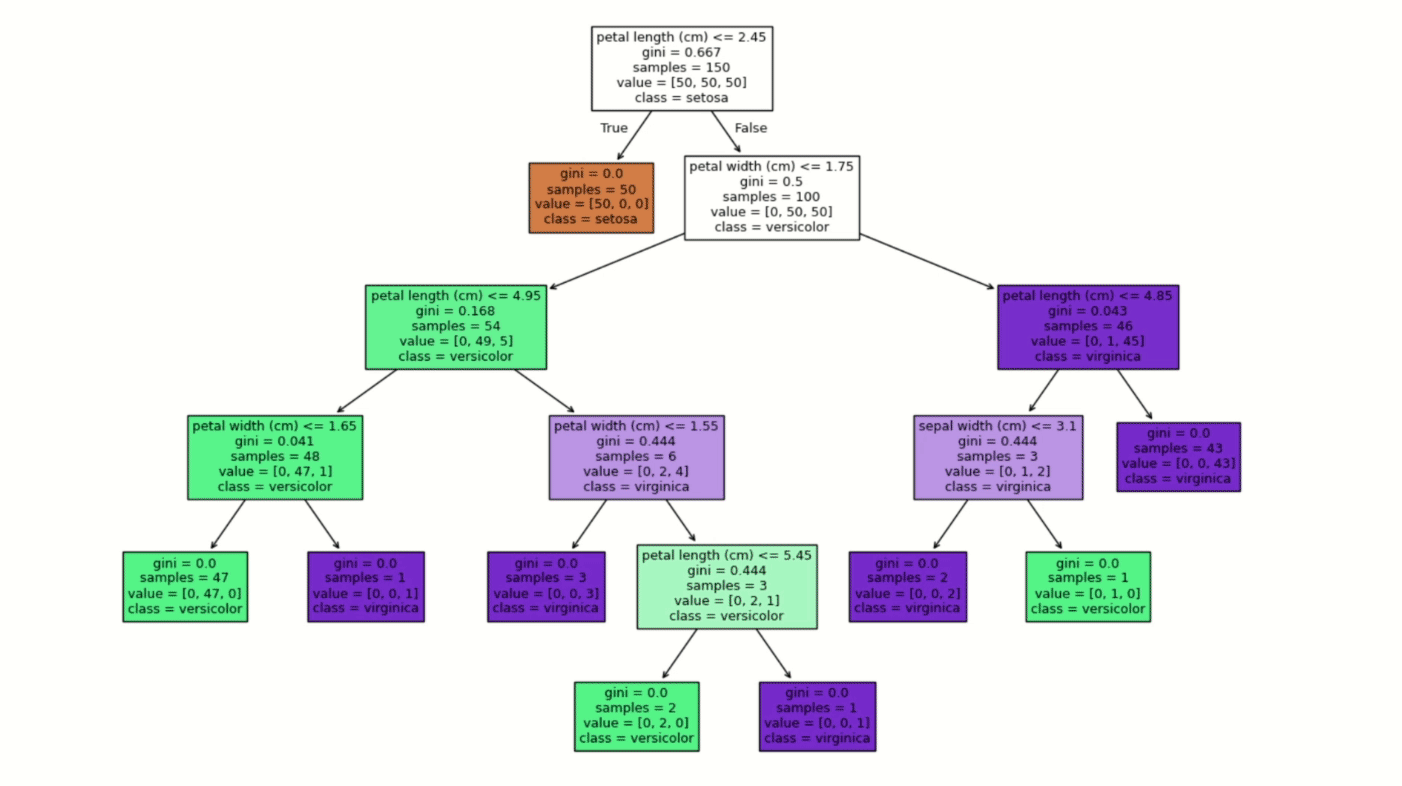
# `supertree` - Interactive Decision Tree Visualization
`supertree` is a Python package designed to visualize decision trees in an **interactive** and user-friendly way within Jupyter Notebooks, Jupyter Lab, Google Colab, and any other notebooks that support HTML rendering. With this tool, you can not only display decision trees, but also interact with them directly within your notebook environment. Key features include:
- ability to zoom and pan through large trees,
- collapse and expand selected nodes,
- explore the structure of the tree in an intuitive and visually appealing manner.
