Name | wah JSON |
Version |
0.1.41
JSON |
| download |
home_page | https://github.com/yupeeee/WAH |
Summary | a library so simple you will learn Within An Hour |
upload_time | 2024-05-03 07:04:43 |
maintainer | None |
docs_url | None |
author | Juyeop Kim |
requires_python | None |
license | MIT |
keywords |
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
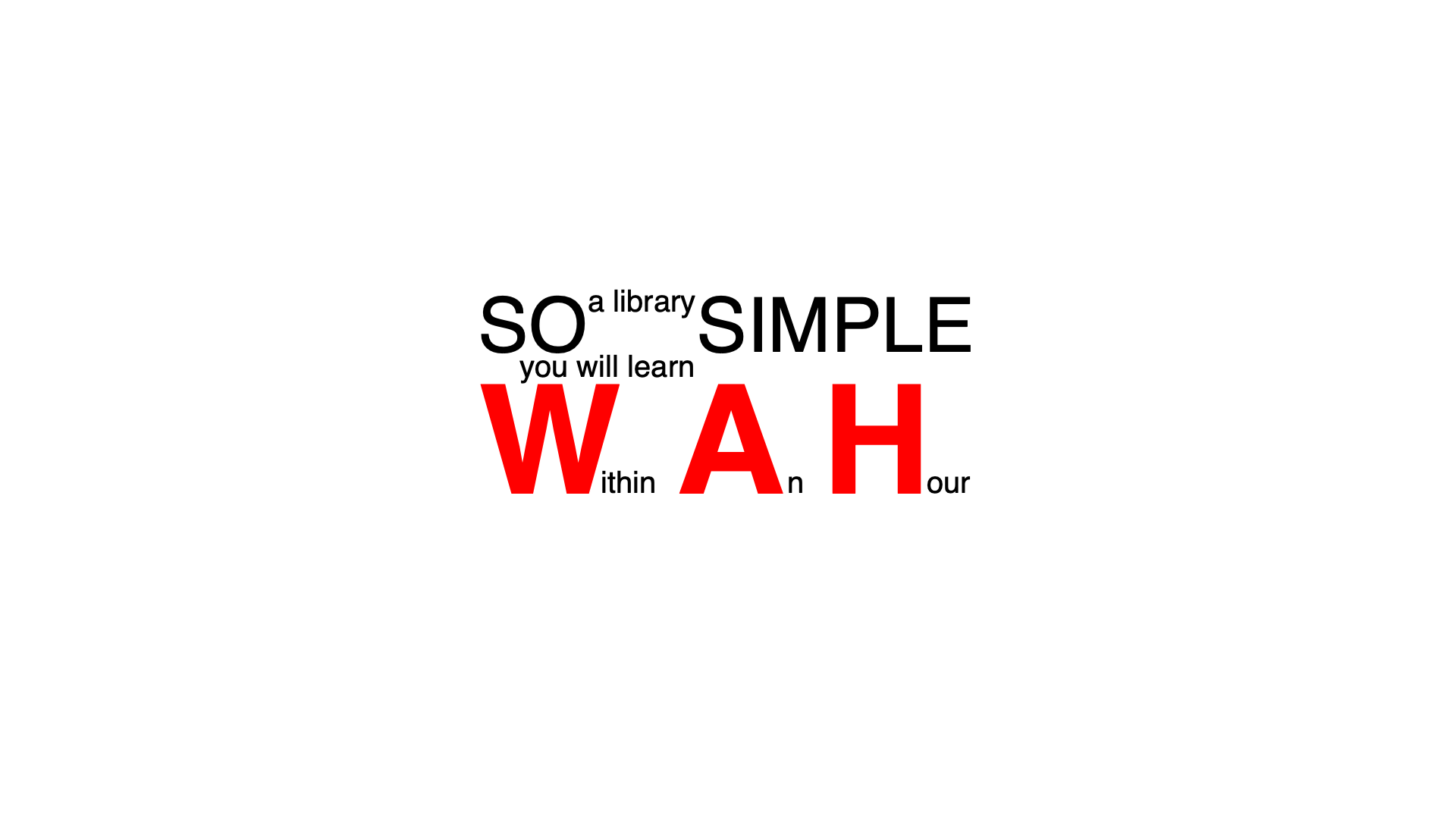
## Install
```commandline
pip install wah
```
### Requirements
You might want to manually install [**PyTorch**](https://pytorch.org/get-started/locally/)
for GPU computation.
