# Aiomql - Bot Building Framework and Asynchronous MetaTrader5 Library



### Installation
```bash
pip install aiomql
```
### Key Features
- Asynchronous Python Library For MetaTrader5
- Asynchronous Bot Building Framework
- Build bots for trading in different financial markets.
- Use threadpool executors to run multiple strategies on multiple instruments concurrently
- Records and keep track of trades and strategies in csv files.
- Helper classes for Bot Building. Easy to use and extend.
- Compatible with pandas-ta.
- Sample Pre-Built strategies
- Specify and Manage Trading Sessions
- Risk Management
- Backtesting Engine
- Run multiple bots concurrently with different accounts from the same broker or different brokers
- Easy to use and very accurate backtesting engine
### As an asynchronous MetaTrader5 Libray
```python
import asyncio
from aiomql import MetaTrader
async def main():
mt5 = MetaTrader()
res = await mt5.initialize(login=31288540, password='nwa0#anaEze', server='Deriv-Demo')
if not res:
print('Unable to login and initialize')
return
# get account information
acc = await mt5.account_info()
print(acc)
# get symbols
symbols = await mt5.symbols_get()
print(symbols)
asyncio.run(main())
```
### As a Bot Building FrameWork using a Sample Strategy
Aiomql allows you to focus on building trading strategies and not worry about the underlying infrastructure.
It provides a simple and easy to use framework for building bots with rich features and functionalities.
```python
from datetime import time
import logging
from aiomql import Bot, ForexSymbol, FingerTrap, Session, Sessions, RAM, SimpleTrader, TimeFrame, Chaos
logging.basicConfig(level=logging.INFO)
def build_bot():
bot = Bot()
# configure the parameters and the trader for a strategy
params = {'fast_period': 8, 'slow_period': 34, 'etf': TimeFrame.M5}
symbols = ['GBPUSD', 'AUDUSD', 'USDCAD', 'EURGBP', 'EURUSD']
symbols = [ForexSymbol(name=sym) for sym in symbols]
strategies = [FingerTrap(symbol=sym, params=params)for sym in symbols]
bot.add_strategies(strategies)
# create a strategy that uses sessions
# sessions are used to specify the trading hours for a particular market
# the strategy will only trade during the specified sessions
london = Session(name='London', start=time(8, 0), end=time(16, 0))
new_york = Session(name='New York', start=time(13, 0), end=time(21, 0))
tokyo = Session(name='Tokyo', start=time(0, 0), end=time(8, 0))
sessions = Sessions(sessions=[london, new_york, tokyo])
jpy_strategy = Chaos(symbol=ForexSymbol(name='USDJPY'), sessions=sessions)
bot.add_strategy(strategy=jpy_strategy)
bot.execute()
# run the bot
build_bot()
```
### Backtesting
Aiomql provides a very accurate backtesting engine that allows you to test your trading strategies before deploying
them in the market. The backtest engine prioritizes accuracy over speed, but allows you to increase the speed
as desired. It is very easy to use and provides a lot of flexibility. The backtester is designed to run strategies
seamlessly without need for modification of the strategy code. When running in backtest mode all the classes that
needs to know if they are running in backtest mode will be able to do so and adjust their behavior accordingly.
```python
from aiomql import MetaBackTester, BackTestEngine, MetaTrader
import logging
from datetime import datetime, UTC
from aiomql.lib.backtester import BackTester
from aiomql.core import Config
from aiomql.contrib.strategies import FingerTrap
from aiomql.contrib.symbols import ForexSymbol
from aiomql.core.backtesting import BackTestEngine
def back_tester():
config = Config(mode="backtest")
logging.basicConfig(level=logging.INFO, format="%(asctime)s - %(name)s - %(levelname)s - %(message)s")
syms = ["Volatility 75 Index", "Volatility 100 Index", "Volatility 25 Index", "Volatility 10 Index"]
symbols = [ForexSymbol(name=sym) for sym in syms]
strategies = [FingerTrap(symbol=symbol) for symbol in symbols]
# create start time and end time for the backtest
start = datetime(2024, 5, 1, tzinfo=UTC)
stop_time = datetime(2024, 5, 2, tzinfo=UTC)
end = datetime(2024, 5, 7, tzinfo=UTC)
# create a backtest engine
back_test_engine = BackTestEngine(start=start, end=end, speed=3600, stop_time=stop_time,
close_open_positions_on_exit=True, assign_to_config=True, preload=True,
account_info={"balance": 350})
# add it to the backtester
backtester = BackTester(backtest_engine=back_test_engine)
# add strategies to the backtester
backtester.add_strategies(strategies=strategies)
backtester.execute()
back_tester()
```
### Writing a Custom Strategy
Aiomql provides a simple and easy to use framework for building trading strategies. You can easily extend the
framework to build your own custom strategies. Below is an example of a simple strategy that buys when the fast
moving average crosses above the slow moving average and sells when the fast moving average crosses below the slow
moving average.