## Features
<div style="overflow: hidden;">
<table style="table-layout: fixed; width: 100%; position: absolute;'">
<tr>
<td><img src="https://github.com/mljar/supertree/blob/main/media/videos/2_regression_details-ezgif.com-video-to-gif-converter.gif" alt="Gif1" width="375"/><br/>See all the details</td>
<td><img src="https://github.com/mljar/supertree/blob/main/media/videos/1_supertree_zoom_an_reset-ezgif.com-video-to-gif-converter.gif" alt="Gif2" width="375"/><br/>Zoom</td>
</tr>
</table>
<table style="table-layout: fixed; width: 100%; position: absolute;'">
<tr>
<td><img src="https://github.com/mljar/supertree/blob/main/media/videos/4_fullscreen-ezgif.com-video-to-gif-converter.gif" alt="Gif3" width="375"/><br/>Fullscreen in Jupyter</td>
<td><img src="https://github.com/mljar/supertree/blob/main/media/videos/6_change_depth_dynamicaly-ezgif.com-video-to-gif-converter.gif" alt="Gif4" width="375"/><br/>Depth change</td>
</tr>
</table>
<table style="table-layout: fixed; width: 100%; position: absolute;'">
<tr>
<td><img src="https://github.com/mljar/supertree/blob/main/media/videos/change_palette.gif" alt="Gif5" width="375"/><br/>Color change</td>
<td><img src="https://github.com/mljar/supertree/blob/main/media/videos/switch_tree_in_forest.gif" alt="Gif6" width="375"/><br/>Navigate in forest</td>
</tr>
</table>
<table style="table-layout: fixed; width: 100%; position: absolute;'">
<tr>
<td><img src="https://github.com/mljar/supertree/blob/main/media/videos/sample_path.gif" alt="Gif7" width="375"/><br/>Show specific sample path</td>
<td><img src="https://github.com/mljar/supertree/blob/main/media/videos/save_svg.gif" alt="Gif8" width="375"/><br/>Save tree to svg</td>
</tr>
</table>
<table style="table-layout: fixed; width: 100%; position: absolute;'">
<tr>
<td><img src="https://github.com/mljar/supertree/blob/main/media/videos/3_amount_of_sample_visualized-ezgif.com-video-to-gif-converter.gif" alt="Gif11" width="375"/><br/>Links sample visualization</td>