```text
lightning
matplotlib
pandas
pyperclip
PyYAML
selenium
tensorboard
torch
torchaudio
torchmetrics
torchvision
webdriver_manager
```
## Model Training
Let's train [**ResNet50**](https://arxiv.org/abs/1512.03385) [1]
on [**CIFAR-10**](https://www.cs.toronto.edu/~kriz/cifar.html) [2] dataset
(Full example codes
[**here**](https://github.com/yupeeee/WAH/tree/main/examples/cifar10)).\
First, import the package.
```python
import wah
```
Second, write your own *config.yaml* file (which will do **everything** for you).
```yaml
--- # config.yaml
#
# Dataset
#
num_classes: 10
batch_size: 64
num_workers: 2
mixup_alpha: 0.
cutmix_alpha: 0.
# use_v2: False
#
# Training hyperparameters
#
epochs: 300
init_lr: 1.e-3
seed: 0
gpu: [ 0, ]
optimizer: AdamW
optimizer_cfg:
weight_decay: 5.e-2
lr_scheduler: CosineAnnealingLR
lr_scheduler_cfg:
eta_min: 1.e-5
warmup_lr_scheduler: LinearLR
warmup_lr_scheduler_cfg:
start_factor: 1.e-2
total_iters: 20
criterion: CrossEntropyLoss
criterion_cfg:
label_smoothing: 0.
#
# Metrics
#
metrics:
- "Acc1"
- "Acc5"
- "ECE"
- "sECE"
track:
- "grad_l2"
- "feature_rms"
... # end
```
- **num_classes** (*int*) -
number of classes in the dataset.
- **batch_size** (*int*) -
how many samples per batch to load.
- **num_workers** (*int*) -
how many subprocesses to use for data loading.
0 means that the data will be loaded in the main process.
- **mixup_alpha** (*float*) -
hyperparameter of the Beta distribution used for mixup.
([*mixup: Beyond Empirical Risk Minimization*](https://arxiv.org/abs/1710.09412))
- **cutmix_alpha** (*float*) -
hyperparameter of the Beta distribution used for mixup.
([*CutMix: Regularization Strategy to Train Strong Classifiers with Localizable Features*](https://arxiv.org/abs/1905.04899))
- **epochs** (*int*) -
stop training once this number of epochs is reached.
- **init_lr** (*float*) -
initial learning rate.
- **seed** (*int*) -
seed value for random number generation.
Must be a non-negative integer.
If a negative integer is provided, no seeding will occur.
- **gpu** (*List[int], optional*) -
the GPU device(s) to be utilized for computation.
If not specified, the system automatically detects and selects devices.
- **optimizer** (*str*) -
specifies which optimizer to use.
Must be one of the optimizers supported in
[*torch.optim*](https://pytorch.org/docs/stable/optim.html#algorithms).
- **optimizer_cfg** -
parameters for the specified optimizer.
The *params* and *lr* parameters do not need to be explicitly provided (automatically initialized).
- **lr_scheduler** (*str*) -
specifies which scheduler to use.
Must be one of the schedulers supported in
[*torch.optim.lr_scheduler*](https://pytorch.org/docs/stable/optim.html#how-to-adjust-learning-rate).
- **lr_scheduler_cfg** -
parameters for the specified scheduler.
The *optimizer* parameter does not need to be explicitly provided (automatically initialized).
- **warmup_lr_scheduler** (*str, optional*) -
specifies which scheduler to use for warmup phase.
Must be one of [*"ConstantLR"*, *"LinearLR"*, ].
- **warmup_lr_scheduler_cfg** (*optional*) -
parameters for the specified warmup scheduler.
The *optimizer* parameter does not need to be explicitly provided (automatically initialized).
Note that the *total_iters* parameter initializes the length of warmup phase.
- **criterion** (*str*) -
specifies which loss function to use.
