Name | vbox-api-soap JSON |
Version |
2.1.1
JSON |
| download |
home_page | None |
Summary | Python bindings to the VirtualBox SOAP API. |
upload_time | 2025-01-07 09:43:42 |
maintainer | Zack Didcott |
docs_url | None |
author | Zack Didcott |
requires_python | >=3.10 |
license | The MIT License (MIT) Copyright © 2024 Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the “Software”), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
virtualbox
api
soap
zeep
flask
|
VCS |
 |
bugtrack_url |
|
requirements |
attrs
blinker
certifi
cffi
charset-normalizer
click
cryptography
Flask
idna
iniconfig
isodate
itsdangerous
Jinja2
jwcrypto
lxml
MarkupSafe
numpy
packaging
pillow
platformdirs
pluggy
psutil
pycparser
pytest
pytz
redis
requests
requests-file
requests-toolbelt
simplejson
six
typing_extensions
urllib3
websockify
Werkzeug
zeep
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# vbox-api
[](https://github.com/Zedeldi/vbox-api/blob/master/LICENSE) [](https://github.com/Zedeldi/vbox-api/commits) [](https://github.com/psf/black)
Python bindings to the VirtualBox SOAP API.
## Table of Contents
- [Description](#description)
- [Examples](#examples)
- [Components](#components)
- [Models](#models)
- [Installation](#installation)
- [PyPI](#pypi)
- [Source](#source)
- [PyInstaller](#pyinstaller)
- [PKGBUILD](#pkgbuild)
- [Docker](#docker)
- [Usage](#usage)
- [Machine](#machine)
- [Medium](#medium)
- [Libraries](#libraries)
- [Testing](#testing)
- [Contributing](#contributing)
- [Changelog](#changelog)
- [Credits](#credits)
- [Contributors](#contributors)
- [Resources](#resources)
- [Other Projects](#other-projects)
- [License](#license)
- [Donate](#donate)
## Description
Provides a Python SOAP client using `zeep` to the VirtualBox SOAP API, with Pythonic bindings, and models for object-oriented usage.
Several methods have been added to models to assist with common operations, and simplify many interface methods.
### Examples
Command-line interface:
<p align="center">
<img src="docs/vbox-api-cli.gif?raw=true" alt="Demonstration of vbox-api-cli."/>
</p>
Web (HTTP) interface:
<p align="center">
<img src="docs/vbox-api-http-machine-view.png?raw=true" alt="Demonstration of vbox-api-http machine.view endpoint."/>
</p>
### Components
`vbox_api.interface` contains all relevant classes to communicate with the VirtualBox API.
`vbox_api.models` defines classes to use an interface in an object-oriented approach (see [below](#models)).
`vbox_api.cli` contains an entry point for an interactive command-line interface and functions to parse arguments.
`vbox_api.http` includes a Flask application to view and manage virtual machines over HTTP.
`vbox_api.constants` defines many enumerations, mostly generated from the VirtualBox WSDL XML, with added enumerations for those not currently specified but used internally, such as `MachineFrontend`.
These can be used as a reference for expected values to/from the VirtualBox interface, when type hinting or passing values.
`vbox_api.helpers` includes a class, `WebSocketProxyProcess`, to wrap `websockify.WebSocketProxy`, allowing remote control of a virtual machine over HTTP, accessible from the web interface.
`vrde_ext_pack` must be set to `VNC` and [noVNC](https://novnc.com) must be available.
### Models
Models allow properties to be obtained and set in an object-oriented fashion, e.g. `machine.name`, instead of `IMachine_getName(handle)`.
To do this, `BaseModel` defines both a `__getattr__` and a `__setattr__` method, which call the relevant interface method for the requested attribute to either get or set, respectively.
Additionally, the `to_dict` and `from_dict` methods allow multiple properties to be handled at once.
Any valid interface name can be used to create a model, using `BaseModel.from_name`.
The interface is passed via a `Context` object, along with the handle of the model.
All methods of the interface are bound to this model, using `functools.partial` to implictly pass its handle, then wrapped to return model instances.
