n```\n\nFor development:\n\n```commandline\n$ git clone https://github.com/quick-trade/quick_trade.git\n$ pip3 install -r quick_trade/requirements.txt\n$ cd quick_trade\n$ python3 setup.py install\n$ cd ..\n```\n\n## Customize your strategy!\n\n```python\nfrom quick_trade.plots import TraderGraph, make_trader_figure\nimport ccxt\nfrom quick_trade import strategy, TradingClient, Trader\nfrom quick_trade.utils import TradeSide\n\n\nclass MyTrader(qtr.Trader):\n @strategy\n def strategy_sell_and_hold(self):\n ret = []\n for i in self.df['Close'].values:\n ret.append(TradeSide.SELL)\n self.returns = ret\n self.set_credit_leverages(2) # if you want to use a leverage\n self.set_open_stop_and_take(stop)\n # or... set a stop loss with only one line of code\n return ret\n\n\nclient = TradingClient(ccxt.binance())\ndf = client.get_data_historical(\"BTC/USDT\")\ntrader = MyTrader(\"BTC/USDT\", df=df)\ntrader.connect_graph(TraderGraph(make_trader_figure()))\ntrader.set_client(client)\ntrader.strategy_sell_and_hold()\ntrader.backtest()\n```\n\n## Find the best strategy!\n\n```python\nimport quick_trade as qtr\nimport ccxt\nfrom quick_trade.tuner import *\nfrom quick_trade import TradingClient\n\n\nclass Test(qtr.ExampleStrategies):\n @strategy\n def strategy_supertrend1(self, plot: bool = False, *st_args, **st_kwargs):\n self.strategy_supertrend(plot=plot, *st_args, **st_kwargs)\n self.convert_signal() # only long trades\n return self.returns\n\n @strategy\n def macd(self, histogram=False, **kwargs):\n if not histogram:\n self.strategy_macd(**kwargs)\n else:\n self.strategy_macd_histogram_diff(**kwargs)\n self.convert_signal()\n return self.returns\n\n @strategy\n def psar(self, **kwargs):\n self.strategy_parabolic_SAR(plot=False, **kwargs)\n self.convert_signal()\n return self.returns\n\n\nparams = {\n 'strategy_supertrend1':\n [\n {\n 'multiplier': Linspace(0.5, 22, 5)\n }\n ],\n 'macd':\n [\n {\n 'slow': Linspace(10, 100, 3),\n 'fast': Linspace(3, 60, 3),\n 'histogram': Choise([False, True])\n }\n ],\n 'psar':\n [\n {\n 'step': 0.01,\n 'max_step': 0.1\n },\n {\n 'step': 0.02,\n 'max_step': 0.2\n }\n ]\n\n}\n\ntuner = QuickTradeTuner(\n TradingClient(ccxt.binance()),\n ['BTC/USDT', 'OMG/USDT', 'XRP/USDT'],\n ['15m', '5m'],\n [1000, 700, 800, 500],\n params\n)\n\ntuner.tune(Test)\nprint(tuner.sort_tunes())\ntuner.save_tunes('quick-trade-tunes.json') # save tunes as JSON\n\n```\n\nYou can also set rules for arranging arguments for each strategy by using `_RULES_` and `kwargs` to access the values of the arguments:\n\n```python\nparams = {\n 'strategy_3_sma':\n [\n dict(\n plot=False,\n slow=Choise([2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597]),\n