```python
# emaxover.py
from aiomql import Strategy, ForexSymbol, TimeFrame, Tracker, OrderType, Sessions, Trader, ScalpTrader
class EMAXOver(Strategy):
ttf: TimeFrame # time frame for the strategy
tcc: int # how many candles to consider
fast_ema: int # fast moving average period
slow_ema: int # slow moving average period
tracker: Tracker # tracker to keep track of strategy state
interval: TimeFrame # intervals to check for entry and exit signals
timeout: int # timeout after placing an order in seconds
# default parameters for the strategy
# they are set as attributes. You can override them in the constructor via the params argument.
parameters = {'ttf': TimeFrame.H1, 'tcc': 3000, 'fast_ema': 34, 'slow_ema': 55, 'interval': TimeFrame.M15,
'timeout': 3 * 60 * 60}
def __init__(self, *, symbol: ForexSymbol, params: dict | None = None, trader: Trader = None,
sessions: Sessions = None, name: str = "EMAXOver"):
super().__init__(symbol=symbol, params=params, sessions=sessions, name=name)
self.tracker = Tracker(snooze=self.interval.seconds)
self.trader = trader or ScalpTrader(symbol=self.symbol)
async def find_entry(self):
# get the candles
candles = await self.symbol.copy_rates_from_pos(timeframe=self.ttf, start_position=0, count=self.tcc)
# get the fast moving average
candles.ta.ema(length=self.fast_ema, append=True)
# get the slow moving average
candles.ta.ema(length=self.slow_ema, append=True)
# rename the columns
candles.rename(**{f"EMA_{self.fast_ema}": "fast_ema", f"EMA_{self.slow_ema}": "slow_ema"}, inplace=True)
# check for crossovers
# fast above slow
fas = candles.ta_lib.cross(candles.fast_ema, candles.slow_ema, above=True)
# fast below slow
fbs = candles.ta_lib.cross(candles.fast_ema, candles.slow_ema, above=False)
## check for entry signals in the current candle
if fas.iloc[-1]:
self.tracker.update(order_type=OrderType.BUY, snooze=self.timeout)
elif fbs.iloc[-1]:
self.tracker.update(order_type=OrderType.SELL, snooze=self.timeout)
else:
self.tracker.update(order_type=None, snooze=self.interval.seconds)
async def trade(self):
await self.find_entry()
if self.tracker.order_type is None:
await self.sleep(secs=self.tracker.snooze)
else:
await self.trader.place_trade(order_type=self.tracker.order_type, parameters=self.parameters)
await self.delay(secs=self.tracker.snooze)
```
### Testing
Run the tests with pytest
```bash
pytest test
```
### API Documentation
see [API Documentation](docs) for more details
### Contributing
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
### Support
Feeling generous, like the package or want to see it become a more mature package?
Consider supporting the project by buying me a coffee.