<td><img src="https://github.com/mljar/supertree/blob/main/media/videos/7_path_to_leaf-ezgif.com-video-to-gif-converter.gif" alt="Gif12" width="375"/><br/>Showing the path to the leaf</td>
</tr>
</table>
</div>
Check this features in example directory :)
## Examples
### Decision Tree classifier on iris data
<a target="_blank" href="https://colab.research.google.com/drive/1f2Xu8CwbXaT33hvh-ze0JK3sBSpXBt5T?usp=sharing">
<img src="https://colab.research.google.com/assets/colab-badge.svg" alt="Open In Colab"/>
</a>
```python
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
from supertree import SuperTree # <- import supertree :)
# Load the iris dataset
iris = load_iris()
X, y = iris.data, iris.target
# Train model
model = DecisionTreeClassifier()
model.fit(X, y)
# Initialize supertree
super_tree = SuperTree(model, X, y, iris.feature_names, iris.target_names)
# show tree in your notebook
super_tree.show_tree()
```
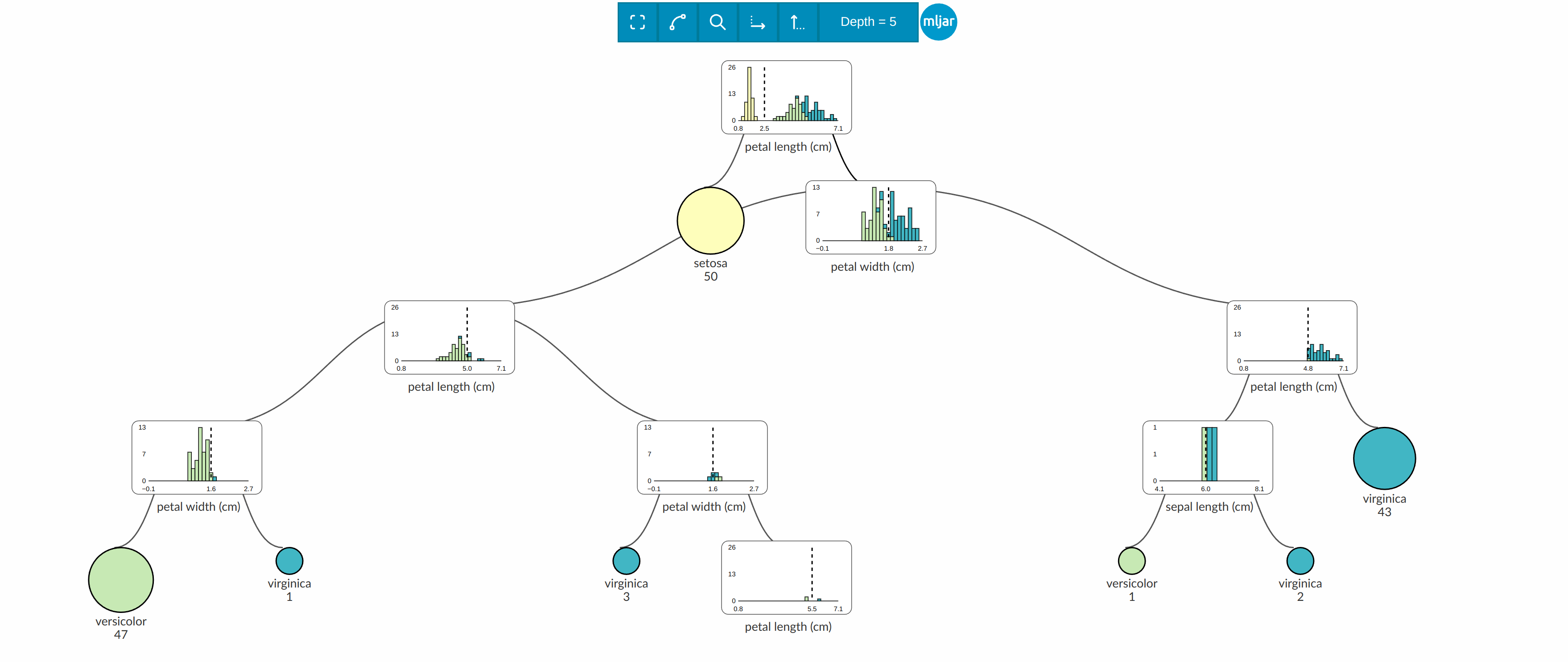
### Random Forest Regressor Example
<a target="_blank" href="https://colab.research.google.com/drive/1nR7GlrIKcMQYdnMm_duY7a6vscyqTCMj?usp=sharing">
<img src="https://colab.research.google.com/assets/colab-badge.svg" alt="Open In Colab"/>
</a>
```python
from sklearn.ensemble import RandomForestRegressor
from sklearn.datasets import load_diabetes
from supertree import SuperTree # <- import supertree :)
# Load the diabetes dataset
diabetes = load_diabetes()
X = diabetes.data
y = diabetes.target
# Train model
model = RandomForestRegressor(n_estimators=100, max_depth=3, random_state=42)
model.fit(X, y)
# Initialize supertree
super_tree = SuperTree(model,X, y)
# show tree with index 2 in your notebook
super_tree.show_tree(2)
```
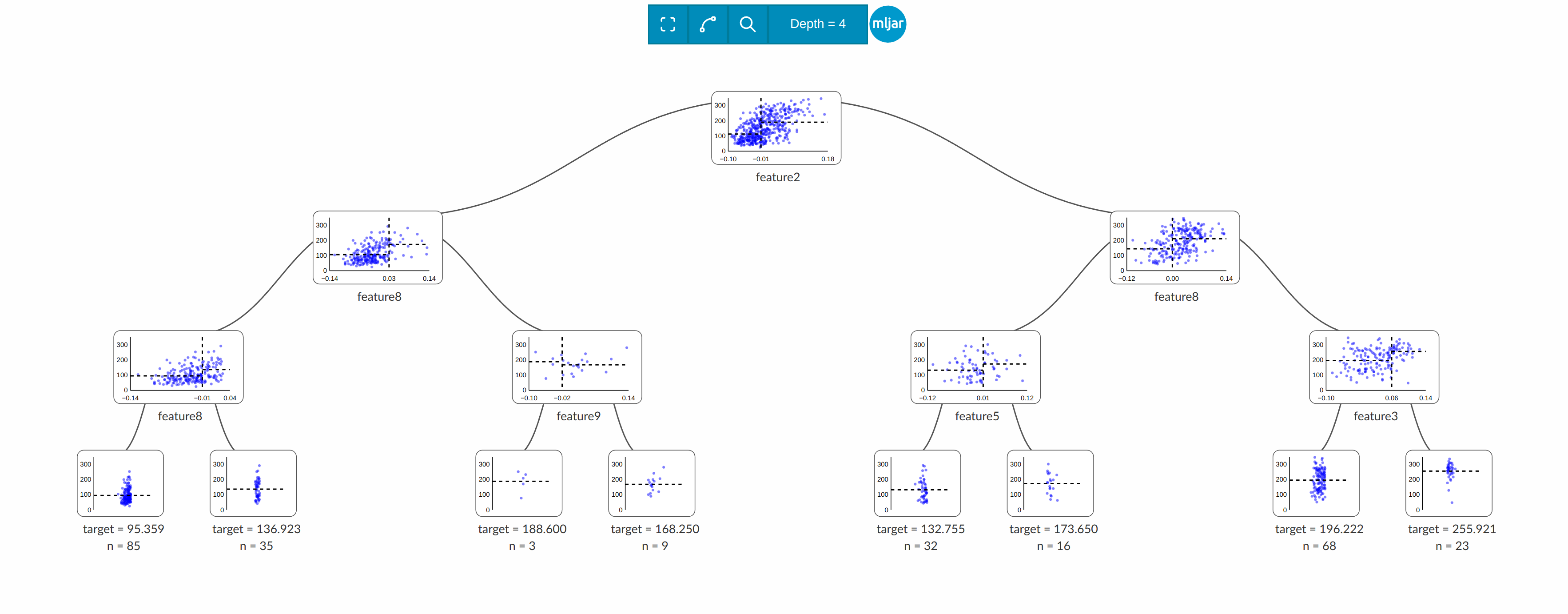
There are more code snippets in the [examples](examples) directory.
