# tbparse
[](https://pypi.org/project/tbparse/)
[](https://pypi.org/project/tbparse/)
[](https://pepy.tech/project/tbparse)
[](https://github.com/j3soon/tbparse/blob/master/LICENSE)
[](https://github.com/j3soon/tbparse/actions/workflows/test-with-tox.yaml)
[](https://github.com/j3soon/tbparse/actions/workflows/publish-to-pypi.yaml)
[](https://tbparse.readthedocs.io/en/latest)
[](https://codecov.io/gh/j3soon/tbparse)
A simple yet powerful tensorboard event log parser/reader.
* Supports parsing tensorboard event [scalars][parsing-scalars], [tensors][parsing-tensors], [histograms][parsing-histograms], [images][parsing-images], [audio][parsing-audio], [hparams][parsing-hparams], and [text][parsing-text].
* Supports event generated by
[PyTorch](https://pytorch.org/docs/stable/tensorboard.html), [Tensorboard/Keras](https://www.tensorflow.org/tensorboard), and [TensorboardX](https://github.com/lanpa/tensorboardX), with their respective usage examples documented in detail.
* Allows parsing multiple tensorboard event files in a hierarchical directory structure.
* Provides plotting examples for each type of events.
* Stores the data in [pandas.DataFrame](https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.html) to allow advanced filtering.
* Both the documentation and code have high test coverage rate.
* Follows [PEP 484](https://www.python.org/dev/peps/pep-0484/) with full type hints.
Installation:
```sh
pip install tensorflow # or tensorflow-cpu
pip install -U tbparse # requires Python >= 3.7
```
**Note**: If you don't want to install TensorFlow, see [Installing without TensorFlow](https://tbparse.readthedocs.io/en/latest/pages/installation.html#installing-without-tensorflow).
We suggest using an additional virtual environment for parsing and plotting the tensorboard events. So no worries if your training code uses Python 3.6 or older versions.
Reading one or more event files with tbparse only requires 5 lines of code:
```py
from tbparse import SummaryReader
log_dir = "<PATH_TO_EVENT_FILE_OR_DIRECTORY>"
reader = SummaryReader(log_dir)
df = reader.scalars
print(df)
```
For beginners, start from the page: [Parsing Scalars][parsing-scalars].
## Gallery
| Event Type | Tensorboard Dashboard | tbparse |