Must be one of the loss functions supported in
[*torch.nn*](https://pytorch.org/docs/stable/nn.html#loss-functions).
- **criterion_cfg** (*optional*) -
parameters for the specified loss function.
- **label_smoothing** (*optional, float*) -
specifies the amount of smoothing when computing the loss, where 0.0 means no smoothing.
The targets become a mixture of the original ground truth and a uniform distribution
as described in [*Rethinking the Inception Architecture for Computer Vision*](https://arxiv.org/abs/1512.00567).
- **metrics** -
metrics to record during the training and validation stages.
Must be one of [*"Acc1"*, *"Acc5"*, *"ECE"*, *"sECE"*, ].
- **track** -
values to track during the training and validation stages.
Must be one of [*"grad_l2"*, *"feature_rms"*, ].
Third, load your *config.yaml* file.
```python
config = wah.load_config(PATH_TO_CONFIG)
```
Fourth, load your dataloaders.
```python
train_dataset = wah.CIFAR10(
root=...,
train=True,
transform="auto",
target_transform="auto",
download=True,
)
val_dataset = wah.CIFAR10(
root=...,
train=False,
transform="auto",
target_transform="auto",
download=True,
)
train_dataloader = wah.load_dataloader(
dataset=train_dataset,
config=config,
train=True,
)
val_dataloader = wah.load_dataloader(
dataset=val_dataset,
config=config,
train=False,
)
```
Fifth, load your model.
```python
from torchvision.models import resnet50
model = resnet50(weights=None, num_classes=10)
model = wah.Wrapper(model, config)
```
Finally, train your model!
```python
trainer = wah.load_trainer(
config=config,
save_dir=TRAIN_LOG_ROOT,
name="cifar10-resnet50",
every_n_epochs=SAVE_CKPT_PER_THIS_EPOCH,
)
trainer.fit(
model=model,
train_dataloaders=train_dataloader,
val_dataloaders=val_dataloader,
)
```
You can check your train logs by running the following command:
```commandline
tensorboard --logdir TRAIN_LOG_ROOT
```
### References
[1] Kaiming He, Xiangyu Zhang, Shaoqing Ren, and Jian Sun. Deep Residual Learning for Image Recognition. CVPR, 2016.\
[2] Alex Krizhevsky and Geoffrey Hinton. Learning Multiple Layers of Features from Tiny Images. Tech. Rep., University
of Toronto, Toronto, Ontario, 2009.
Raw data
{
"_id": null,
"home_page": "https://github.com/yupeeee/WAH",
"name": "wah",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": null,
"author": "Juyeop Kim",
"author_email": "juyeopkim@yonsei.ac.kr",
"download_url": "https://files.pythonhosted.org/packages/3d/f2/bafd526f9fa7f094cf854833ebaee045eb0930732f6c6e82061318a53e08/wah-0.1.41.tar.gz",
"platform": null,