## Instalation
You can install SuperTree package using pip:
```
pip install supertree
```
Conda support coming soon.
## Supported Libraries
- **scikit-learn** (`sklearn`)
- **LightGBM**
- **XGBoost**
- **ONNX**:
### Supported Algorithms
The package is compatible with a wide range of classifiers and regressors from these libraries, specifically:
#### Scikit-learn
- `DecisionTreeClassifier`
- `ExtraTreeClassifier`
- `ExtraTreesClassifier`
- `RandomForestClassifier`
- `GradientBoostingClassifier`
- `HistGradientBoostingClassifier`
- `DecisionTreeRegressor`
- `ExtraTreeRegressor`
- `ExtraTreesRegressor`
- `RandomForestRegressor`
- `GradientBoostingRegressor`
- `HistGradientBoostingRegressor`
#### LightGBM
- `LGBMClassifier`
- `LGBMRegressor`
- `Booster`
#### XGBoost
- `XGBClassifier`
- `XGBRFClassifier`
- `XGBRegressor`
- `XGBRFRegressor`
- `Booster`
If we do not support the model you want to use, please let us know.
## Articles
- [Visualize decision tree from scikit-learn package](https://mljar.com/blog/visualize-decision-tree/)
- [4 ways to vizualize decision tree from LightGBM](https://mljar.com/blog/visualize-lightgbm-tree/)
- [How to visualize decision tree from Xgboost](https://mljar.com/blog/visualize-xgboost-tree/)
## Support
If you encounter any issues, find a bug, or have a feature request, we would love to hear from you! Please don't hesitate to reach out to us at supertree/issues. We are committed to improving this package and appreciate any feedback or suggestions you may have.
## License
`supertree` is a commercial software with two licenses available:
- AGPL-3.0 license
- Commercial license with support and maintenance included. Pricing website https://mljar.com/supertree/ License [supertree-commercial-license.pdf](supertree-commercial-license.pdf).
Raw data
{
"_id": null,
"home_page": "https://github.com/mljar/supertree",
"name": "supertree",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.7.1",
"maintainer_email": null,
"keywords": "visualization, decision-tree, machine-learning, data-analysis, data-mining, classification, regression, mljar",