fast=Choise([2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597]),\n mid=Choise([2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597]),\n _RULES_='kwargs[\"slow\"] > kwargs[\"mid\"] > kwargs[\"fast\"]'\n )\n ],\n}\n```\n\n## User's code example (backtest)\n\n```python\nfrom quick_trade import brokers\nfrom quick_trade import trading_sys as qtr\nfrom quick_trade.plots import *\nimport ccxt\nfrom numpy import inf\n\n\nclient = brokers.TradingClient(ccxt.binance())\ndf = client.get_data_historical('BTC/USDT', '15m', 1000)\ntrader = qtr.ExampleStrategies('BTC/USDT', df=df, interval='15m')\ntrader.set_client(client)\ntrader.connect_graph(TraderGraph(make_trader_figure(height=731, width=1440, row_heights=[10, 5, 2])))\ntrader.strategy_2_sma(55, 21)\ntrader.backtest(deposit=1000, commission=0.075, bet=inf) # backtest on one pair\n```\n\n## Output plotly chart:\n\n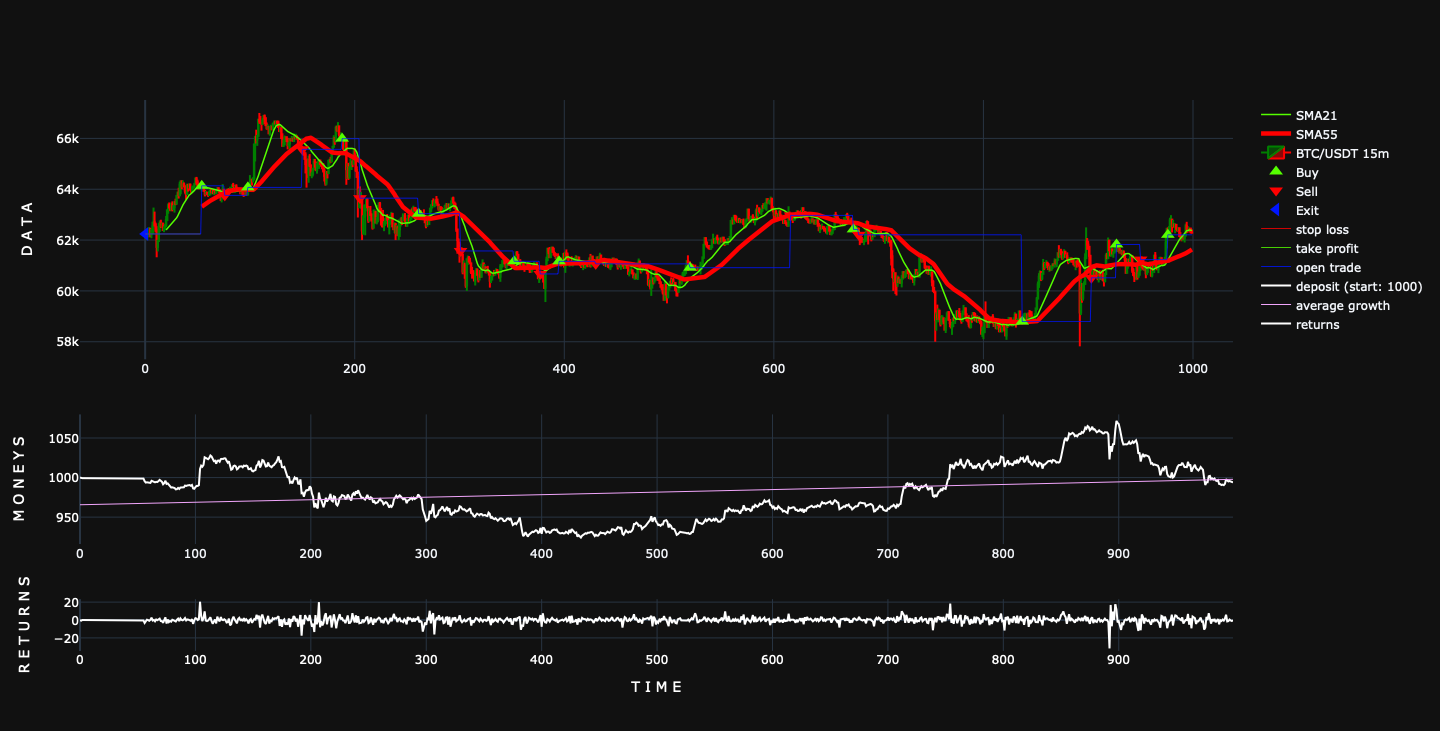\n\n## Output print\n\n```commandline\nlosses: 12\ntrades: 20\nprofits: 8\nmean year percentage profit: 215.1878652911773%\nwinrate: 40.0%\nmean deviation: 2.917382949881604%\nSharpe ratio: 0.02203412259055281\nSortino ratio: 0.02774402450236864\ncalmar ratio: 21.321078596349782\nmax drawdown: 10.092728860725552%\n```\n\n## Run strategy\n\nUse the strategy on real moneys. YES, IT'S FULLY AUTOMATED!\n\n```python\nimport datetime\nfrom quick_trade.trading_sys import ExampleStrategies\nfrom quick_trade.brokers import TradingClient\nfrom quick_trade.plots import TraderGraph, make_figure\nimport ccxt\n\nticker = 'MATIC/USDT'\n\nstart_time = datetime.datetime(2021, # year\n 6, # month\n 24, # day\n\n 5, # hour\n 16, # minute\n 57) # second (Leave a few seconds to download data from the exchange)\n\n\nclass MyTrade(ExampleStrategies):\n @strategy\n def strategy(self):\n self.strategy_supertrend(multiplier=2, length=1, plot=False)\n self.convert_signal()\n self.set_credit_leverages(1)\n self.sl_tp_adder(10)\n return self.returns\n\n\nkeys = {'apiKey': 'your api key',\n 'secret': 'your secret key'}\nclient = TradingClient(ccxt.binance(config=keys)) # or any other exchange\n\ntrader = MyTrade(ticker=ticker,\n interval='1m',\n df=client.get_data_historical(ticker, limit=10))\nfig = make_trader_figure()\ngraph = TraderGraph(figure=fig)\ntrader.connect_graph(graph)\ntrader.set_client(client)\n\ntrader.realtime_trading(\n strategy=trader.strategy,\n start_time=start_time,\n ticker=ticker,\n limit=100,\n wait_sl_tp_checking=5\n)\n\n```\n\n\n\n## Additional Resources\n\nOld documentation (V3 doc): https://vladkochetov007.github.io/quick_trade.github.io\n\n# License\n\n<a rel=\"license\" href=\"http://creativecommons.org/licenses/by-nc-sa/4.0/\"><img