[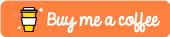](https://www.buymeacoffee.com/ichingasamuel)
Raw data
{
"_id": null,
"home_page": null,
"name": "aiomql",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.11",
"maintainer_email": null,
"keywords": "MetaTrader5, Asynchronous, Algorithmic Trading, Trading Bot, Backtesting, Technical Analysis, Forex, Stocks, Cryptocurrency, Futures, Options",
"author": null,
"author_email": "Ichinga Samuel <ichingasamuel@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/5d/5d/77959bcf191e64238c6b87fc8accec9a979a1aae3f68b81bb4207f0cc60f/aiomql-4.0.0.tar.gz",
"platform": null,
"description": "# Aiomql - Bot Building Framework and Asynchronous MetaTrader5 Library\r\n\r\n\r\n\r\n\r\n\r\n### Installation\r\n```bash\r\npip install aiomql\r\n```\r\n\r\n### Key Features\r\n- Asynchronous Python Library For MetaTrader5\r\n- Asynchronous Bot Building Framework\r\n- Build bots for trading in different financial markets.\r\n- Use threadpool executors to run multiple strategies on multiple instruments concurrently\r\n- Records and keep track of trades and strategies in csv files.\r\n- Helper classes for Bot Building. Easy to use and extend.\r\n- Compatible with pandas-ta.\r\n- Sample Pre-Built strategies\r\n- Specify and Manage Trading Sessions\r\n- Risk Management\r\n- Backtesting Engine\r\n- Run multiple bots concurrently with different accounts from the same broker or different brokers\r\n- Easy to use and very accurate backtesting engine\r\n\r\n### As an asynchronous MetaTrader5 Libray\r\n```python\r\nimport asyncio\r\n\r\nfrom aiomql import MetaTrader\r\n\r\n\r\nasync def main():\r\n mt5 = MetaTrader()\r\n res = await mt5.initialize(login=31288540, password='nwa0#anaEze', server='Deriv-Demo')\r\n if not res:\r\n print('Unable to login and initialize')\r\n return \r\n # get account information\r\n acc = await mt5.account_info()\r\n print(acc)\r\n # get symbols\r\n symbols = await mt5.symbols_get()\r\n print(symbols)\r\n \r\nasyncio.run(main())\r\n```\r\n\r\n### As a Bot Building FrameWork using a Sample Strategy\r\nAiomql allows you to focus on building trading strategies and not worry about the underlying infrastructure.\r\nIt provides a simple and easy to use framework for building bots with rich features and functionalities.\r\n\r\n\r\n```python\r\nfrom datetime import time\r\nimport logging\r\n\r\nfrom aiomql import Bot, ForexSymbol, FingerTrap, Session, Sessions, RAM, SimpleTrader, TimeFrame, Chaos\r\n\r\nlogging.basicConfig(level=logging.INFO)\r\n\r\n\r\ndef build_bot():\r\n bot = Bot()\r\n # configure the parameters and the trader for a strategy\r\n params = {'fast_period': 8, 'slow_period': 34, 'etf': TimeFrame.M5}\r\n symbols = ['GBPUSD', 'AUDUSD', 'USDCAD', 'EURGBP', 'EURUSD']\r\n symbols = [ForexSymbol(name=sym) for sym in symbols]\r\n strategies = [FingerTrap(symbol=sym, params=params)for sym in symbols]\r\n bot.add_strategies(strategies)\r\n \r\n # create a strategy that uses sessions\r\n # sessions are used to specify the trading hours for a particular market\r\n # the strategy will only trade during the specified sessions\r\n london = Session(name='London', start=time(8, 0), end=time(16, 0))\r\n new_york = Session(name='New York', start=time(13, 0), end=time(21, 0))\r\n tokyo = Session(name='Tokyo', start=time(0, 0), end=time(8, 0))\r\n \r\n sessions = Sessions(sessions=[london, new_york, tokyo]) \r\n jpy_strategy = Chaos(symbol=ForexSymbol(name='USDJPY'), sessions=sessions)\r\n bot.add_strategy(strategy=jpy_strategy)\r\n bot.execute()\r\n\r\n# run the bot\r\nbuild_bot()\r\n```\r\n\r\n### Backtesting\r\nAiomql provides a very accurate backtesting engine that allows you to test your trading strategies before deploying\r\nthem in the market. The backtest engine prioritizes accuracy over speed, but allows you to increase the speed\r\nas desired. It is very easy to use and provides a lot of flexibility. The backtester is designed to run strategies\r\nseamlessly without need for modification of the strategy code. When running in backtest mode all the classes that\r\nneeds to know if they are running in backtest mode will be able to do so and adjust their behavior accordingly.