"author": "MLJAR Sp. z o.o.",
"author_email": "contact@mljar.com",
"download_url": "https://files.pythonhosted.org/packages/89/cd/c100f5b9f2845cb5fb58941e5e34157d750c1e5bc02c5b5dd0bbb75d3684/supertree-0.5.4.tar.gz",
"platform": null,
"description": "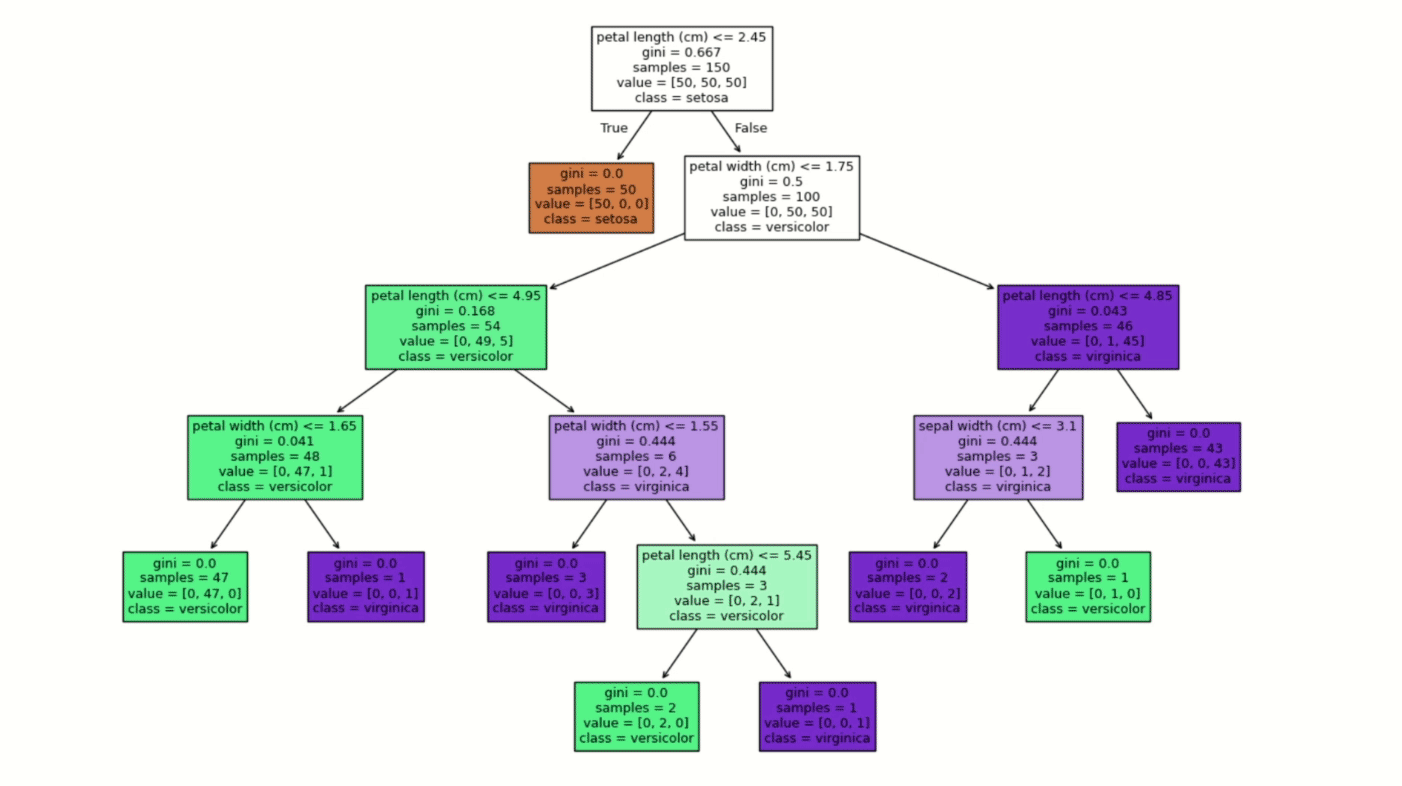\n\n# `supertree` - Interactive Decision Tree Visualization\n\n`supertree` is a Python package designed to visualize decision trees in an **interactive** and user-friendly way within Jupyter Notebooks, Jupyter Lab, Google Colab, and any other notebooks that support HTML rendering. With this tool, you can not only display decision trees, but also interact with them directly within your notebook environment. Key features include:\n- ability to zoom and pan through large trees,\n- collapse and expand selected nodes, \n- explore the structure of the tree in an intuitive and visually appealing manner.\n\n## Features\n\n<div style=\"overflow: hidden;\">\n <table style=\"table-layout: fixed; width: 100%; position: absolute;'\">\n <tr>\n <td><img src=\"https://github.com/mljar/supertree/blob/main/media/videos/2_regression_details-ezgif.com-video-to-gif-converter.gif\" alt=\"Gif1\" width=\"375\"/><br/>See all the details</td>\n <td><img src=\"https://github.com/mljar/supertree/blob/main/media/videos/1_supertree_zoom_an_reset-ezgif.com-video-to-gif-converter.gif\" alt=\"Gif2\" width=\"375\"/><br/>Zoom</td>\n </tr>\n </table>\n <table style=\"table-layout: fixed; width: 100%; position: absolute;'\">\n <tr>\n <td><img src=\"https://github.com/mljar/supertree/blob/main/media/videos/4_fullscreen-ezgif.com-video-to-gif-converter.gif\" alt=\"Gif3\" width=\"375\"/><br/>Fullscreen in Jupyter</td>\n <td><img src=\"https://github.com/mljar/supertree/blob/main/media/videos/6_change_depth_dynamicaly-ezgif.com-video-to-gif-converter.gif\" alt=\"Gif4\" width=\"375\"/><br/>Depth change</td>\n </tr>\n </table>\n <table style=\"table-layout: fixed; width: 100%; position: absolute;'\">\n <tr>\n <td><img