`BaseModel` also implements `__str__`, allowing models to be passed directly to interface methods as a handle.
Models are automatically instantiated from returned results, by obtaining the interface name from the returned handle, using `ManagedObjectRef.get_interface_name`.
Alternatively, a passed value can be matched by string comparison to an existing interface name.
A metaclass is used to ensure that objects with the same handle and class are not recreated; the same object is returned.
The instances are stored in a `WeakValueDictionary`, allowing them to be garbage collected when no longer referenced.
For example:
```py
machine = api.machines[0]
assert machine is Machine(api.ctx, machine.handle)
```
## Installation
### PyPI
1. Install project: `pip install vbox-api-soap`
2. Run: `vbox-api-cli` (CLI) or `vbox-api-http` (HTTP)
As the name `vbox-api` conflicts with official `vboxapi` on PyPI,
`vbox-api-soap` is used as the package name.
### Source
Alternativelty, after cloning the repository with:
`git clone https://github.com/Zedeldi/vbox-api.git`
#### Build
1. Install project: `pip install .`
2. Run: `vbox-api-cli` (CLI) or `vbox-api-http` (HTTP)
#### Development
1. Install dependencies: `pip install -r requirements.txt`
2. Run: `python -m vbox_api.cli` (CLI) or `python -m vbox_api.http` (HTTP)
### PyInstaller
The server application can be bundled with PyInstaller, by adding the required static files and templates for Flask to the bundle.
Additionally, `vbox_api.http.gui` provides a simple wrapper using [flaskwebgui](https://github.com/ClimenteA/flaskwebgui), to serve and open the Flask application in a browser window.
```sh
pyinstaller \
--name "vbox-api-gui" \
--add-data "vbox_api/http/static:vbox_api/http/static" \
--add-data "vbox_api/http/templates:vbox_api/http/templates" \
--onefile \
vbox_api/http/gui.py
```
### PKGBUILD
1. Build and install the package: `makepkg -si`
2. Run: `vbox-api-cli` (CLI) or `vbox-api-http` (HTTP)
- The HTTP interface can also be started using a systemd unit: `systemctl start vbox-api-http`
Note: if the optional dependency `novnc` is installed from the AUR, HTTP remote control will be available out of the box.
### Docker
Dockerfiles are supplied in `docker/`, to run both `vbox-api-http` and `vboxwebsrv` in a container.
If you already have VirtualBox installed to your machine, you can build and start `vbox-api-http` in a Docker container easily.
The VirtualBox container requires the host kernel modules to be installed for `/dev/vboxdrv` to be available.
#### Build
Build vbox-api image, passing current directory as the build context:
```sh
docker build -t "vbox-api" -f docker/api/Dockerfile .
```
Build VirtualBox image, optionally passing additional build arguments:
```sh
docker build -t "virtualbox" -f docker/virtualbox/Dockerfile .
docker build -t "virtualbox" --build-arg USER="user" --build-arg PASS="password" -f docker/virtualbox/Dockerfile .
docker build -t "virtualbox" --build-arg PACMAN_ARGS="--disable-download-timeout" -f docker/virtualbox/Dockerfile .
```
#### Run
Run `vbox-api-http` in a container interactively, with host network:
```sh
docker run -it --rm --network=host "vbox-api"
```
Run `vboxwebsrv` in a container interactively, with host network (note: VirtualBox host kernel modules must be installed):
```sh
docker run -it --rm --network=host --device /dev/vboxdrv:/dev/vboxdrv -e DISPLAY=unix:0 "virtualbox"
```
## Usage
Ensure that `vboxwebsrv` is running as the intended user.
The `virtualbox` package also provides a systemd unit for this: `systemctl start vboxweb`.
In the following examples, `api` refers to a `VBoxAPI` (or `VirtualBox` model) instance.