alt=\"Creative Commons License\" style=\"border-width:0\" src=\"https://i.creativecommons.org/l/by-nc-sa/4.0/88x31.png\" /></a><br /><span xmlns:dct=\"http://purl.org/dc/terms/\" property=\"dct:title\">quick_trade</span> by <a xmlns:cc=\"http://creativecommons.org/ns#\" href=\"https://github.com/VladKochetov007\" property=\"cc:attributionName\" rel=\"cc:attributionURL\">Vladyslav Kochetov</a> is licensed under a <a rel=\"license\" href=\"http://creativecommons.org/licenses/by-nc-sa/4.0/\">Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License</a>.<br />Permissions beyond the scope of this license may be available at <a xmlns:cc=\"http://creativecommons.org/ns#\" href=\"vladyslavdrrragonkoch@gmail.com\" rel=\"cc:morePermissions\">vladyslavdrrragonkoch@gmail.com</a>.\n",
"bugtrack_url": null,
"license": "cc-by-sa-4.0",
"summary": "Library for easy management and customization of algorithmic trading.",
"version": "8.0.0",
"project_urls": {
"Bug Tracker": "https://github.com/quick-trade/quick_trade/issues",
"Documentation": "https://quick-trade.github.io/quick_trade/#/",
"Download": "https://github.com/quick-trade/quick_trade/archive/8.0.0.tar.gz",
"Source": "https://github.com/quick-trade/quick_trade",
"Twitter": "https://twitter.com/quick_trade_tw"
},
"split_keywords": [
"technical-analysis",
" python3",
" trading",
" trading-bot",
" trading",
" binance-trading",
" ccxt"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "689db81d5f8e005ed9e6a22b000b5c2b8b04a905f173fe41e30c866fd8947ff8",
"md5": "456b1695dfb0ea15cdb3b82f93fc6ff3",
"sha256": "3d78c762e40c631d9b62d2c3c579ba8d80b32a2d772ab58bb2cea9c89a705570"
},
"downloads": -1,
"filename": "quick_trade-8.0.0.tar.gz",
"has_sig": false,
"md5_digest": "456b1695dfb0ea15cdb3b82f93fc6ff3",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 39458,
"upload_time": "2024-12-06T00:52:36",
"upload_time_iso_8601": "2024-12-06T00:52:36.658107Z",
"url": "https://files.pythonhosted.org/packages/68/9d/b81d5f8e005ed9e6a22b000b5c2b8b04a905f173fe41e30c866fd8947ff8/quick_trade-8.0.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-12-06 00:52:36",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "quick-trade",
"github_project": "quick_trade",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "numpy",
"specs": [
[
"==",
"1.26.1"
]
]
},
{
"name": "plotly",
"specs": [
[
"==",
"5.15.0"
]
]
},
{
"name": "pandas",
"specs": [
[
"==",
"2.0.2"
]
]
},
{
"name": "ta",
"specs": [
[
"==",
"0.10.2"
]
]
},
{
"name": "ccxt",
"specs": [
[
"==",
"4.1.19"
]
]
},
{
"name": "tqdm",
"specs": [
[
"==",
"4.65.0"
]
]
},
{
"name": "scikit-learn",
"specs": []
}
],
"lcname": "quick-trade"
}