src=\"https://github.com/mljar/supertree/blob/main/media/videos/change_palette.gif\" alt=\"Gif5\" width=\"375\"/><br/>Color change</td>\n <td><img src=\"https://github.com/mljar/supertree/blob/main/media/videos/switch_tree_in_forest.gif\" alt=\"Gif6\" width=\"375\"/><br/>Navigate in forest</td>\n </tr>\n </table>\n <table style=\"table-layout: fixed; width: 100%; position: absolute;'\">\n <tr>\n <td><img src=\"https://github.com/mljar/supertree/blob/main/media/videos/sample_path.gif\" alt=\"Gif7\" width=\"375\"/><br/>Show specific sample path</td>\n <td><img src=\"https://github.com/mljar/supertree/blob/main/media/videos/save_svg.gif\" alt=\"Gif8\" width=\"375\"/><br/>Save tree to svg</td>\n </tr>\n </table>\n <table style=\"table-layout: fixed; width: 100%; position: absolute;'\">\n <tr>\n <td><img src=\"https://github.com/mljar/supertree/blob/main/media/videos/3_amount_of_sample_visualized-ezgif.com-video-to-gif-converter.gif\" alt=\"Gif11\" width=\"375\"/><br/>Links sample visualization</td>\n <td><img src=\"https://github.com/mljar/supertree/blob/main/media/videos/7_path_to_leaf-ezgif.com-video-to-gif-converter.gif\" alt=\"Gif12\" width=\"375\"/><br/>Showing the path to the leaf</td>\n </tr>\n </table>\n</div>\n\nCheck this features in example directory :)\n\n## Examples\n\n### Decision Tree classifier on iris data \n\n<a target=\"_blank\" href=\"https://colab.research.google.com/drive/1f2Xu8CwbXaT33hvh-ze0JK3sBSpXBt5T?usp=sharing\">\n <img src=\"https://colab.research.google.com/assets/colab-badge.svg\" alt=\"Open In Colab\"/>\n</a>\n\n```python\nfrom sklearn.tree import DecisionTreeClassifier\nfrom sklearn.datasets import load_iris\nfrom supertree import SuperTree # <- import supertree :)\n\n# Load the iris dataset\niris = load_iris()\nX, y = iris.data, iris.target\n\n# Train model\nmodel = DecisionTreeClassifier()\nmodel.fit(X, y)\n\n# Initialize supertree\nsuper_tree = SuperTree(model, X, y, iris.feature_names, iris.target_names)\n\n# show tree in your notebook\nsuper_tree.show_tree()\n```\n\n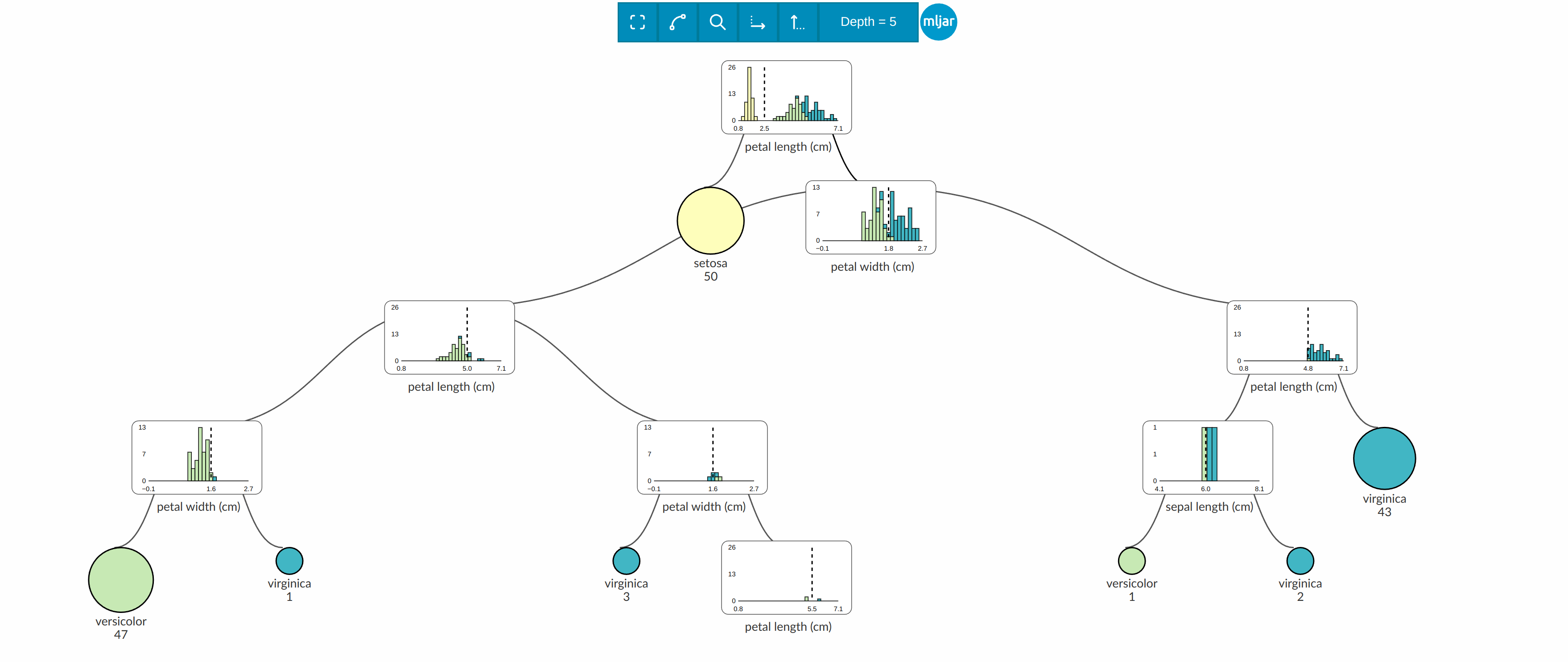\n\n### Random Forest Regressor