### Machine
Get machine information:
```py
for machine in api.machines:
print(machine.to_dict())
```
Wait for machine to start in headless mode:
```py
progress = machine.start(front_end="headless") # or MachineFrontend.HEADLESS
progress.wait_for_completion(-1)
```
Write-lock machine and set machine name:
```py
with machine.with_lock(save_settings=True) as locked_machine:
locked_machine.name = "Machine Name"
# or
locked_machine.set_name("Machine Name")
assert machine.name == "Machine Name"
```
Create machine with default settings for Windows 11:
```py
machine = api.create_machine_with_defaults(
name="Windows 11",
os_type_id="Windows11_64",
)
```
Create machine from Windows 11 ISO and start unattended installation:
```py
machine = api.create_machine_from_iso(
iso_path="Win11_23H2_EnglishInternational_x64.iso",
name="Windows 11",
unattended_options={
"user": "username",
"password": "password",
"install_guest_additions": True,
},
)
machine.attach_medium(hard_disk) # See Medium examples
machine.start()
```
Clone machine:
```py
cloned_machine = machine.clone(f"{machine.name} - Clone")
```
Interact with guest OS of machine:
```py
with machine.with_lock() as locked_machine:
guest = locked_machine.session.console.guest
session = guest.create_session("username", "password", "domain", "session_name")
# Wait for session.status to be GuestSessionStatus.STARTED
progress = session.copy_from_guest(r"C:\path\to\copy", [], FileCopyFlag.NONE, "/path/to/destination/")
process = session.process_create(r"C:\path\to\file.exe", ["argument"], ["var=1"], ProcessCreateFlag.NONE, 0)
```
Teleport machine to another on the same host, with a password:
```py
# Configure then start target machine
target_machine.teleport_listen(port=6000, password="password")
target_machine.start()
# Start then teleport source machine to target machine
source_machine.start()
source_machine.teleport_to(host="localhost", port=6000, password="password")
```
### Medium
Get medium information:
```py
for medium in api.mediums: # or specify dvd_images, floppy_images, hard_disks
print(medium.to_dict())
```
Create hard-disk medium:
```py
medium = api.create_medium_with_defaults(
location="/path/to/medium.vdi",
logical_size=1024 ** 4, # 1 TiB
format_="VDI",
)
```
## Libraries
- [Zeep](https://pypi.org/project/zeep/) - SOAP client
- [Flask](https://pypi.org/project/Flask/) - HTTP interface
- [Pillow](https://pypi.org/project/pillow/) - image support
- [psutil](https://pypi.org/project/psutil/) - process information
- [websockify](https://pypi.org/project/websockify/) - remote control
## Testing
`vbox-api` has been tested successfully on Arch Linux and Windows 10.
Unit tests can be run using [pytest](https://pypi.org/project/pytest/), though tests require authentication for the VirtualBox interface: `python -m pytest`
## Contributing
Please contribute by raising an [issue](https://github.com/Zedeldi/vbox-api/issues) or submitting a [pull request](https://github.com/Zedeldi/vbox-api/pulls), whether for code or documentation.
Source code is formatted using [black](https://pypi.org/project/black/), and type hints should be added where possible.
If there are any questions, please do not hesitate to ask.
All contributions are welcome!
## Changelog
For VirtualBox `v7.1.0` onwards (released 09/09/2024), use `v2.0.0` or above of `vbox-api`.
`v7.1.0` was a major update, causing some breaking changes with their API, specifically with the introduction of `PlatformProperties`.
For previous versions of VirtualBox, use `v1.5.3` or below.
For more information, see the [VirtualBox Changelog](https://www.virtualbox.org/wiki/Changelog).
## Credits
### Contributors
- [@Zedeldi](https://github.com/Zedeldi) - creator & maintainer
### Resources
- [VirtualBox](https://www.virtualbox.org/) - well, duh.
- [API Documentation](https://www.virtualbox.org/sdkref/) - reference for interface methods and properties
- [Bootstrap](https://getbootstrap.com/) - used for HTTP interface
- [noVNC](https://novnc.com)/[websockify](https://github.com/novnc/websockify) - support for HTTP remote control
- See [libraries](#libraries) for list of dependencies
### Other Projects
- [vboxwebber](https://github.com/larshson/vboxwebber/) - VirtualBox SOAP API client for Python
- [phpVirtualBox](https://github.com/phpvirtualbox/phpvirtualbox) - web interface to manage and access Virtualbox machines
- [RemoteBox](https://remotebox.knobgoblin.org.uk/) - VirtualBox GUI (GTK3) client
## License
`vbox-api` is licensed under the [MIT Licence](https://mit-license.org/) for everyone to use, modify and share freely.