"description": "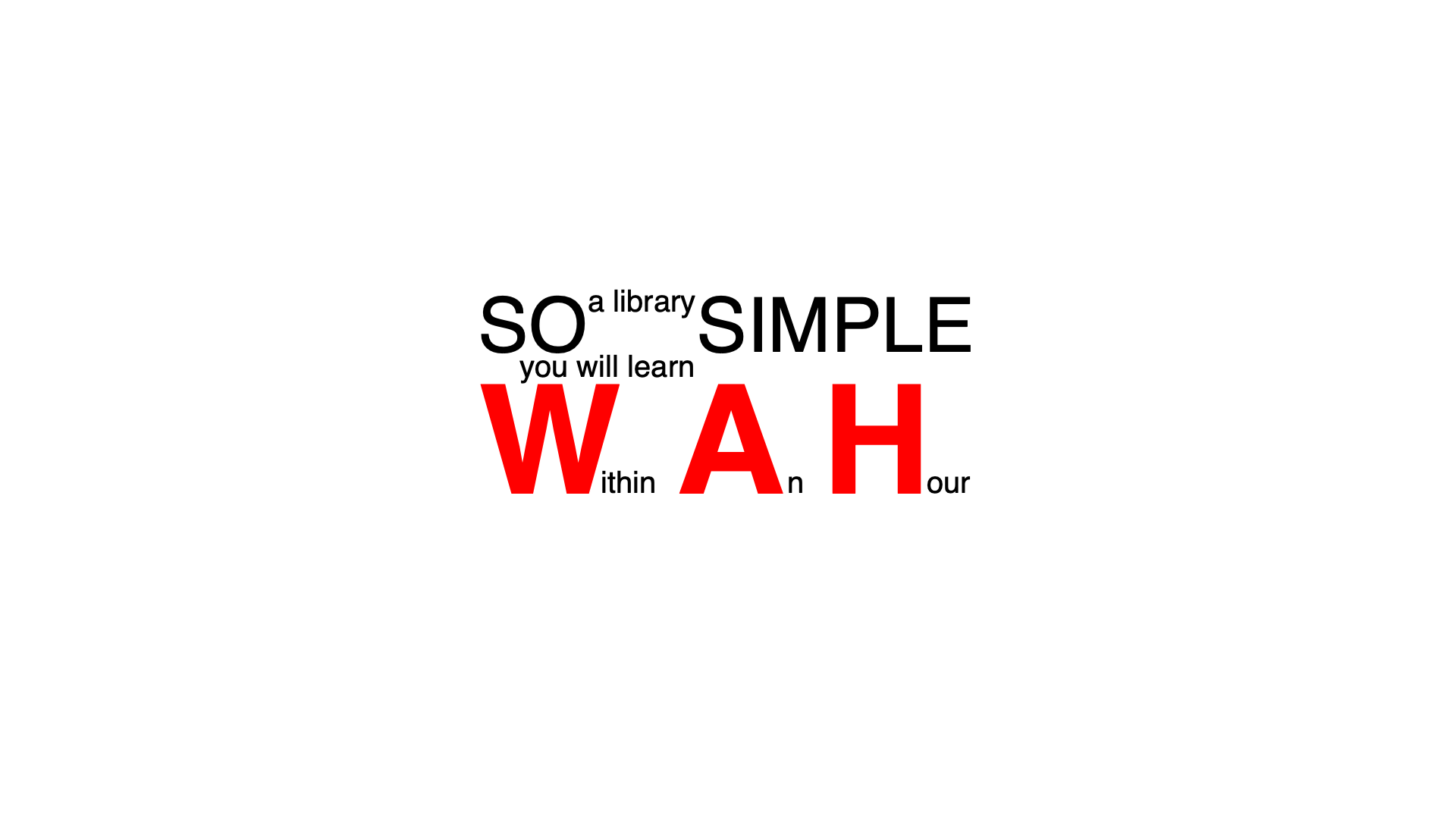\r\n\r\n## Install\r\n\r\n```commandline\r\npip install wah\r\n```\r\n\r\n### Requirements\r\n\r\nYou might want to manually install [**PyTorch**](https://pytorch.org/get-started/locally/)\r\nfor GPU computation.\r\n\r\n```text\r\nlightning\r\nmatplotlib\r\npandas\r\npyperclip\r\nPyYAML\r\nselenium\r\ntensorboard\r\ntorch\r\ntorchaudio\r\ntorchmetrics\r\ntorchvision\r\nwebdriver_manager\r\n```\r\n\r\n## Model Training\r\n\r\nLet's train [**ResNet50**](https://arxiv.org/abs/1512.03385) [1]\r\non [**CIFAR-10**](https://www.cs.toronto.edu/~kriz/cifar.html) [2] dataset\r\n(Full example codes\r\n[**here**](https://github.com/yupeeee/WAH/tree/main/examples/cifar10)).\\\r\nFirst, import the package.\r\n\r\n```python\r\nimport wah\r\n```\r\n\r\nSecond, write your own *config.yaml* file (which will do **everything** for you).\r\n\r\n```yaml\r\n--- # config.yaml\r\n\r\n\r\n#\r\n# Dataset\r\n#\r\nnum_classes: 10\r\nbatch_size: 64\r\nnum_workers: 2\r\nmixup_alpha: 0.\r\ncutmix_alpha: 0.\r\n# use_v2: False\r\n\r\n\r\n#\r\n# Training hyperparameters\r\n#\r\nepochs: 300\r\ninit_lr: 1.e-3\r\nseed: 0\r\ngpu: [ 0, ]\r\n\r\noptimizer: AdamW\r\noptimizer_cfg:\r\n weight_decay: 5.e-2\r\n\r\nlr_scheduler: CosineAnnealingLR\r\nlr_scheduler_cfg:\r\n eta_min: 1.e-5\r\n\r\nwarmup_lr_scheduler: LinearLR\r\nwarmup_lr_scheduler_cfg:\r\n start_factor: 1.e-2\r\n total_iters: 20\r\n\r\ncriterion: CrossEntropyLoss\r\ncriterion_cfg:\r\n label_smoothing: 0.\r\n\r\n\r\n#\r\n# Metrics\r\n#\r\nmetrics:\r\n- \"Acc1\"\r\n- \"Acc5\"\r\n- \"ECE\"\r\n- \"sECE\"\r\n\r\ntrack:\r\n- \"grad_l2\"\r\n- \"feature_rms\"\r\n\r\n\r\n... # end\r\n```\r\n\r\n- **num_classes** (*int*) -\r\n number of classes in the dataset.\r\n\r\n- **batch_size** (*int*) -\r\n how many samples per batch to load.\r\n\r\n- **num_workers** (*int*) -\r\n how many subprocesses to use for data loading.\r\n 0 means that the data will be loaded in the main process.\r\n\r\n- **mixup_alpha** (*float*) -\r\n hyperparameter of the Beta distribution used for mixup.\r\n ([*mixup: Beyond Empirical Risk Minimization*](https://arxiv.org/abs/1710.09412))\r\n\r\n- **cutmix_alpha** (*float*) -\r\n hyperparameter of the Beta distribution used for mixup.\r\n ([*CutMix: Regularization Strategy to Train Strong Classifiers with Localizable Features*](https://arxiv.org/abs/1905.04899))\r\n\r\n- **epochs** (*int*) -\r\n stop training once this number of epochs is reached.\r\n\r\n- **init_lr** (*float*) -\r\n initial learning rate.\r\n\r\n- **seed** (*int*) -\r\n seed value for random number generation.