Example\n\n<a target=\"_blank\" href=\"https://colab.research.google.com/drive/1nR7GlrIKcMQYdnMm_duY7a6vscyqTCMj?usp=sharing\">\n <img src=\"https://colab.research.google.com/assets/colab-badge.svg\" alt=\"Open In Colab\"/>\n</a>\n\n```python\nfrom sklearn.ensemble import RandomForestRegressor\nfrom sklearn.datasets import load_diabetes\nfrom supertree import SuperTree # <- import supertree :)\n\n# Load the diabetes dataset\ndiabetes = load_diabetes()\nX = diabetes.data\ny = diabetes.target\n\n# Train model\nmodel = RandomForestRegressor(n_estimators=100, max_depth=3, random_state=42)\nmodel.fit(X, y)\n\n# Initialize supertree\nsuper_tree = SuperTree(model,X, y)\n# show tree with index 2 in your notebook\nsuper_tree.show_tree(2)\n```\n\n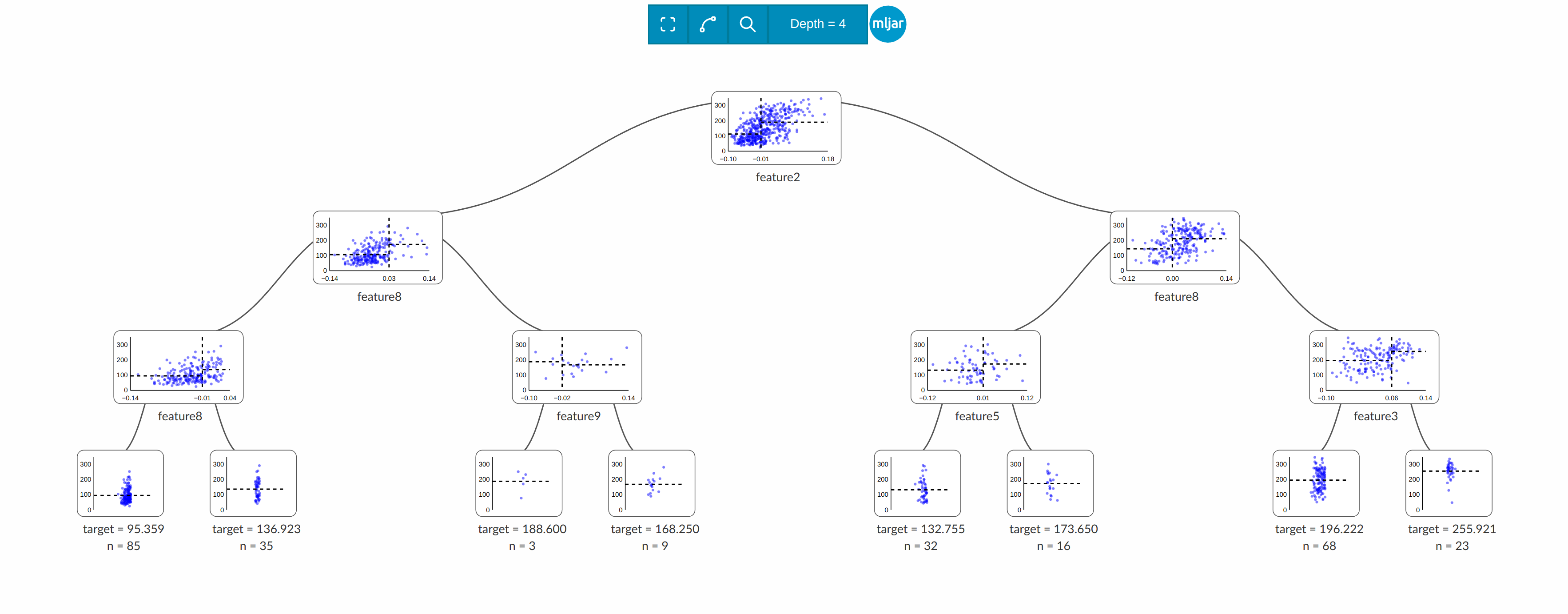\n\nThere are more code snippets in the [examples](examples) directory.\n\n\n\n## Instalation\nYou can install SuperTree package using pip:\n\n```\npip install supertree\n```\n\nConda support coming soon.\n\n## Supported Libraries\n\n- **scikit-learn** (`sklearn`)\n- **LightGBM**\n- **XGBoost**\n- **ONNX**:\n\n### Supported Algorithms\n\nThe package is compatible with a wide range of classifiers and regressors from these libraries, specifically:\n\n#### Scikit-learn\n- `DecisionTreeClassifier`\n- `ExtraTreeClassifier`\n- `ExtraTreesClassifier`\n- `RandomForestClassifier`\n- `GradientBoostingClassifier`\n- `HistGradientBoostingClassifier`\n- `DecisionTreeRegressor`\n- `ExtraTreeRegressor`\n- `ExtraTreesRegressor`\n- `RandomForestRegressor`\n- `GradientBoostingRegressor`\n- `HistGradientBoostingRegressor`\n\n#### LightGBM\n- `LGBMClassifier`\n- `LGBMRegressor`\n- `Booster`\n\n#### XGBoost\n- `XGBClassifier`\n- `XGBRFClassifier`\n- `XGBRegressor`\n- `XGBRFRegressor`\n- `Booster`\n\nIf we do not support the model you want to use, please let us know.