This project is distributed in the hope that it will be useful, but without any warranty.
The VirtualBox logo is licensed under the [GPL-2.0](https://www.gnu.org/licenses/old-licenses/gpl-2.0.html).
## Donate
If you found this project useful, please consider donating. Any amount is greatly appreciated! Thank you :smiley:
[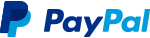](https://paypal.me/ZackDidcott)
Raw data
{
"_id": null,
"home_page": null,
"name": "vbox-api-soap",
"maintainer": "Zack Didcott",
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": null,
"keywords": "virtualbox, api, soap, zeep, flask",
"author": "Zack Didcott",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/4c/d2/17a5137149a29f7cde575ca2bfe6c167d238c3d48dc7cc9cc0ddd6b0f7d6/vbox_api_soap-2.1.1.tar.gz",
"platform": null,
"description": "# vbox-api\n\n[](https://github.com/Zedeldi/vbox-api/blob/master/LICENSE) [](https://github.com/Zedeldi/vbox-api/commits) [](https://github.com/psf/black)\n\nPython bindings to the VirtualBox SOAP API.\n\n## Table of Contents\n\n- [Description](#description)\n - [Examples](#examples)\n - [Components](#components)\n - [Models](#models)\n- [Installation](#installation)\n - [PyPI](#pypi)\n - [Source](#source)\n - [PyInstaller](#pyinstaller)\n - [PKGBUILD](#pkgbuild)\n - [Docker](#docker)\n- [Usage](#usage)\n - [Machine](#machine)\n - [Medium](#medium)\n- [Libraries](#libraries)\n- [Testing](#testing)\n- [Contributing](#contributing)\n- [Changelog](#changelog)\n- [Credits](#credits)\n - [Contributors](#contributors)\n - [Resources](#resources)\n - [Other Projects](#other-projects)\n- [License](#license)\n- [Donate](#donate)\n\n## Description\n\nProvides a Python SOAP client using `zeep` to the VirtualBox SOAP API, with Pythonic bindings, and models for object-oriented usage.\nSeveral methods have been added to models to assist with common operations, and simplify many interface methods.\n\n### Examples\n\nCommand-line interface:\n\n<p align=\"center\">\n <img src=\"docs/vbox-api-cli.gif?raw=true\" alt=\"Demonstration of vbox-api-cli.\"/>\n</p>\n\nWeb (HTTP) interface:\n\n<p align=\"center\">\n <img src=\"docs/vbox-api-http-machine-view.png?raw=true\" alt=\"Demonstration of vbox-api-http machine.view endpoint.\"/>\n</p>\n\n\n### Components\n\n`vbox_api.interface` contains all relevant classes to communicate with the VirtualBox API.\n\n`vbox_api.models` defines classes to use an interface in an object-oriented approach (see [below](#models)).\n\n`vbox_api.cli` contains an entry point for an interactive command-line interface and functions to parse arguments.\n\n`vbox_api.http` includes a Flask application to view and manage virtual machines over HTTP.\n\n`vbox_api.constants` defines many enumerations, mostly generated from the VirtualBox WSDL XML, with added enumerations for those not currently specified but used internally, such as `MachineFrontend`.\nThese can be used as a reference for expected values to/from the VirtualBox interface, when type hinting or passing values.\n\n`vbox_api.helpers` includes a class, `WebSocketProxyProcess`, to wrap `websockify.WebSocketProxy`, allowing remote control of a virtual machine over HTTP, accessible from the web interface.\n`vrde_ext_pack` must be set to `VNC` and [noVNC](https://novnc.com) must be available.\n\n### Models\n\nModels allow properties to be obtained and set in an object-oriented fashion, e.g. `machine.name`, instead of `IMachine_getName(handle)`.