|----------------------------------|----------------------------------------------|-----------------------------------------|
| [Scalars][parsing-scalars] | 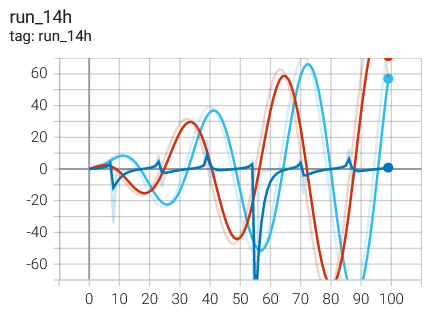 |  |
| [Tensors][parsing-tensors] | 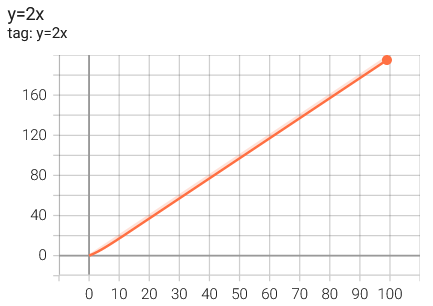 |  |
| [Histograms][parsing-histograms] | 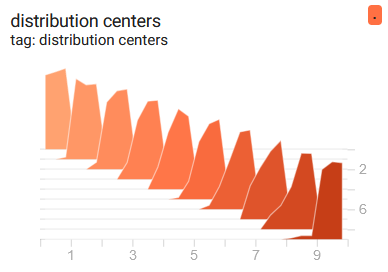 |  |
| [Images][parsing-images] | 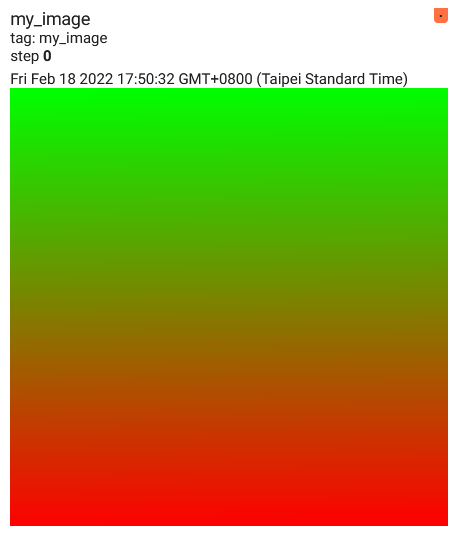 | 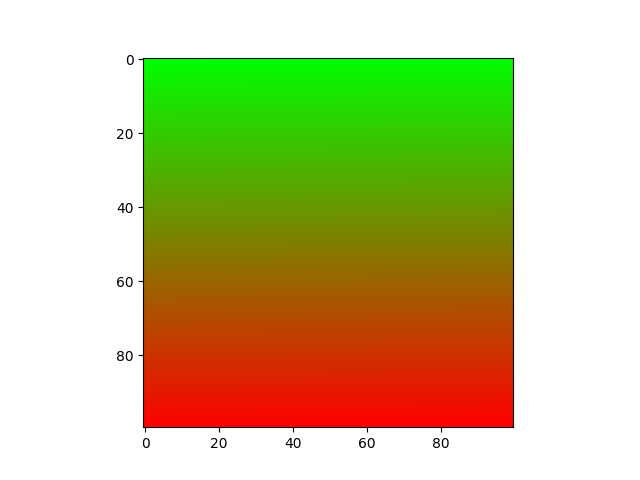 |
| [Audio][parsing-audio] | 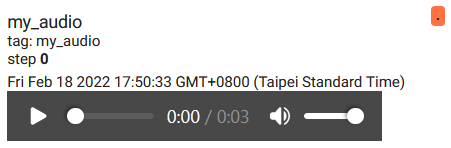 |  |
| [HParams][parsing-hparams] |  | 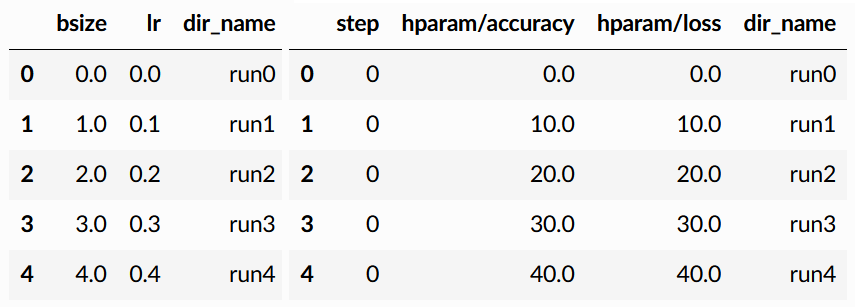 |
| [Text][parsing-text] |  |  |
[parsing-scalars]: https://tbparse.readthedocs.io/en/latest/pages/parsing-scalars.html
[parsing-tensors]: https://tbparse.readthedocs.io/en/latest/pages/parsing-tensors.html
[parsing-histograms]: https://tbparse.readthedocs.io/en/latest/pages/parsing-histograms.html
[parsing-images]: https://tbparse.readthedocs.io/en/latest/pages/parsing-images.html
[parsing-audio]: https://tbparse.readthedocs.io/en/latest/pages/parsing-audio.html
[parsing-hparams]: https://tbparse.readthedocs.io/en/latest/pages/parsing-hparams.html
[parsing-text]: https://tbparse.readthedocs.io/en/latest/pages/parsing-text.html
All events above are generated and plotted in [gallery-pytorch.ipynb](https://github.com/j3soon/tbparse/blob/master/docs/notebooks/gallery-pytorch.ipynb).
## Installation
```sh
pip install tensorflow # or tensorflow-cpu
pip install -U tbparse # requires Python >= 3.7
```
**Note**: If you don't want to install TensorFlow, see [Installing without TensorFlow](https://tbparse.readthedocs.io/en/latest/pages/installation.html#installing-without-tensorflow).
## Testing the Source Code
Test source code:
```sh
python3 -m pip install tox
python3 -m tox
```
Test and build documentation:
```sh
cd docs
make clean
# sphinx-tabs seems to require html be built before doctest
make html
make doctest
```
Generate test coverage:
```sh
python3 -m pip install pandas tensorflow torch tensorboardX pytest pytest-cov
python3 -m pytest --cov=./ --cov-report html
```
## License
tbparse is distributed under the [Apache License 2.0](LICENSE).