\r\n\r\n```python\r\nfrom aiomql import MetaBackTester, BackTestEngine, MetaTrader\r\nimport logging\r\nfrom datetime import datetime, UTC\r\n\r\nfrom aiomql.lib.backtester import BackTester\r\nfrom aiomql.core import Config\r\nfrom aiomql.contrib.strategies import FingerTrap\r\nfrom aiomql.contrib.symbols import ForexSymbol\r\nfrom aiomql.core.backtesting import BackTestEngine\r\n\r\n\r\ndef back_tester():\r\n config = Config(mode=\"backtest\")\r\n logging.basicConfig(level=logging.INFO, format=\"%(asctime)s - %(name)s - %(levelname)s - %(message)s\")\r\n syms = [\"Volatility 75 Index\", \"Volatility 100 Index\", \"Volatility 25 Index\", \"Volatility 10 Index\"]\r\n symbols = [ForexSymbol(name=sym) for sym in syms]\r\n strategies = [FingerTrap(symbol=symbol) for symbol in symbols]\r\n \r\n # create start time and end time for the backtest\r\n start = datetime(2024, 5, 1, tzinfo=UTC)\r\n stop_time = datetime(2024, 5, 2, tzinfo=UTC)\r\n end = datetime(2024, 5, 7, tzinfo=UTC)\r\n \r\n # create a backtest engine\r\n back_test_engine = BackTestEngine(start=start, end=end, speed=3600, stop_time=stop_time,\r\n close_open_positions_on_exit=True, assign_to_config=True, preload=True,\r\n account_info={\"balance\": 350})\r\n # add it to the backtester\r\n backtester = BackTester(backtest_engine=back_test_engine)\r\n # add strategies to the backtester\r\n backtester.add_strategies(strategies=strategies)\r\n backtester.execute()\r\n\r\n\r\nback_tester()\r\n```\r\n\r\n### Writing a Custom Strategy\r\nAiomql provides a simple and easy to use framework for building trading strategies. You can easily extend the\r\nframework to build your own custom strategies. Below is an example of a simple strategy that buys when the fast\r\nmoving average crosses above the slow moving average and sells when the fast moving average crosses below the slow\r\nmoving average.\r\n\r\n```python\r\n# emaxover.py\r\nfrom aiomql import Strategy, ForexSymbol, TimeFrame, Tracker, OrderType, Sessions, Trader, ScalpTrader\r\n\r\n\r\nclass EMAXOver(Strategy):\r\n ttf: TimeFrame # time frame for the strategy\r\n tcc: int # how many candles to consider\r\n fast_ema: int # fast moving average period\r\n slow_ema: int # slow moving average period\r\n tracker: Tracker # tracker to keep track of strategy state\r\n interval: TimeFrame # intervals to check for entry and exit signals\r\n timeout: int # timeout after placing an order in seconds\r\n\r\n # default parameters for the strategy\r\n # they are set as attributes. You can override them in the constructor via the params argument.\r\n parameters = {'ttf': TimeFrame.H1, 'tcc': 3000, 'fast_ema': 34, 'slow_ema': 55, 'interval': TimeFrame.M15,\r\n 'timeout': 3 * 60 * 60}\r\n\r\n def __init__(self, *, symbol: ForexSymbol, params: dict | None = None, trader: Trader = None,\r\n sessions: Sessions = None, name: str = \"EMAXOver\"):\r\n super().