\r\n Must be a non-negative integer.\r\n If a negative integer is provided, no seeding will occur.\r\n\r\n- **gpu** (*List[int], optional*) -\r\n the GPU device(s) to be utilized for computation.\r\n If not specified, the system automatically detects and selects devices.\r\n\r\n- **optimizer** (*str*) -\r\n specifies which optimizer to use.\r\n Must be one of the optimizers supported in\r\n [*torch.optim*](https://pytorch.org/docs/stable/optim.html#algorithms).\r\n\r\n- **optimizer_cfg** -\r\n parameters for the specified optimizer.\r\n The *params* and *lr* parameters do not need to be explicitly provided (automatically initialized).\r\n\r\n- **lr_scheduler** (*str*) -\r\n specifies which scheduler to use.\r\n Must be one of the schedulers supported in\r\n [*torch.optim.lr_scheduler*](https://pytorch.org/docs/stable/optim.html#how-to-adjust-learning-rate).\r\n\r\n- **lr_scheduler_cfg** -\r\n parameters for the specified scheduler.\r\n The *optimizer* parameter does not need to be explicitly provided (automatically initialized).\r\n\r\n- **warmup_lr_scheduler** (*str, optional*) -\r\n specifies which scheduler to use for warmup phase.\r\n Must be one of [*\"ConstantLR\"*, *\"LinearLR\"*, ].\r\n\r\n- **warmup_lr_scheduler_cfg** (*optional*) -\r\n parameters for the specified warmup scheduler.\r\n The *optimizer* parameter does not need to be explicitly provided (automatically initialized).\r\n Note that the *total_iters* parameter initializes the length of warmup phase.\r\n\r\n- **criterion** (*str*) -\r\n specifies which loss function to use.\r\n Must be one of the loss functions supported in\r\n [*torch.nn*](https://pytorch.org/docs/stable/nn.html#loss-functions).\r\n\r\n- **criterion_cfg** (*optional*) -\r\n parameters for the specified loss function.\r\n\r\n - **label_smoothing** (*optional, float*) -\r\n specifies the amount of smoothing when computing the loss, where 0.0 means no smoothing.\r\n The targets become a mixture of the original ground truth and a uniform distribution\r\n as described in [*Rethinking the Inception Architecture for Computer Vision*](https://arxiv.org/abs/1512.00567).\r\n\r\n- **metrics** -\r\n metrics to record during the training and validation stages.\r\n Must be one of [*\"Acc1\"*, *\"Acc5\"*, *\"ECE\"*, *\"sECE\"*, ].\r\n\r\n- **track** -\r\n values to track during the training and validation stages.\r\n Must be one of [*\"grad_l2\"*, *\"feature_rms\"*, ].\r\n\r\nThird, load your *config.yaml* file.\r\n\r\n```python\r\nconfig = wah.load_config(PATH_TO_CONFIG)\r\n```\r\n\r\nFourth, load your dataloaders.