The tbparse PyPI package depends on (imports) the following third-party package. Each third-party software package is provided under its own license:
- [pandas](https://github.com/pandas-dev/pandas) is distributed under the [BSD-3-Clause License ](https://github.com/pandas-dev/pandas/blob/main/LICENSE)
- [tensorboard](https://github.com/tensorflow/tensorboard) is distributed under the [Apache License 2.0](https://github.com/tensorflow/tensorboard/blob/master/LICENSE)
Raw data
{
"_id": null,
"home_page": "https://github.com/j3soon/tbparse",
"name": "tbparse",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "",
"keywords": "package,parser,plot,python,pytorch,reader,tensorboard,tensorboardx,tensorflow",
"author": "Johnson",
"author_email": "admin@j3soon.com",
"download_url": "https://files.pythonhosted.org/packages/dc/94/454fb5b3d8156b2d055c5e6537db0f26b8a784097d8bbe36c31223861657/tbparse-0.0.7.tar.gz",
"platform": null,
"description": "# tbparse\n\n[](https://pypi.org/project/tbparse/)\n[](https://pypi.org/project/tbparse/)\n[](https://pepy.tech/project/tbparse)\n[](https://github.com/j3soon/tbparse/blob/master/LICENSE)\n\n[](https://github.com/j3soon/tbparse/actions/workflows/test-with-tox.yaml)\n[](https://github.com/j3soon/tbparse/actions/workflows/publish-to-pypi.yaml)\n[](https://tbparse.readthedocs.io/en/latest)\n[](https://codecov.io/gh/j3soon/tbparse)\n\nA simple yet powerful tensorboard event log parser/reader.\n\n* Supports parsing tensorboard event [scalars][parsing-scalars], [tensors][parsing-tensors], [histograms][parsing-histograms], [images][parsing-images], [audio][parsing-audio], [hparams][parsing-hparams], and [text][parsing-text].\n* Supports event generated by\n [PyTorch](https://pytorch.org/docs/stable/tensorboard.html), [Tensorboard/Keras](https://www.tensorflow.org/tensorboard), and [TensorboardX](https://github.com/lanpa/tensorboardX), with their respective usage examples documented in detail.\n* Allows parsing multiple tensorboard event files in a hierarchical directory structure.\n* Provides plotting examples for each type of events.\n* Stores the data in [pandas.DataFrame](https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.html) to allow advanced filtering.\n* Both the documentation and code have high test coverage rate.\n* Follows [PEP 484](https://www.python.org/dev/peps/pep-0484/) with full type hints.\n\nInstallation:\n\n```sh\npip install tensorflow # or tensorflow-cpu\npip install -U tbparse # requires Python >= 3.7\n```\n\n**Note**: If you don't want to install TensorFlow, see [Installing without TensorFlow](https://tbparse.readthedocs.io/en/latest/pages/installation.html#installing-without-tensorflow).