\nTo do this, `BaseModel` defines both a `__getattr__` and a `__setattr__` method, which call the relevant interface method for the requested attribute to either get or set, respectively.\nAdditionally, the `to_dict` and `from_dict` methods allow multiple properties to be handled at once.\n\nAny valid interface name can be used to create a model, using `BaseModel.from_name`.\nThe interface is passed via a `Context` object, along with the handle of the model.\nAll methods of the interface are bound to this model, using `functools.partial` to implictly pass its handle, then wrapped to return model instances.\n`BaseModel` also implements `__str__`, allowing models to be passed directly to interface methods as a handle.\n\nModels are automatically instantiated from returned results, by obtaining the interface name from the returned handle, using `ManagedObjectRef.get_interface_name`.\nAlternatively, a passed value can be matched by string comparison to an existing interface name.\n\nA metaclass is used to ensure that objects with the same handle and class are not recreated; the same object is returned.\nThe instances are stored in a `WeakValueDictionary`, allowing them to be garbage collected when no longer referenced.\n\nFor example:\n\n```py\nmachine = api.machines[0]\nassert machine is Machine(api.ctx, machine.handle)\n```\n\n## Installation\n\n### PyPI\n\n1. Install project: `pip install vbox-api-soap`\n2. Run: `vbox-api-cli` (CLI) or `vbox-api-http` (HTTP)\n\nAs the name `vbox-api` conflicts with official `vboxapi` on PyPI,\n`vbox-api-soap` is used as the package name.\n\n### Source\n\nAlternativelty, after cloning the repository with:\n`git clone https://github.com/Zedeldi/vbox-api.git`\n\n#### Build\n\n1. Install project: `pip install .`\n2. Run: `vbox-api-cli` (CLI) or `vbox-api-http` (HTTP)\n\n#### Development\n\n1. Install dependencies: `pip install -r requirements.txt`\n2. Run: `python -m vbox_api.cli` (CLI) or `python -m vbox_api.http` (HTTP)\n\n### PyInstaller\n\nThe server application can be bundled with PyInstaller, by adding the required static files and templates for Flask to the bundle.\nAdditionally, `vbox_api.http.gui` provides a simple wrapper using [flaskwebgui](https://github.com/ClimenteA/flaskwebgui), to serve and open the Flask application in a browser window.\n\n```sh\npyinstaller \\\n --name \"vbox-api-gui\" \\\n --add-data \"vbox_api/http/static:vbox_api/http/static\" \\\n --add-data \"vbox_api/http/templates:vbox_api/http/templates\" \\\n --onefile \\\n vbox_api/http/gui.py\n```\n\n### PKGBUILD\n\n1. Build and install the package: `makepkg -si`\n2. Run: `vbox-api-cli` (CLI) or `vbox-api-http` (HTTP)\n - The HTTP interface can also be started using a systemd unit: `systemctl start vbox-api-http`\n\nNote: if the optional dependency `novnc` is installed from the AUR, HTTP remote control will be available out of the box.\n\n### Docker\n\nDockerfiles are supplied in `docker/`, to run both `vbox-api-http` and `vboxwebsrv` in a container.\n\nIf you already have VirtualBox installed to your machine, you can build and start `vbox-api-http` in a Docker container easily.\nThe VirtualBox container requires the host kernel modules to be installed for `/dev/vboxdrv` to be available.\n\n#### Build\n\nBuild vbox-api image, passing current directory as the build context:\n```sh\ndocker build -t \"vbox-api\" -f docker/api/Dockerfile .\n```\n\nBuild VirtualBox image, optionally passing additional build arguments:\n```sh\ndocker build -t \"virtualbox\" -f docker/virtualbox/Dockerfile .