__init__(symbol=symbol, params=params, sessions=sessions, name=name)\r\n self.tracker = Tracker(snooze=self.interval.seconds)\r\n self.trader = trader or ScalpTrader(symbol=self.symbol)\r\n\r\n async def find_entry(self):\r\n # get the candles\r\n candles = await self.symbol.copy_rates_from_pos(timeframe=self.ttf, start_position=0, count=self.tcc)\r\n\r\n # get the fast moving average\r\n candles.ta.ema(length=self.fast_ema, append=True)\r\n # get the slow moving average\r\n candles.ta.ema(length=self.slow_ema, append=True)\r\n # rename the columns\r\n candles.rename(**{f\"EMA_{self.fast_ema}\": \"fast_ema\", f\"EMA_{self.slow_ema}\": \"slow_ema\"}, inplace=True)\r\n\r\n # check for crossovers\r\n # fast above slow\r\n fas = candles.ta_lib.cross(candles.fast_ema, candles.slow_ema, above=True)\r\n # fast below slow\r\n fbs = candles.ta_lib.cross(candles.fast_ema, candles.slow_ema, above=False)\r\n\r\n ## check for entry signals in the current candle\r\n if fas.iloc[-1]:\r\n self.tracker.update(order_type=OrderType.BUY, snooze=self.timeout)\r\n elif fbs.iloc[-1]:\r\n self.tracker.update(order_type=OrderType.SELL, snooze=self.timeout)\r\n else:\r\n self.tracker.update(order_type=None, snooze=self.interval.seconds)\r\n\r\n async def trade(self):\r\n await self.find_entry()\r\n if self.tracker.order_type is None:\r\n await self.sleep(secs=self.tracker.snooze)\r\n else:\r\n await self.trader.place_trade(order_type=self.tracker.order_type, parameters=self.parameters)\r\n await self.delay(secs=self.tracker.snooze)\r\n\r\n```\r\n\r\n### Testing\r\n\r\nRun the tests with pytest\r\n\r\n```bash\r\npytest test \r\n```\r\n\r\n### API Documentation\r\nsee [API Documentation](docs) for more details\r\n\r\n### Contributing\r\nPull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.\r\n\r\n### Support\r\nFeeling generous, like the package or want to see it become a more mature package?\r\n\r\nConsider supporting the project by buying me a coffee.\r\n\r\n\r\n[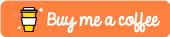](https://www.buymeacoffee.com/ichingasamuel)\r\n",
"bugtrack_url": null,
"license": null,
"summary": "Asynchronous MetaTrader5 library and Algorithmic Trading Framework",
"version": "4.0.0",
"project_urls": {
"Bug Tracker": "https://github.com/Ichinga-Samuel/aiomql/issues",
"Homepage": "https://github.com/Ichinga-Samuel/aiomql"
},
"split_keywords": [
"metatrader5",
" asynchronous",
" algorithmic trading",
" trading bot",
" backtesting",
" technical analysis",
" forex",
" stocks",
" cryptocurrency",
" futures",
" options"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "0b341aeb5572b0d39f827be078b1f0967a304213c4018f89607f2682aac0eb52",
"md5": "d17319c21bf73a1242aebe8030f104a2",
"sha256": "9536cb28eb5a822aa9bdd26164095da52ec0fb901dcfc005fa560d05645762db"
},
"downloads": -1,
"filename": "aiomql-4.0.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "d17319c21bf73a1242aebe8030f104a2",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.11",
"size": 96017,
"upload_time": "2024-11-16T11:39:08",
"upload_time_iso_8601": "2024-11-16T11:39:08.220035Z",
"url": "https://files.pythonhosted.org/packages/0b/34/1aeb5572b0d39f827be078b1f0967a304213c4018f89607f2682aac0eb52/aiomql-4.0.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5d5d77959bcf191e64238c6b87fc8accec9a979a1aae3f68b81bb4207f0cc60f",
"md5": "a0547d2d69f0b117aec1ef8613344c6f",
"sha256": "be7f32ae46429632e0e3d2520f70386852057a3c004b35e7c8dc650f0bbc2b85"
},
"downloads": -1,
"filename": "aiomql-4.0.0.tar.gz",
"has_sig": false,
"md5_digest": "a0547d2d69f0b117aec1ef8613344c6f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.11",
"size": 81286,
"upload_time": "2024-11-16T11:39:10",
"upload_time_iso_8601": "2024-11-16T11:39:10.555246Z",
"url": "https://files.pythonhosted.org/packages/5d/5d/77959bcf191e64238c6b87fc8accec9a979a1aae3f68b81bb4207f0cc60f/aiomql-4.0.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-11-16 11:39:10",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Ichinga-Samuel",
"github_project": "aiomql",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "aiomql"
}