\n\nWe suggest using an additional virtual environment for parsing and plotting the tensorboard events. So no worries if your training code uses Python 3.6 or older versions. \n\nReading one or more event files with tbparse only requires 5 lines of code:\n\n```py\nfrom tbparse import SummaryReader\nlog_dir = \"<PATH_TO_EVENT_FILE_OR_DIRECTORY>\"\nreader = SummaryReader(log_dir)\ndf = reader.scalars\nprint(df)\n```\n\nFor beginners, start from the page: [Parsing Scalars][parsing-scalars].\n\n## Gallery\n\n| Event Type | Tensorboard Dashboard | tbparse |\n|----------------------------------|----------------------------------------------|-----------------------------------------|\n| [Scalars][parsing-scalars] | 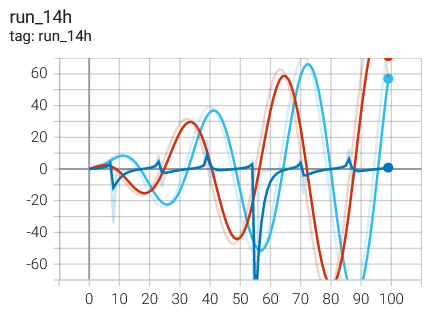 |  |\n| [Tensors][parsing-tensors] | 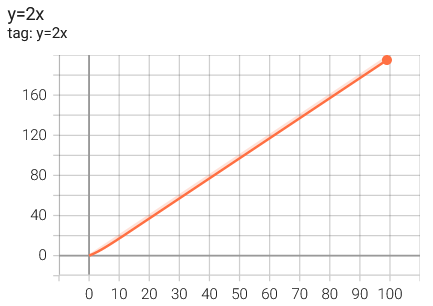 |  |\n| [Histograms][parsing-histograms] | 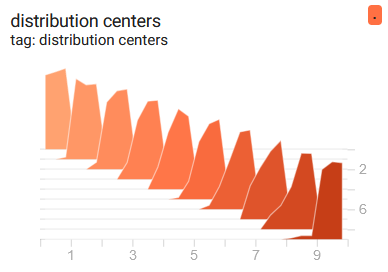 |  |\n| [Images][parsing-images] | 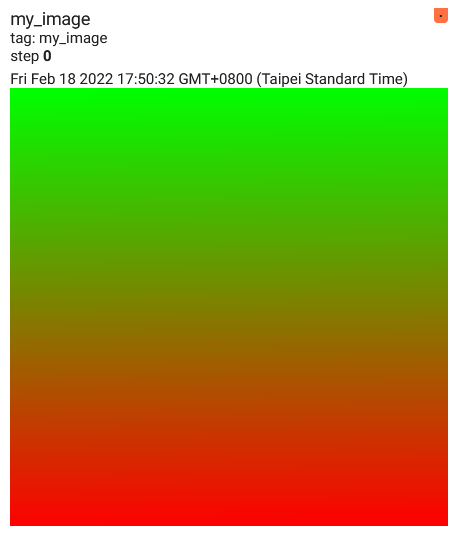 | 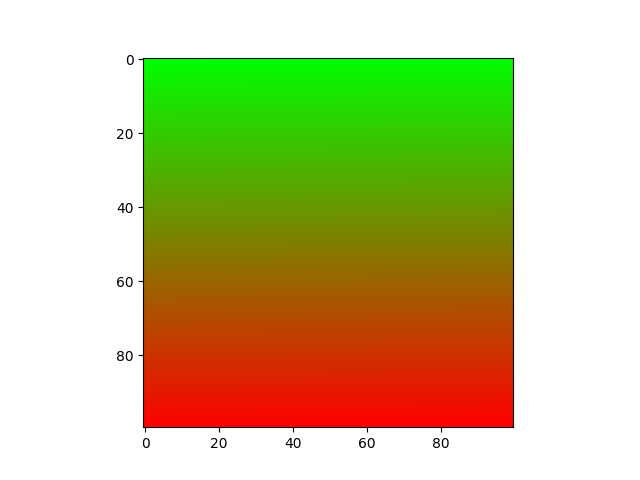 |\n| [Audio][parsing-audio] | 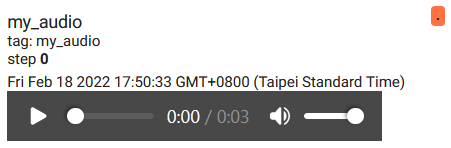 |  |\n| [HParams][parsing-hparams] |  | 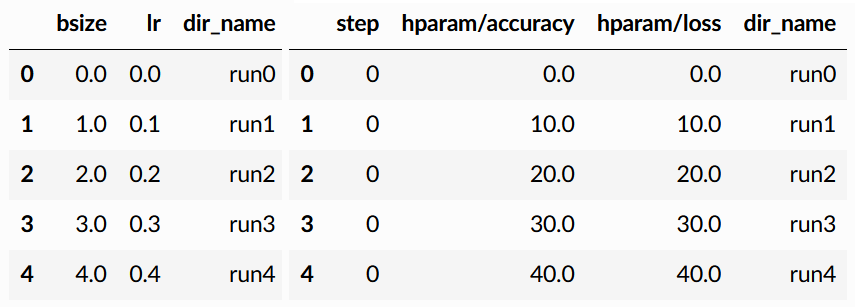 |\n| [Text][parsing-text] |  |  |\n\n[parsing-scalars]: https://tbparse.readthedocs.io/en/latest/pages/parsing-scalars.html\n[parsing-tensors]: https://tbparse.readthedocs.io/en/latest/pages/parsing-tensors.html\n[parsing-histograms]: https://tbparse.readthedocs.io/en/latest/pages/parsing-histograms.html\n[parsing-images]: https://tbparse.readthedocs.io/en/latest/pages/parsing-images.html\n[parsing-audio]: https://tbparse.readthedocs.io/en/latest/pages/parsing-audio.html\n[parsing-hparams]: https://tbparse.readthedocs.io/en/latest/pages/parsing-hparams.html\n[parsing-text]: https://tbparse.readthedocs.io/en/latest/pages/parsing-text.html\n\nAll events above are generated and plotted in [gallery-pytorch.ipynb](https://github.com/j3soon/tbparse/blob/master/docs/notebooks/gallery-pytorch.ipynb).\n\n## Installation\n\n```sh\npip install tensorflow # or tensorflow-cpu\npip install -U tbparse # requires Python >= 3.7\n```\n\n**Note**: If you don't want to install TensorFlow, see [Installing without TensorFlow](https://tbparse.readthedocs.io/en/latest/pages/installation.html#installing-without-tensorflow).