\ndocker build -t \"virtualbox\" --build-arg USER=\"user\" --build-arg PASS=\"password\" -f docker/virtualbox/Dockerfile .\ndocker build -t \"virtualbox\" --build-arg PACMAN_ARGS=\"--disable-download-timeout\" -f docker/virtualbox/Dockerfile .\n```\n\n#### Run\n\nRun `vbox-api-http` in a container interactively, with host network:\n```sh\ndocker run -it --rm --network=host \"vbox-api\"\n```\n\nRun `vboxwebsrv` in a container interactively, with host network (note: VirtualBox host kernel modules must be installed):\n```sh\ndocker run -it --rm --network=host --device /dev/vboxdrv:/dev/vboxdrv -e DISPLAY=unix:0 \"virtualbox\"\n```\n\n## Usage\n\nEnsure that `vboxwebsrv` is running as the intended user.\nThe `virtualbox` package also provides a systemd unit for this: `systemctl start vboxweb`.\n\nIn the following examples, `api` refers to a `VBoxAPI` (or `VirtualBox` model) instance.\n\n### Machine\n\nGet machine information:\n\n```py\nfor machine in api.machines:\n print(machine.to_dict())\n```\n\nWait for machine to start in headless mode:\n\n```py\nprogress = machine.start(front_end=\"headless\") # or MachineFrontend.HEADLESS\nprogress.wait_for_completion(-1)\n```\n\nWrite-lock machine and set machine name:\n\n```py\nwith machine.with_lock(save_settings=True) as locked_machine:\n locked_machine.name = \"Machine Name\"\n # or\n locked_machine.set_name(\"Machine Name\")\nassert machine.name == \"Machine Name\"\n```\n\nCreate machine with default settings for Windows 11:\n\n```py\nmachine = api.create_machine_with_defaults(\n name=\"Windows 11\",\n os_type_id=\"Windows11_64\",\n)\n```\n\nCreate machine from Windows 11 ISO and start unattended installation:\n\n```py\nmachine = api.create_machine_from_iso(\n iso_path=\"Win11_23H2_EnglishInternational_x64.iso\",\n name=\"Windows 11\",\n unattended_options={\n \"user\": \"username\",\n \"password\": \"password\",\n \"install_guest_additions\": True,\n },\n)\nmachine.attach_medium(hard_disk) # See Medium examples\nmachine.start()\n```\n\nClone machine:\n\n```py\ncloned_machine = machine.clone(f\"{machine.name} - Clone\")\n```\n\nInteract with guest OS of machine:\n\n```py\nwith machine.with_lock() as locked_machine:\n guest = locked_machine.session.console.guest\n session = guest.create_session(\"username\", \"password\", \"domain\", \"session_name\")\n # Wait for session.status to be GuestSessionStatus.STARTED\n progress = session.copy_from_guest(r\"C:\\path\\to\\copy\", [], FileCopyFlag.NONE, \"/path/to/destination/\")\n process = session.process_create(r\"C:\\path\\to\\file.exe\", [\"argument\"], [\"var=1\"], ProcessCreateFlag.NONE, 0)\n```\n\nTeleport machine to another on the same host, with a password:\n\n```py\n# Configure then start target machine\ntarget_machine.teleport_listen(port=6000, password=\"password\")\ntarget_machine.start()\n\n# Start then teleport source machine to target machine\nsource_machine.start()\nsource_machine.teleport_to(host=\"localhost\", port=6000, password=\"password\")\n```\n\n### Medium\n\nGet medium information:\n\n```py\nfor medium in api.mediums: # or specify dvd_images, floppy_images, hard_disks\n print(medium.to_dict())\n```\n\nCreate hard-disk medium:\n\n```py\nmedium = api.create_medium_with_defaults(\n location=\"/path/to/medium.vdi\",\n logical_size=1024 ** 4, # 1 TiB\n format_=\"VDI\",\n)\n```\n\n## Libraries\n\n- [Zeep](https://pypi.org/project/zeep/) - SOAP client\n- [Flask](https://pypi.org/project/Flask/) - HTTP interface\n- [Pillow](https://pypi.org/project/pillow/) - image support\n- [psutil](https://pypi.org/project/psutil/) - process information\n- [websockify](https://pypi.org/project/websockify/) - remote control\n\n## Testing\n\n`vbox-api` has been tested successfully on Arch Linux and Windows 10.