\r\n\r\n```python\r\ntrain_dataset = wah.CIFAR10(\r\n root=...,\r\n train=True,\r\n transform=\"auto\",\r\n target_transform=\"auto\",\r\n download=True,\r\n)\r\nval_dataset = wah.CIFAR10(\r\n root=...,\r\n train=False,\r\n transform=\"auto\",\r\n target_transform=\"auto\",\r\n download=True,\r\n)\r\n\r\ntrain_dataloader = wah.load_dataloader(\r\n dataset=train_dataset,\r\n config=config,\r\n train=True,\r\n)\r\nval_dataloader = wah.load_dataloader(\r\n dataset=val_dataset,\r\n config=config,\r\n train=False,\r\n)\r\n```\r\n\r\nFifth, load your model.\r\n\r\n```python\r\nfrom torchvision.models import resnet50\r\n\r\nmodel = resnet50(weights=None, num_classes=10)\r\nmodel = wah.Wrapper(model, config)\r\n```\r\n\r\nFinally, train your model!\r\n\r\n```python\r\ntrainer = wah.load_trainer(\r\n config=config,\r\n save_dir=TRAIN_LOG_ROOT,\r\n name=\"cifar10-resnet50\",\r\n every_n_epochs=SAVE_CKPT_PER_THIS_EPOCH,\r\n)\r\ntrainer.fit(\r\n model=model,\r\n train_dataloaders=train_dataloader,\r\n val_dataloaders=val_dataloader,\r\n)\r\n```\r\n\r\nYou can check your train logs by running the following command:\r\n\r\n```commandline\r\ntensorboard --logdir TRAIN_LOG_ROOT\r\n```\r\n\r\n### References\r\n\r\n[1] Kaiming He, Xiangyu Zhang, Shaoqing Ren, and Jian Sun. Deep Residual Learning for Image Recognition. CVPR, 2016.\\\r\n[2] Alex Krizhevsky and Geoffrey Hinton. Learning Multiple Layers of Features from Tiny Images. Tech. Rep., University\r\nof Toronto, Toronto, Ontario, 2009.\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "a library so simple you will learn Within An Hour",
"version": "0.1.41",
"project_urls": {
"Homepage": "https://github.com/yupeeee/WAH"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "3df2bafd526f9fa7f094cf854833ebaee045eb0930732f6c6e82061318a53e08",
"md5": "611ad31318eb3a2d23b28c02f555073e",
"sha256": "1535ec26de9a23ab85389e23b52bf8390dab4ffcb88baaef6a0e9f7cf24281eb"
},
"downloads": -1,
"filename": "wah-0.1.41.tar.gz",
"has_sig": false,
"md5_digest": "611ad31318eb3a2d23b28c02f555073e",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 32352,
"upload_time": "2024-05-03T07:04:43",
"upload_time_iso_8601": "2024-05-03T07:04:43.546451Z",
"url": "https://files.pythonhosted.org/packages/3d/f2/bafd526f9fa7f094cf854833ebaee045eb0930732f6c6e82061318a53e08/wah-0.1.41.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-05-03 07:04:43",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "yupeeee",
"github_project": "WAH",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "wah"
}