\n\n## Testing the Source Code\n\nTest source code:\n\n```sh\npython3 -m pip install tox\npython3 -m tox\n```\n\nTest and build documentation:\n\n```sh\ncd docs\nmake clean\n# sphinx-tabs seems to require html be built before doctest\nmake html\nmake doctest\n```\n\nGenerate test coverage:\n\n```sh\npython3 -m pip install pandas tensorflow torch tensorboardX pytest pytest-cov\npython3 -m pytest --cov=./ --cov-report html\n```\n\n## License\n\ntbparse is distributed under the [Apache License 2.0](LICENSE).\n\nThe tbparse PyPI package depends on (imports) the following third-party package. Each third-party software package is provided under its own license:\n- [pandas](https://github.com/pandas-dev/pandas) is distributed under the [BSD-3-Clause License ](https://github.com/pandas-dev/pandas/blob/main/LICENSE)\n- [tensorboard](https://github.com/tensorflow/tensorboard) is distributed under the [Apache License 2.0](https://github.com/tensorflow/tensorboard/blob/master/LICENSE)\n",
"bugtrack_url": null,
"license": "",
"summary": "Load tensorboard event logs as pandas DataFrames; Read, parse, and plot tensorboard event logs with ease!",
"version": "0.0.7",
"split_keywords": [
"package",
"parser",
"plot",
"python",
"pytorch",
"reader",
"tensorboard",
"tensorboardx",
"tensorflow"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "6984bda53f22def6091216123e7e8cc89a916bfdc31c03f26f71a7ab871af5f6",
"md5": "4e98d76c531f81533bdfe563404d4897",
"sha256": "f9a140f45b8ff00158fd406aba0b3b56a46793d6ea65db346e8d80db0e4fb90d"
},
"downloads": -1,
"filename": "tbparse-0.0.7-py3-none-any.whl",
"has_sig": false,
"md5_digest": "4e98d76c531f81533bdfe563404d4897",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 17193,
"upload_time": "2022-08-06T16:59:26",
"upload_time_iso_8601": "2022-08-06T16:59:26.783667Z",
"url": "https://files.pythonhosted.org/packages/69/84/bda53f22def6091216123e7e8cc89a916bfdc31c03f26f71a7ab871af5f6/tbparse-0.0.7-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "dc94454fb5b3d8156b2d055c5e6537db0f26b8a784097d8bbe36c31223861657",
"md5": "6d4de885b004869055015c88b023a222",
"sha256": "0ddd3c764ceb1859bc0cb69ca355bff4fd5936c4bfe885e252e481564b2371a9"
},
"downloads": -1,
"filename": "tbparse-0.0.7.tar.gz",
"has_sig": false,
"md5_digest": "6d4de885b004869055015c88b023a222",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 19178,
"upload_time": "2022-08-06T16:59:28",
"upload_time_iso_8601": "2022-08-06T16:59:28.230571Z",
"url": "https://files.pythonhosted.org/packages/dc/94/454fb5b3d8156b2d055c5e6537db0f26b8a784097d8bbe36c31223861657/tbparse-0.0.7.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2022-08-06 16:59:28",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "j3soon",
"github_project": "tbparse",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"requirements": [],
"tox": true,
"lcname": "tbparse"
}