\n\nUnit tests can be run using [pytest](https://pypi.org/project/pytest/), though tests require authentication for the VirtualBox interface: `python -m pytest`\n\n## Contributing\n\nPlease contribute by raising an [issue](https://github.com/Zedeldi/vbox-api/issues) or submitting a [pull request](https://github.com/Zedeldi/vbox-api/pulls), whether for code or documentation.\n\nSource code is formatted using [black](https://pypi.org/project/black/), and type hints should be added where possible.\n\nIf there are any questions, please do not hesitate to ask.\nAll contributions are welcome!\n\n## Changelog\n\nFor VirtualBox `v7.1.0` onwards (released 09/09/2024), use `v2.0.0` or above of `vbox-api`.\n`v7.1.0` was a major update, causing some breaking changes with their API, specifically with the introduction of `PlatformProperties`.\n\nFor previous versions of VirtualBox, use `v1.5.3` or below.\n\nFor more information, see the [VirtualBox Changelog](https://www.virtualbox.org/wiki/Changelog).\n\n## Credits\n\n### Contributors\n\n - [@Zedeldi](https://github.com/Zedeldi) - creator & maintainer\n\n### Resources\n\n - [VirtualBox](https://www.virtualbox.org/) - well, duh.\n - [API Documentation](https://www.virtualbox.org/sdkref/) - reference for interface methods and properties\n - [Bootstrap](https://getbootstrap.com/) - used for HTTP interface\n - [noVNC](https://novnc.com)/[websockify](https://github.com/novnc/websockify) - support for HTTP remote control\n - See [libraries](#libraries) for list of dependencies\n\n### Other Projects\n\n - [vboxwebber](https://github.com/larshson/vboxwebber/) - VirtualBox SOAP API client for Python\n - [phpVirtualBox](https://github.com/phpvirtualbox/phpvirtualbox) - web interface to manage and access Virtualbox machines\n - [RemoteBox](https://remotebox.knobgoblin.org.uk/) - VirtualBox GUI (GTK3) client\n\n## License\n\n`vbox-api` is licensed under the [MIT Licence](https://mit-license.org/) for everyone to use, modify and share freely.\n\nThis project is distributed in the hope that it will be useful, but without any warranty.\n\nThe VirtualBox logo is licensed under the [GPL-2.0](https://www.gnu.org/licenses/old-licenses/gpl-2.0.html).\n\n## Donate\n\nIf you found this project useful, please consider donating. Any amount is greatly appreciated! Thank you :smiley:\n\n[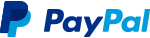](https://paypal.me/ZackDidcott)\n",
"bugtrack_url": null,
"license": "The MIT License (MIT) Copyright \u00a9 2024 Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \u201cSoftware\u201d), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \u201cAS IS\u201d, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.",
"summary": "Python bindings to the VirtualBox SOAP API.",
"version": "2.1.1",
"project_urls": {
"Homepage": "https://github.com/Zedeldi/vbox-api",
"Issues": "https://github.com/Zedeldi/vbox-api/issues",
"Repository": "https://github.com/Zedeldi/vbox-api.git"
},
"split_keywords": [
"virtualbox",
" api",
" soap",
" zeep",
" flask"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "481bdf9af9d6e35c0df607f9d311503c5535df554ebe042d48a767d2390f9579",