\n\n## Articles\n\n- [Visualize decision tree from scikit-learn package](https://mljar.com/blog/visualize-decision-tree/)\n- [4 ways to vizualize decision tree from LightGBM](https://mljar.com/blog/visualize-lightgbm-tree/)\n- [How to visualize decision tree from Xgboost](https://mljar.com/blog/visualize-xgboost-tree/)\n\n\n## Support\n\nIf you encounter any issues, find a bug, or have a feature request, we would love to hear from you! Please don't hesitate to reach out to us at supertree/issues. We are committed to improving this package and appreciate any feedback or suggestions you may have.\n\n## License \n\n`supertree` is a commercial software with two licenses available:\n\n- AGPL-3.0 license\n- Commercial license with support and maintenance included. Pricing website https://mljar.com/supertree/ License [supertree-commercial-license.pdf](supertree-commercial-license.pdf).\n\n",
"bugtrack_url": null,
"license": "LICENSE.txt",
"summary": "Visualize decision tree in Python",
"version": "0.5.4",
"project_urls": {
"Homepage": "https://github.com/mljar/supertree"
},
"split_keywords": [
"visualization",
" decision-tree",
" machine-learning",
" data-analysis",
" data-mining",
" classification",
" regression",
" mljar"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "89cdc100f5b9f2845cb5fb58941e5e34157d750c1e5bc02c5b5dd0bbb75d3684",
"md5": "196845561bc7c983a9e9a9c54e392cde",
"sha256": "798450bcfd61c5010e3c33b6c1076e6005480b4a9b971711e7290bb0807f717f"
},
"downloads": -1,
"filename": "supertree-0.5.4.tar.gz",
"has_sig": false,
"md5_digest": "196845561bc7c983a9e9a9c54e392cde",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7.1",
"size": 321421,
"upload_time": "2024-11-20T14:57:26",
"upload_time_iso_8601": "2024-11-20T14:57:26.439099Z",
"url": "https://files.pythonhosted.org/packages/89/cd/c100f5b9f2845cb5fb58941e5e34157d750c1e5bc02c5b5dd0bbb75d3684/supertree-0.5.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-11-20 14:57:26",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "mljar",
"github_project": "supertree",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [
{
"name": "pandas",
"specs": []
},
{
"name": "numpy",
"specs": []
},
{
"name": "ipython",
"specs": []
},
{
"name": "ipywidgets",
"specs": []
}
],
"lcname": "supertree"
}