"md5": "2073ca9464df1d303d852fe0c8812e0a",
"sha256": "0f9da2b61e420580ab9a5208c181f239b8ff042a342a4a08050076cde38484de"
},
"downloads": -1,
"filename": "vbox_api_soap-2.1.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "2073ca9464df1d303d852fe0c8812e0a",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10",
"size": 126935,
"upload_time": "2025-01-07T09:43:38",
"upload_time_iso_8601": "2025-01-07T09:43:38.958260Z",
"url": "https://files.pythonhosted.org/packages/48/1b/df9af9d6e35c0df607f9d311503c5535df554ebe042d48a767d2390f9579/vbox_api_soap-2.1.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4cd217a5137149a29f7cde575ca2bfe6c167d238c3d48dc7cc9cc0ddd6b0f7d6",
"md5": "1bbeaf5acfd4daffddbe98fff1b8cf91",
"sha256": "67dda377c4375e6eb921309f31dcc2910193b94e010c91e45238f2a9694892b0"
},
"downloads": -1,
"filename": "vbox_api_soap-2.1.1.tar.gz",
"has_sig": false,
"md5_digest": "1bbeaf5acfd4daffddbe98fff1b8cf91",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10",
"size": 117506,
"upload_time": "2025-01-07T09:43:42",
"upload_time_iso_8601": "2025-01-07T09:43:42.052858Z",
"url": "https://files.pythonhosted.org/packages/4c/d2/17a5137149a29f7cde575ca2bfe6c167d238c3d48dc7cc9cc0ddd6b0f7d6/vbox_api_soap-2.1.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-01-07 09:43:42",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Zedeldi",
"github_project": "vbox-api",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [
{
"name": "attrs",
"specs": [
[
"==",
"23.2.0"
]
]
},
{
"name": "blinker",
"specs": [
[
"==",
"1.7.0"
]
]
},
{
"name": "certifi",
"specs": [
[
"==",
"2024.2.2"
]
]
},
{
"name": "cffi",
"specs": [
[
"==",
"1.16.0"
]
]
},
{
"name": "charset-normalizer",
"specs": [
[
"==",
"3.3.2"
]
]
},
{
"name": "click",
"specs": [
[
"==",
"8.1.7"
]
]
},
{
"name": "cryptography",
"specs": [
[
"==",
"42.0.7"
]
]
},
{
"name": "Flask",
"specs": [
[
"==",
"3.0.3"
]
]
},
{
"name": "idna",
"specs": [
[
"==",
"3.7"
]
]
},
{
"name": "iniconfig",
"specs": [
[
"==",
"2.0.0"
]
]
},
{
"name": "isodate",
"specs": [
[
"==",
"0.6.1"
]
]
},
{
"name": "itsdangerous",
"specs": [
[
"==",
"2.2.0"
]
]
},
{
"name": "Jinja2",
"specs": [
[
"==",
"3.1.3"
]
]
},
{
"name": "jwcrypto",
"specs": [
[
"==",
"1.5.6"
]
]
},
{
"name": "lxml",
"specs": [
[
"==",
"5.2.1"
]
]
},
{
"name": "MarkupSafe",
"specs": [
[
"==",
"2.1.5"
]
]
},
{
"name": "numpy",
"specs": [
[
"==",
"1.26.4"
]
]
},
{
"name": "packaging",
"specs": [
[
"==",
"24.0"
]
]
},
{
"name": "pillow",
"specs": [
[
"==",
"10.3.0"
]
]
},
{
"name": "platformdirs",
"specs": [
[
"==",
"4.2.0"
]
]
},
{
"name": "pluggy",
"specs": [
[
"==",
"1.5.0"
]
]
},
{
"name": "psutil",
"specs": [
[
"==",
"5.9.8"
]
]
},
{
"name": "pycparser",
"specs": [
[
"==",
"2.22"
]
]
},
{
"name": "pytest",
"specs": [
[
"==",
"8.2.0"
]
]
},
{
"name": "pytz",
"specs": [
[
"==",
"2024.1"
]
]
},
{
"name": "redis",
"specs": [
[
"==",
"5.0.4"
]
]
},
{
"name": "requests",
"specs": [
[
"==",
"2.31.0"
]
]
},
{
"name": "requests-file",
"specs": [
[
"==",
"2.0.0"
]
]
},
{
"name": "requests-toolbelt",
"specs": [
[
"==",
"1.0.0"
]
]
},
{
"name": "simplejson",
"specs": [
[
"==",
"3.19.2"
]
]
},
{
"name": "six",
"specs": [
[
"==",
"1.16.0"
]
]
},
{
"name": "typing_extensions",
"specs": [
[
"==",
"4.11.0"
]
]
},
{
"name": "urllib3",
"specs": [
[
"==",
"2.2.1"
]
]
},
{
"name": "websockify",
"specs": [
[
"==",
"0.11.0"
]
]
},
{
"name": "Werkzeug",
"specs": [
[
"==",
"3.0.2"
]
]
},
{
"name": "zeep",
"specs": [
[
"==",
"4.2.1"
]
]
}
],
"lcname": "vbox-api-soap"
}